Tutorial Series: Python Advanced
Python is a versatile and powerful programming language that can be used to build a wide range of applications, from simple scripts to complex systems. In the advanced level of Python, you will learn about more complex programming concepts that are essential for building large-scale applications. Some of the key topics covered at this level include:
Object-oriented programming: This is a programming paradigm that focuses on the creation of objects, which have their own attributes and methods. You will learn how to design and implement classes, and how to use inheritance and polymorphism to create reusable code.
Functional programming: This is a programming paradigm that focuses on the use of pure functions, which do not have side effects and only depend on their input. You will learn how to use higher-order functions, closures, and lambda expressions to create more modular and reusable code.
Concurrency and parallelism: These are essential concepts for building high-performance applications that can take advantage of modern hardware. You will learn how to use threads, processes, and asynchronous programming to create applications that can handle multiple tasks simultaneously.
Data structures and algorithms: These are essential concepts for building efficient and scalable applications. You will learn about different data structures such as arrays, linked lists, trees, and graphs, and different algorithms such as sorting, searching, and graph traversal.
Networking and web development: These are essential concepts for building networked and web-based applications. You will learn how to use sockets, HTTP requests, and web frameworks like Flask and Django to build server-side applications and APIs.
Overall, the advanced level of Python is essential for anyone who wants to build large-scale and complex applications. By mastering these concepts, you will be able to build efficient, scalable, and maintainable code that can handle a wide range of tasks and requirements.
Python Advanced: Improve Your Python Programming Knowledge
Python Advanced: How to use Lambda Function with python Methods
Python Advanced: How to use Doctest and Unit Test in Python
Python Advanced: What is Test-Driven Development (TDD) with Python
Python Advanced: What is the Zip method in Python
Python Advanced: How to Utilize Memoization Technique in Python
Python Advanced: What is Reflection in Python Programming
Python Advanced: What are the Coroutine and Subroutine in Python
Python Advanced: How to use the Currying Method in Python
Python Advanced: What is the Garbage Collection in Python Programming
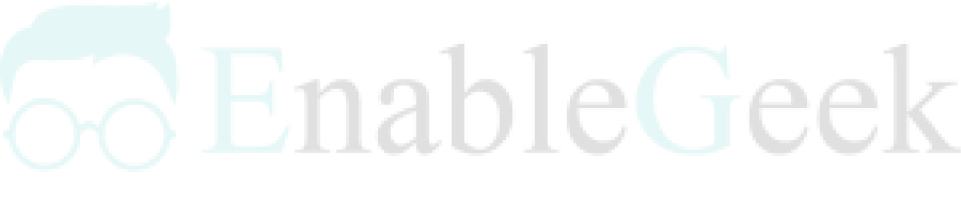
Check Our Ebook for This Online Course
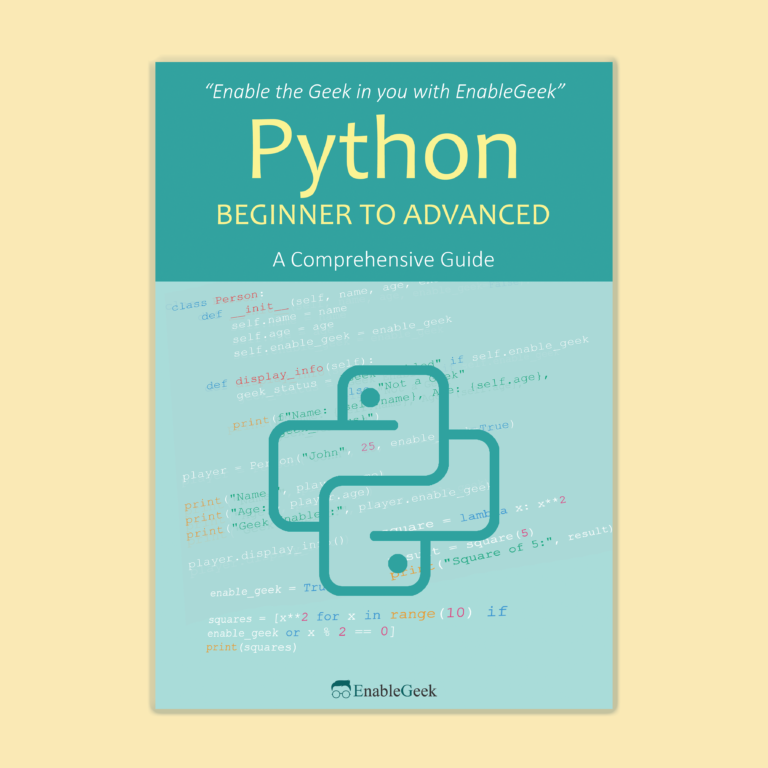
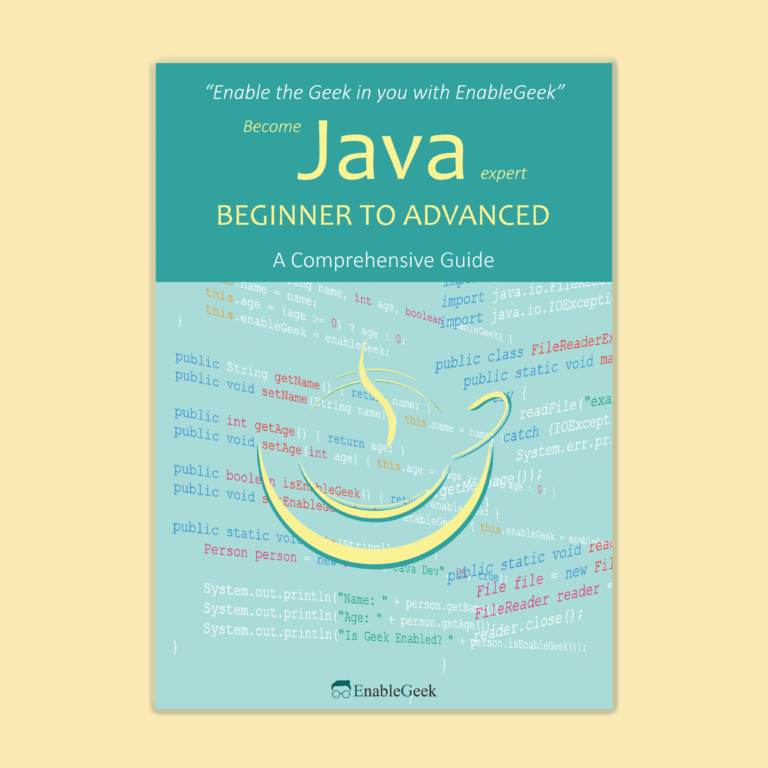
Advanced topics are covered in this ebook with many examples.