A Coroutine in Python
Function, which is also referred to as a subroutine, procedure, subprocess, etc., is a term that we are all familiar with. A function is a set of instructions that are packaged together to carry out a certain activity. These functions are known as helper functions or subroutines when the logic of a complex function is broken down into a number of independent steps that are also functions.
In Python, the main function, which is in charge of coordinating their use, calls the subroutines. There is just one entry point for a subroutine.
The generalizations of subroutines are called coroutines. They are used for cooperative multitasking, which allows multiple applications to run simultaneously by allowing a process to willingly relinquish (give away) control regularly or while it is inactive.
Coroutine and subroutine distinctions are:
- Coroutines provide numerous entry points for pausing and resuming operations, whereas subroutines do not. A coroutine can pause its operation, hand off control to another coroutine, and then pick up where it left off when it resumes.
- Unlike subroutines, coroutines don’t have a main function that may call them in a specific order and coordinate the outcomes. Cooperative indicates that coroutines connect to one another to build a pipeline. Data input from one coroutine may be sent to another for processing by another coroutine. A coroutine to display the outcome might then be present.
Illustration for Subroutine Process
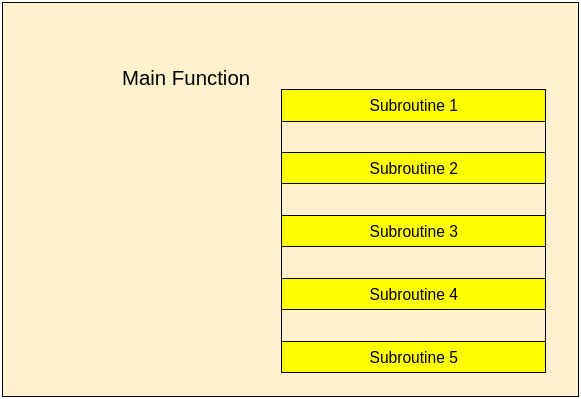
Illustration for Coroutine Process
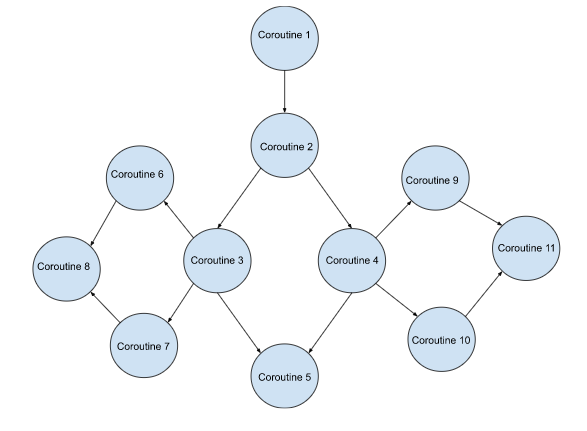
Discover more Python tips and elevate your coding skills with our comprehensive guide, “Python Beginner to Advanced.” Whether you’re a beginner or an experienced developer, this book covers everything from basic concepts to advanced techniques. And if you’re interested in expanding your programming collection, don’t miss out on our “Java Beginner to Advanced” guide as well!
Application of Coroutine
Coroutines in Python are similar to generators but have a few extra methods and use yield statements a little differently. Coroutines can also consume data, while generators can provide data for iteration.
The yield statement was slightly altered in Python 2.5 and can now be used as an expression. For instance, the assignment’s right side should read:
statement = (yield)
The expression “(yield)” captures and returns any value we pass to the coroutine.
The send() method allows a value to be passed to the coroutine. Take this procedure, for instance, which outputs out names with the prefix “Dear” in them. Using the send() method, we will transmit names to the coroutine.
Coroutine Execution
The generator and coroutine both operate in a similar way. Nothing happens when we call a coroutine; it merely responds to the next() and sends() methods. This is evident in the example above since our coroutine doesn’t begin running until we call the __next__() method. Following this call, the first yield expression is reached; at this point, the execution pauses as it awaits the value’s transmission to the co_routine object. It looks for a prefix when the first value is provided to it and prints the name if one is found. The name is printed, and then the loop is repeated until the C_name = (yield) expression is encountered once again.
Example:
def coroutine_name(name):
print("Searching prefix:{}".format(name))
while True:
C_name = (yield)
if name in C_name:
print(C_name)
co_routine = coroutine_name("Python")
co_routine.__next__()
co_routine.send("Python is a powerful programming language.")
co_routine.send("Java is the great one!")
co_routine.send("Kotlin is evergreen.")
co_routine.send("Python has a lot of fields for building applications.")
co_routine.send("Python is vastly used in data science.")
Output:
Searching prefix:Python
Python is a powerful programming language
Python has a lot of fields for building applications.
Python is vastly used in data science.
Closing a Routine
The coroutine’s close() method is used to stop it from running endlessly. When a coroutine is closed, a GeneratorExit exception is generated, which can be handled as usual. If we attempt to submit values after stopping the coroutine, the StopIteration exception will be thrown.
Example:
def coroutine_name(name):
print("Searching prefix:{}".format(name))
try :
while True:
C_name = (yield)
if name in C_name:
print(C_name)
except GeneratorExit:
print("Closing coroutine!!")
co_routine = coroutine_name("Python")
co_routine.__next__()
co_routine.send("Python is a powerful programming language")
co_routine.send("Java is the great one!")
co_routine.send("Kotlin is evergreen.")
co_routine.send("Python has a lot of field of build application.")
co_routine.send("Python is vastly used in data science")
co_routine.close()
Output:
Searching prefix:Python
Python is a powerful programming language
Python has a lot of field of build application.
Python is vastly used in data science
Closing coroutine!!
Thread vs Coroutine
You might be wondering now how coroutines differ from threads since they appear to do the same task.
In the case of threads, the scheduler is followed by an operating system (or run-time environment) that alternates between threads. The decision to switch between coroutines in a coroutine is made by the programmer and the programming language. Coroutines cooperate to do multiple tasks by pausing and starting again at predetermined programmatic points.
Threads and coroutines are extremely similar. Coroutines, however, multitask cooperatively, whereas threads often multitask preemptively. Concurrency is offered via coroutines, but not parallelism. Coroutines have several advantages over threads, including the ability to be used in hard real-time environments (switching between coroutines need not involve any blocking or system calls), the lack of dependence on the operating system for support, and the lack of synchronization primitives like mutexes and semaphores for the protection of critical sections.
The suitability for real-time operation and the relative cheapness of switching between them will be lost, but it is still conceivable to build coroutines using preemptively scheduled threads in a fashion that is invisible to the calling code.
Subroutine vs Functions
In programming, a subroutine and a function are both kinds of procedures, or named blocks of code, that perform a specific task. The main difference between a subroutine and a function is the return value. A subroutine does not return a value to the caller, whereas a function returns a value to the caller.
Subroutines are typically used to break down larger tasks into smaller, more manageable tasks. They can be called from other parts of the program as many times as needed. Subroutines are useful when you need to perform a task that does not require a return value, such as displaying output or updating a database.
Functions, on the other hand, are used to perform calculations and return a value to the caller. Functions can be called from other parts of the program and can be used to perform complex calculations or data manipulations. Functions are useful when you need to perform a calculation or return a value to the caller.