Welcome, fellow travelers, to the realm of Java mastery – a realm where the possibilities are as boundless as the horizons of the digital universe. In this journey, we shall embark upon an odyssey of learning, traversing the vast expanse of Java’s syntax, semantics, and intricacies. So strap yourselves in, for the adventure of a lifetime awaits!
Setting Sail: Beginning the Java Odyssey
Picture yourself standing on the shores of a vast and uncharted sea, gazing out at the shimmering waters that stretch as far as the eye can see. This is your gateway to the world of Java – a world brimming with potential and ripe for exploration. As you step onto the deck of your metaphorical ship, you feel a sense of excitement coursing through your veins. The wind whispers tales of adventure, and the stars above beckon you onward.
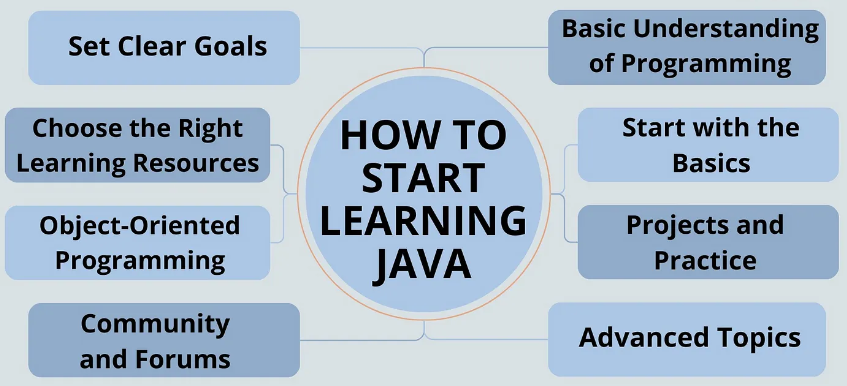
The path to becoming a Java developer calls for commitment, perseverance, and time. Java is a widely used programming language that may be used to create a wide range of applications, from straightforward desktop apps to intricate business systems. In the employment market, there is a significant need for Java developers, and this demand is only projected to increase.
Understanding the Java Ecosystem
The essential elements of JDK, JRE, and JVM—each with a distinct impact on molding the Java programming experience—are at the center of this journey.
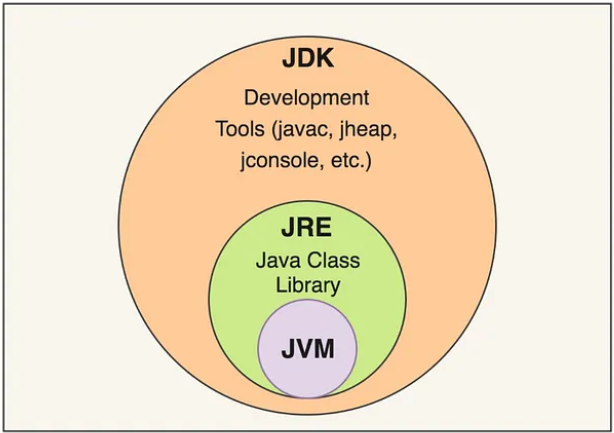
- JDK (Java Development Kit): The Java Development Kit (JDK) is a powerful tool for developers, facilitating unit testing, code quality, and maintenance. Its JUnit framework enables easy test execution. The JDK’s extensive toolset includes JavaFX and GUI libraries, allowing developers to create interactive applications. It also supports containerization and packaging, allowing developers to create custom runtime images for their applications, reducing the overall Java application footprint.
- JRE (Java Runtime Environment): The Java Runtime Environment (JRE) simplifies network communication in Java applications by including the java.net package. This package simplifies networking tasks and allows developers to interact with external services. The JRE also supports runtime support, including Java Naming and Directory Interface (JNDI) and Java Management Extensions (JMX). It also supports internationalization and localization, allowing developers to create applications that cater to diverse linguistic and cultural preferences.
- JVM (Java Virtual Machine): The Java Virtual Machine (JVM) is a virtualized execution system that allows for “Write Once, Run Anywhere” capabilities. It offers advanced features like Garbage Collection tuning options, Just-In-Time compilation, Metaspace and Class Data Sharing, and Ahead-of-Time (AOT) compilation. These options allow developers to optimize memory usage, optimize code based on the running application and hardware, and reduce startup times and memory overhead. The JVM adapts to the evolving nature of applications, ensuring efficient and efficient application development.
Basics of Java Syntax
The grammar of Java is comparable to those of other C-based computer languages, such as C++ and C#. One or more classes, each with methods defining the program’s behavior, make up a Java program. A simple Java application that outputs “Hello, World!” to the console is shown here:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Let’s break down the key components of this program:
- “public class HelloWorld” : Declares a class named HelloWorld.
