Tutorial Series: Java OOP
Java is a powerful object-oriented programming (OOP) language that is widely used for developing large-scale applications. OOP is a programming paradigm that revolves around the concept of objects, which are instances of classes that encapsulate data and behavior.
In Java, everything is an object, including primitive types such as integers and characters. This means that every object in Java has a state (represented by its data) and behavior (represented by its methods). By defining classes, developers can create new types of objects that can interact with each other and with objects from other classes.
One of the key benefits of OOP in Java is code reuse. By using inheritance, developers can create new classes that inherit properties and behavior from existing classes, allowing them to reuse code and avoid duplicating effort. Polymorphism is another important concept in OOP, which allows objects to take on different forms depending on the context in which they are used.
Encapsulation is also an important concept in Java OOP, which involves hiding the implementation details of a class from other classes. This makes it easier to maintain and modify the codebase and can help to prevent bugs and security vulnerabilities.
Java also supports interfaces, which are contracts that define a set of methods that a class must implement. This allows for polymorphism and code reuse in a flexible and extensible way. Additionally, Java supports abstract classes, which are classes that cannot be instantiated but can be used as a blueprint for creating other classes.
Java OOP is a powerful tool for creating complex, maintainable, and scalable applications. By using OOP concepts such as inheritance, polymorphism, and encapsulation, developers can create code that is easy to read, maintain, and extend.
Introduction To Object Oriented Programming In Java
A Sneak Peek Of Objects in Java
Class In Object Oriented Programming
A More In-Depth Inspection Of Class In Java
FAQs About Classes In Java
Class Fields in Java
Memory Management In Java
Methods In Java
Java OOP - Introduction To Arrays
Constructors In Java
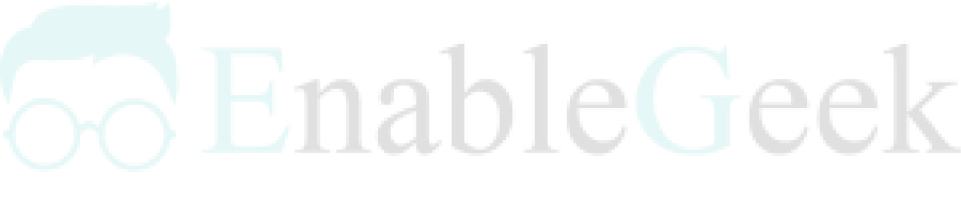
Check Our Ebook for This Online Course
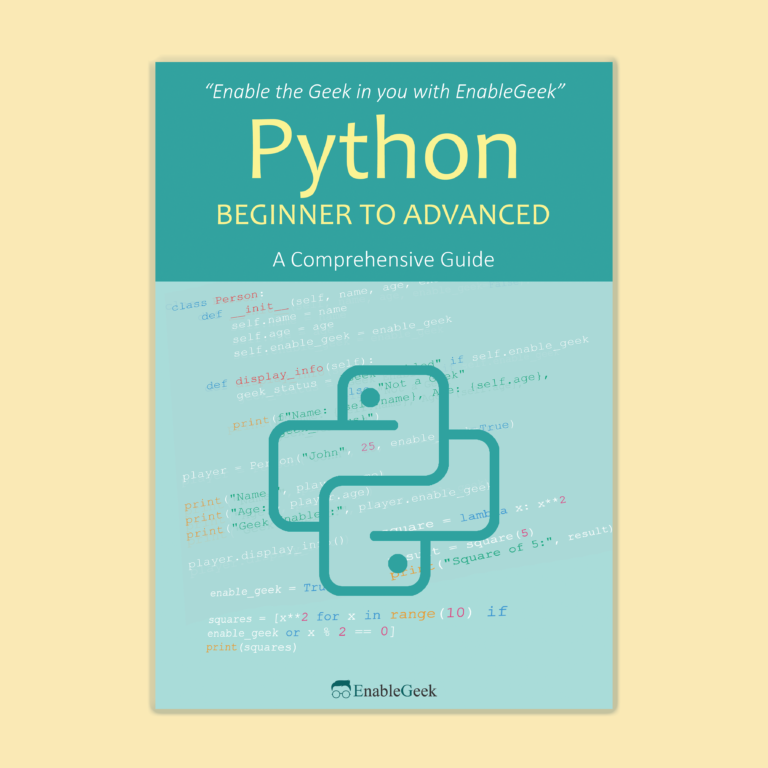
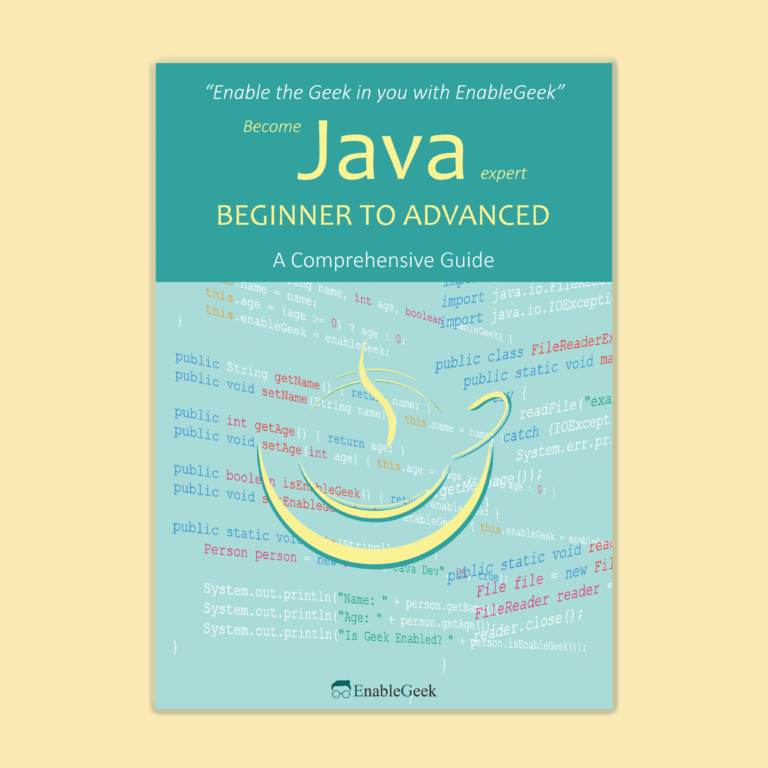
Advanced topics are covered in this ebook with many examples.