Tutorial Series: Java Intermediate
Java Intermediate is a level of proficiency in the Java programming language that requires a deeper understanding of the language and its various features. At this level, developers are expected to be able to use Java to develop more complex applications and solve more difficult programming problems.
One key area of focus at the intermediate level is Java collections. Collections are built-in data structures that are used to store and manipulate groups of objects. Understanding collections is essential for developing applications that deal with large amounts of data, as well as for performing common programming tasks such as sorting and searching.
Another important concept at the intermediate level is concurrency. Java provides built-in support for creating multi-threaded applications, which can be used to take advantage of modern multi-core processors and improve performance. However, writing concurrent code can be complex and error-prone, so developers must be familiar with concepts such as synchronization, locks, and threads to create code that is safe and efficient.
At the intermediate level, developers must also be familiar with common design patterns and best practices. These include principles such as SOLID, DRY, and YAGNI, which can be used to create maintainable, scalable, and extensible code. Additionally, developers must be able to use tools such as Git, Maven, and Gradle, which are used for version control, dependency management, and building Java applications.
Finally, at the intermediate level, developers must be familiar with Java frameworks and technologies that are commonly used in the industry. These include frameworks such as Spring and Hibernate, which are used for building enterprise-level applications, as well as technologies such as RESTful web services and JPA, which are used for building scalable and flexible web applications.
Java Intermediate is a level of proficiency in the Java programming language that requires a deeper understanding of the language and its features. By mastering concepts such as collections, concurrency, and design patterns, as well as tools and frameworks used in the industry, developers can create more complex and sophisticated applications that meet the evolving needs of users and businesses.
A Brief Introduction To Java IO
Network Programming In Java
Concurrent Programming in Java - PART ONE
Concurrent Programming in Java – PART TWO
Concurrent Programming in Java - PART THREE
Concurrent Programming In Java - PART FOUR
Concurrent Programming In Java - PART FIVE
GUI Programming In Java - PART ONE
GUI Programming In Java - PART TWO
GUI Programming In Java - PART THREE
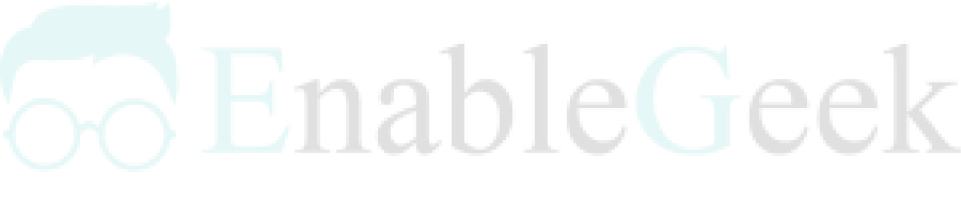
Check Our Ebook for This Online Course
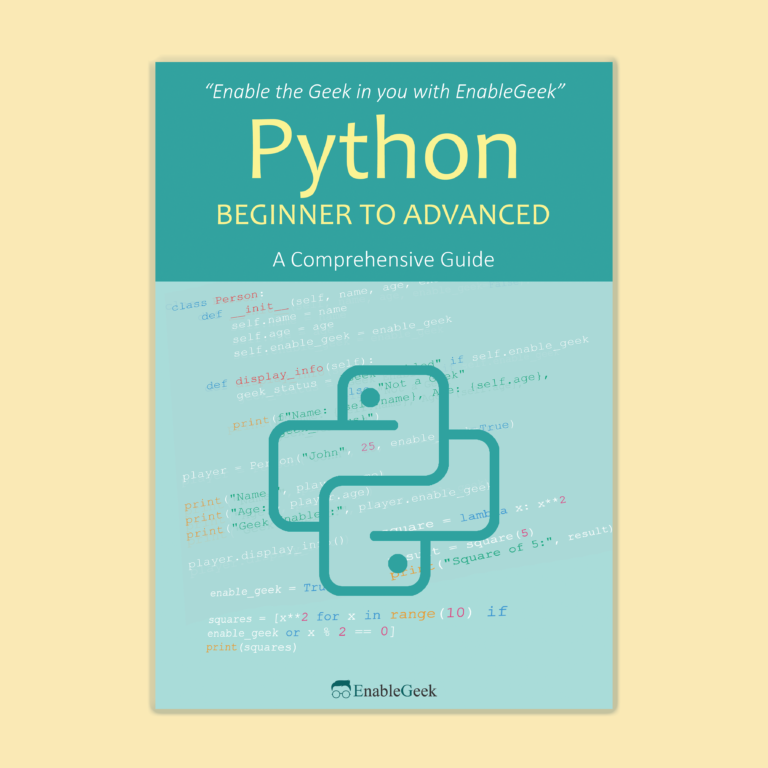
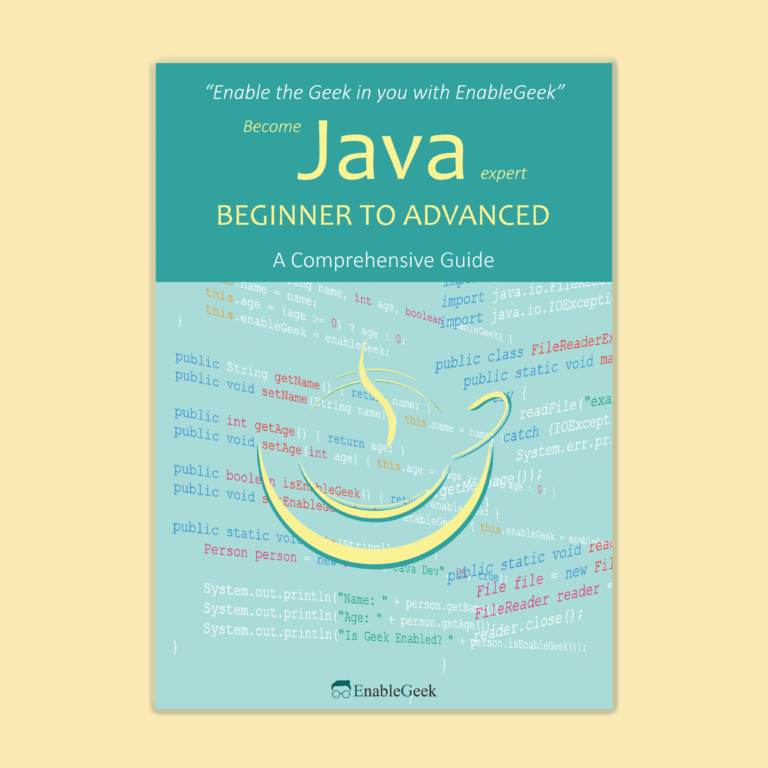
Advanced topics are covered in this ebook with many examples.