- “public static void main(String[] args)” : Declares the main method, which is the entry point of the program.
- “System.out.println(“Hello, World!”);” : Prints “Hello, World!” to the console.
Variables, Data Types, and Operators
Java offers a wide range of data types, including reference types (such as String, arrays, and objects) and basic types (such as int, double, and boolean). Variables may be declared to hold various kinds of data, and operators can be used to manipulate the data. As an illustration:
int age = 30;
double price = 9.99;
boolean isStudent = true;
String name = "John";
Control Flow Statements
In a Java program, control flow statements let you manage how the program is executed. Java allows looping statements (for, while, do-while), switch-case statements, and conditional statements (if-else). As an illustration:
int x = 10;
if (x > 0) {
System.out.println("Positive");
} else if (x < 0) {
System.out.println("Negative");
} else {
System.out.println("Zero");
}
Exploring Object-Oriented Programming (OOP) in Java
The programming paradigm known as object-oriented programming, or OOP, is based on the idea that objects are representations of actual entities in the real world with characteristics and actions. Java is an object-oriented language, that supports encapsulation, inheritance, and polymorphism, among other OOP concepts.
Classes and Objects
A class in Java is an object creation blueprint. It outlines the characteristics and actions that class objects will exhibit. Here’s an illustration of a basic Java class:
public class Car {
String make;
String model;
int year;
public void drive() {
System.out.println("Driving the " + year + " " + make + " " + model);
}
}
In this example, “Car” is a class that defines three attributes (‘make’, ‘model’, ‘year’) and one method (‘drive’). You can create objects of this class and access their properties and methods as follows:
Car myCar = new Car();
myCar.make = "Toyota";
myCar.model = "Camry";
myCar.year = 2022;
myCar.drive();
This will output: Driving the 2022 Toyota Camry.
Encapsulation, Inheritance, and Polymorphism
Encapsulation is the process of using methods to expose just the functionality that is essential while concealing the internal state of an object. Encapsulation in Java is achieved by designating class members (methods and attributes) as private and making them accessible to the public via getter and setter methods. Below is the example with Java Encapsulation:
class Person {
// Encapsulating the name and age
private String name;
private int age;
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
}
public class Main {
public static void main(String[] args)
{
// person object created
Person person = new Person();
person.setName("John");
person.setAge(30);
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
Inheritance allows you to create new classes (derived classes) based on existing classes (base classes). The derived classes inherit the properties and behaviors of the base class and can override or extend them as needed. In the below example of inheritance, class Bicycle is a base class, class MountainBike is a derived class that extends the Bicycle class and class Test is a driver class to run the program.
class Bicycle {
public int gear;
public int speed;
// the Bicycle class has one constructor
public Bicycle(int gear, int speed)
{
this.gear = gear;
this.speed = speed;
}
public void applyBrake(int decrement)
{
speed -= decrement;
}
public void speedUp(int increment)
{
speed += increment;
}
public String toString()
{
return ("No of gears are " + gear + "\n"
+ "speed of bicycle is " + speed);
}
}
class MountainBike extends Bicycle {
public int seatHeight;
public MountainBike(int gear, int speed,
int startHeight)
{
// invoking base-class(Bicycle) constructor
super(gear, speed);
seatHeight = startHeight;
}
public void setHeight(int newValue)
{
seatHeight = newValue;
}
// overriding toString() method
@Override public String toString()
{
return (super.toString() + "\nseat height is "
+ seatHeight);
}
}
public class Test {
public static void main(String args[])
{
MountainBike mb = new MountainBike(3, 100, 25);
System.out.println(mb.toString());
}
}
Polymorphism allows objects of different types to be treated as objects of a common superclass. It enables you to write code that works with objects of multiple classes without knowing their specific types at compile time. Polymorphism is achieved in Java through method overriding and method overloading.
class Bike{
void run(){System.out.println("running");}
}
class Splendor extends Bike{
void run(){System.out.println("running safely with 60km");}
public static void main(String args[]){
Bike b = new Splendor();//upcasting
b.run();
}
}
Anchoring the Basics: Foundations of Java Mastery
As our journey begins, we must first lay the groundwork for our Java mastery. Like a skilled architect laying the cornerstone of a magnificent edifice, we shall familiarize ourselves with the basic syntax and structure of the Java language. From variables and data types to control flow constructs, we shall traverse the landscape of foundational concepts with confidence and determination.
