Tutorial Category: Data Structure
Data structures are a fundamental concept in computer science and are used to organize and store data in a way that makes it efficient and easy to access. A data structure is a way of organizing and storing data in memory so that it can be used efficiently by programs. Different data structures are designed to handle different types of data, and each has its own strengths and weaknesses.
Common data structures include arrays, linked lists, stacks, queues, trees, and graphs. Arrays are a simple data structure that stores data in a linear fashion, whereas linked lists store data in a series of nodes that point to each other. Stacks and queues are specialized data structures that store data in a Last-In-First-Out (LIFO) or First-In-First-Out (FIFO) order, respectively.
Trees and graphs are more complex data structures that allow for more sophisticated organization of data. Trees are hierarchical structures that have a root node and one or more child nodes, while graphs are a more general structure that consist of nodes and edges that connect them.
Choosing the right data structure is an important part of programming, as it can have a significant impact on the performance and efficiency of a program. Different data structures are better suited to different tasks, and choosing the right one can make a program run faster, use less memory, and be easier to understand and maintain.
In addition to basic data structures, many programming languages also provide built-in data structures such as dictionaries, sets, and tuples. These data structures can be used to store and manipulate data in a way that is optimized for the specific needs of the programming language or application.
Edge List
Adjacency Matrix
Adjacency List
Linked List
Doubly Linked List
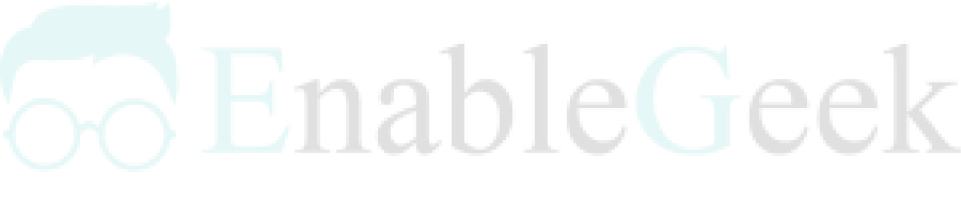
Check Our Ebook for This Online Course
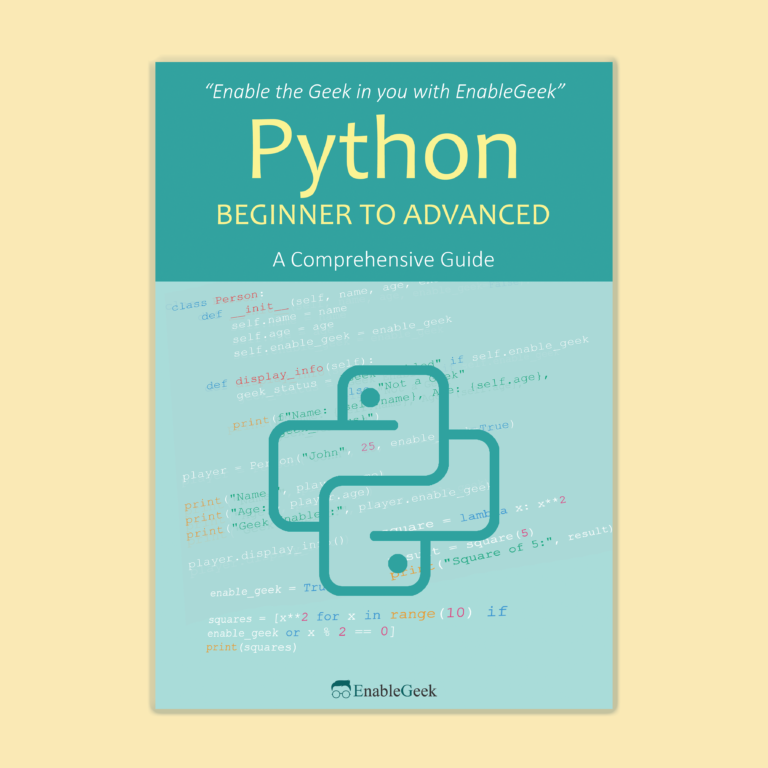
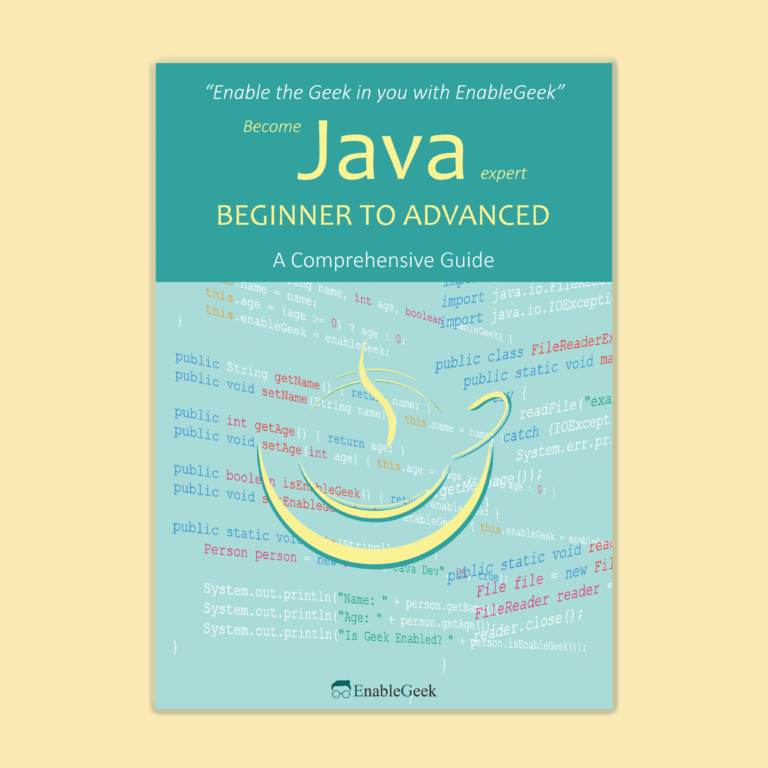
Advanced topics are covered in this ebook with many examples.