But mastery, dear traveler, is not merely a matter of rote memorization; it is a journey of understanding and enlightenment. We shall delve deep into the inner workings of Java, unraveling its secrets and deciphering its mysteries. With each passing lesson, we shall gain insight into the language’s nuances and intricacies, honing our skills with every line of code we write. We’ll go into greater detail on core Java ideas and programming methods in this section, which will help you grasp the language and prepare you for more difficult subjects.
Working with Arrays and Collections
Arrays: In Java, arrays are essential data structures that let you store and work with sets of the same kind of elements. Since arrays have a fixed size, when you define them, you have to indicate how many elements they will contain. Here’s an illustration of how to add and get entries from an array:
int[] numbers = {1, 2, 3, 4, 5};
System.out.println("First element: " + numbers[0]);
System.out.println("Length of array: " + numbers.length);
Collections: Compared to arrays, collections offer greater versatility since they let you store and dynamically resize components of various sorts. The java.util package in Java offers a wide range of collection classes, such as ArrayList, LinkedList, HashMap, HashSet, and others. Here is an instance of how to use an ArrayList:
import java.util.ArrayList;
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
System.out.println("First name: " + names.get(0));
System.out.println("Size of list: " + names.size());
Exception Handling and Error Management
Handling Exceptions: The ability to handle exceptions is essential for creating dependable Java programs. Try-catch blocks are a method built into Java to handle exceptions. Control is moved to the catch block, where you may handle the exception properly when it arises inside a try block. As an illustration, consider this:
try {
int result = 10 / 0; // ArithmeticException: division by zero
} catch (ArithmeticException e) {
System.out.println("An error occurred: " + e.getMessage());
}
Throwing Exceptions: The throw keyword can also be used to explicitly throw exceptions when indicating abnormal situations in your code. This enables you to propagate exceptions to handlers at higher program levels. As an illustration, consider this:
public void withdraw(double amount) throws InsufficientFundsException {
if (amount > balance) {
throw new InsufficientFundsException("Not enough funds in account");
}
// Withdraw logic
}
Introduction to File I/O
Reading and Writing Files: Java has classes for reading from and writing to files, enabling you to work with streams, binary files, and text files, among other external data sources. Classes supporting file I/O operations, such as FileInputStream, FileOutputStream, FileReader, and FileWriter, are included in the java.io package. This is an illustration of how to read text from a file:
try (BufferedReader reader = new BufferedReader(new FileReader("data.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
Handling File Operations
To avoid memory leaks and resource fatigue while interacting with files, it’s crucial to handle errors and terminate resources appropriately. The try-with-resources statement, which was first included in Java 7, allows Java to automatically terminate resources such as file streams when they are not in use. As an illustration, consider this:
try (BufferedReader reader = new BufferedReader(new FileReader("data.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
Ascending the Summit: Conquering Advanced Java Concepts
As we journey ever onward, we shall ascend the lofty peaks of Java mastery, scaling heights previously thought unattainable. Along the way, we shall encounter challenges and obstacles that will test our resolve but fear not, for every trial is an opportunity for growth.
We shall explore the realms of object-oriented programming, mastering the art of encapsulation, inheritance, and polymorphism. Like skilled artisans sculpting a masterpiece from raw marble, we shall craft elegant and efficient class hierarchies that embody the essence of our designs.
But our quest for mastery continues, for Java is a language rich with advanced features and techniques waiting to be discovered. We shall venture into multithreading and concurrency, mastering the art of parallelism and synchronization. We shall harness the power of Java’s vast ecosystem of libraries and frameworks, unleashing the full potential of our programming prowess.
Multithreading and Concurrency
Understanding Threads and Processes
Java applications may run many threads concurrently thanks to multithreading, which enhances responsiveness and makes better use of available resources. A Java Virtual Machine (JVM) allows for the simultaneous operation of several threads, each of which is a lightweight process inside another process.
Creating and Managing Threads
Java has many thread creation and management methods. To specify the code that will execute on a different thread, you may either implement the Runnable interface or extend the Thread class. This is an illustration of how to use the Runnable interface to create a thread:
public class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running");
}
}
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(new MyRunnable());
thread.start();
}
}
Synchronization and Thread Safety
When several threads access shared resources at once, concurrency poses the possibility of racial conditions and corrupted data. Java offers synchronization features like locks and synchronized blocks to guarantee thread safety and avoid inconsistent data.
Networking and Socket Programming
Building distributed systems and facilitating communication between apps across a network need networking. With classes like Socket, ServerSocket, and InetAddress in the java.net package, Java offers strong networking capabilities.
Creating Client-Server Applications
You may write client-server Java programs for TCP/IP and UDP, among other network protocols. Sockets can be used to create network connections and facilitate data transmission between clients and servers. An illustration of a basic TCP client-server program is provided here:
// Server code
ServerSocket serverSocket = new ServerSocket(8080);
Socket clientSocket = serverSocket.accept();
InputStream inputStream = clientSocket.getInputStream();
OutputStream outputStream = clientSocket.getOutputStream();
// Client code
Socket socket = new Socket("localhost", 8080);
InputStream inputStream = socket.getInputStream();
OutputStream outputStream = socket.getOutputStream();
Java Applications and Database Connectivity(JDBC)
Java apps may communicate with relational databases using the JDBC Java API. It offers a collection of classes and interfaces for handling database connections, running SQL queries, and getting results. Here’s an illustration of how to open a database connection and run a query:
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
Managing Database Interactions and Transactions
You may run several SQL statements as part of a single transaction thanks to JDBC’s support for transaction management. The commit() and rollback() methods of the Connection object allow you to commit or rollback transactions according on whether they succeed or fail.
The Quest for Knowledge: A Lifelong Endeavor
As we near the end of our journey, let us pause to reflect on the path we have traveled and the lessons we have learned. We have delved into the depths of Java’s syntax and semantics, scaled the dizzying heights of object-oriented programming, and explored the uncharted territories of advanced topics and techniques.
But let us not forget that the quest for Java mastery is not a destination but a journey – a lifelong endeavor fueled by curiosity, passion, and a thirst for knowledge. As we bid farewell to this chapter of our odyssey, let us do so with a sense of pride and accomplishment, knowing that the journey continues ever onward.
Exploring Advanced Frameworks and Libraries
Java has a thriving ecosystem of frameworks and libraries that make development easier and address common problems. While libraries like Apache Commons and Google Guava offer utility classes and methods for a variety of tasks, frameworks like Spring, Hibernate, and Apache Struts provide extensive support for developing web applications.
Leveraging Advanced Tools and Technologies
It is essential for Java developers to keep up with the newest tools and technologies available in the ecosystem. IDEs that improve productivity and efficiency include IntelliJ IDEA and Eclipse, which provide strong functionality for code editing, debugging, and refactoring. Build technologies, such as Maven and Gradle, streamline project management and collaboration by automating the build process and managing dependencies.
Building Real-World Projects
Working on real-world projects is one of the best methods to improve your grasp of Java and acquire real-world experience. Whether you’re creating a mobile app, web application, or contributing to an open-source project, having practical experience enables you to apply your knowledge in relevant contexts and get insight from real-world problems and situations.
Embracing Software Design Patterns
Design patterns are tried-and-true fixes for typical design issues that arise during software development. You can create scalable, reliable, and stable Java programs by being familiar with design patterns like Factory, Observer, Singleton, and Strategy. Design patterns make it easier to reuse and maintain code by giving designers a consistent vocabulary to express their ideas.
Design Patterns: A Practical Guide
It’s crucial to successfully implement design patterns in your projects after you grasp their underlying ideas. Look for ways to reorganize your codebase and, as necessary, add design patterns. You may write code that is simpler to read, edit, and expand by using well-established design patterns and principles. This lowers the likelihood of errors and defects developing in the future.
Getting Knowledge from Case Studies and Real-World Examples
Examining case studies and real-world examples of design patterns in use can give important insights into the advantages and practical uses of these patterns. To learn from other developers’ experiences, investigate open-source projects, peruse books and articles, and take part in online forums and communities. You may learn more about design patterns’ advantages, disadvantages, and best practices by examining how they are applied in active codebases.
Contributing to the Java Community
The global Java community is lively and welcoming, with developers, instructors, enthusiasts, and supporters from many walks of life. Collaboration, creativity, and development are encouraged when one gives back to the Java community through open-source initiatives, information exchanges, and mentoring. You can give back to the community, broaden your network, and make a lasting impact on the Java ecosystem by taking part in community-driven initiatives like arranging conferences or meetups, writing articles or tutorials, organizing open-source projects on GitHub, or mentoring aspiring developers.
The Odyssey Continues . . .
And so, dear traveler, as we bring our journey to a close, let us remember that the pursuit of Java mastery is not a solitary endeavor but a shared voyage of discovery. Let us embrace the challenges that lie ahead, knowing that with each obstacle overcome, we grow stronger and wiser.
May this guide serve as a beacon to illuminate your path on the road to Java mastery. May it inspire you to push the boundaries of your knowledge and explore the depths of your potential. And may your journey be filled with wonder, excitement, and endless growth opportunities.
You can start your Java journey here: “Java beginner to advanced: A Comprehensive Guide“.