We have this concept of variables and constants in programming. If you have done high school algebra then you might be somewhat familiar with this concept. The concept of variables in mathematics differs from the concept of variables in programming though. The first one is abstract, having no reference to physical objects such as storage location.
First, let us understand the concept of constants and variables in a generic way. Data values can be either constant or variable. If the user or the program can change the data values, then they are called variables. If the data values stay the same every time a program is executed, then they are constants.
An understanding of data types is a prerequisite for this tutorial.
Constant
It is expected that constants do not change. The unit of gravity, the PI value, speed of light are some examples of constants. They do not change. In programming, constants can be classified into two categories.
Variable
During the execution of a program, data values may change. For example, suppose a program asks a user for a user name, it will be different for each different user.
In layman’s terms, the variable is a name used to identify the location of your actual data. How the concept of a variable can be associated with memory storage location is discussed below.
In our discussion of a contiguous group of bits, we considered each “bit” as a box. In this tutorial, we consider each “byte” as a “cell” in an array of bytes.
We are briefly going to discuss the array here. It will help you understand the following sections better. An array is a collection of similar data elements stored at contiguous memory locations. It is the simplest data structure where each data element can be accessed directly by only using its index number. Suppose we have 10 bytes(not bits). And these 10 bytes are placed next to each other. This is an example of an array or more specifically a byte array. The following diagram demonstrates this unique concept.
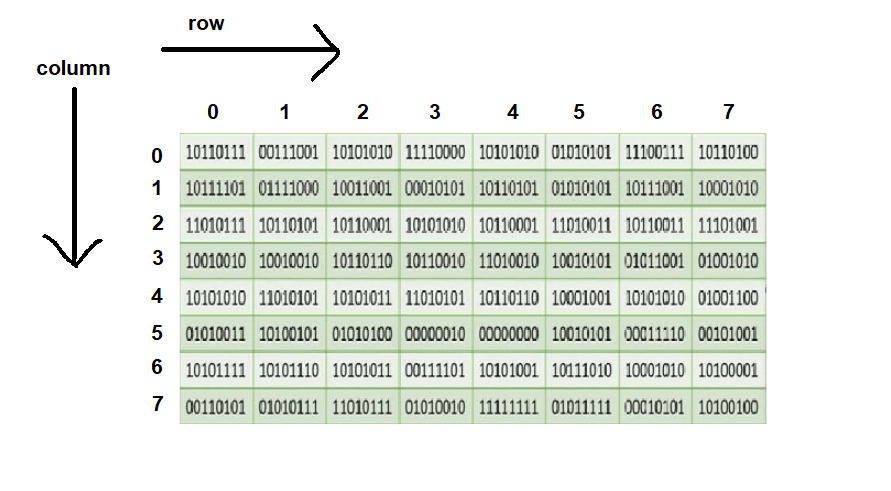
In the above figure, a total of 64 contagious bytes are stored in an 8 by 8 matrix format. They are stored in contagious memory locations in the RAM. So they can be regarded as an array, or in this case array of bytes or byte array. Each cell of the array stores 8 bits as can be seen in the above figure. Each cell can be accessed by its row and column indices. For each cell the indexes are unique. Note that the index number starts from 0, not from 1. This is the norm for an array. Indices for an array start from 0.
During the execution of a program, we know that data is held in RAM. Now we will try to understand how the program locates these values in memory to be processed by the CPU. Main memory or ram consists of a large array of bytes(each byte consists of 8 consecutive bits). The address of each byte or cell in the array of bytes can be thought of as the index of each array element or byte within the array of bytes.
Remember, when we talk about memory location, we talk about a byte (8 contiguous bits ), not a bit.
Our modern-day computers are typically equipped with many gigabytes of memory, and each byte of memory has a unique address associated with it. Now you may be wondering how other data types such as integers, doubles, etc are addressed as they consist of more than one byte.
Java has 8 primitive data types. How all those data types are addressed in the memory when that types of data are stored in the memory? More specifically, say, how an integer data type is addressed in THE RAM.
If the data type stored in the memory is bigger than a byte, which is most often the case, the address of each data type is the index of the first byte of that data type stored in the memory. If we want to store an integer in the memory, we need 32 contagious bits(4 contagious bytes). So, if an integer takes the cells(each cell size is 1 byte or 8 bits wide) 316, 317, 318, and 319, then that integer data type is addressed using only the address of the first byte/cell. But data will be written on all four bytes starting from cell 316 through 319 in a series of 32 contagious bits.
Most data types stored in the RAM are larger than a byte. It means they need more than 8 contagious bits to store data in the memory. The same rules apply to them all.
The amount of memory a computer has as well as how the computer addresses the memory locations are physical properties of the hardware. We can not change the memory address of a memory location in the RAM as it is a property of the hardware, but the data stored in a memory location can change or vary over time. In simple words, we can replace previously stored data in a cell(byte) with our new data, whenever we want to. That cell would forget about the previous data and treat the newly stored data as if it has been always there in that specific cell. Again, for an integer, it would concern four bytes or four cells. The mechanism is the same.
Suppose, to properly perform a task, our program needs some data, say an Integer number whose value is 23. Now, the data is stored in the RAM, and the CPU can directly access and process it. To access the data in the memory the CPU must know where the data is saved in the memory, called the memory address of that data. As we mentioned earlier, modern computers have memory in gigabytes. So the total storable and accessible memory address available is about 4 billion(in other words 4 billion bytes of data can be stored/accessed in this memory). That memory address is addressed using the hexadecimal format for convenience – 0xaacc2270(nothing to do there). This number looks scary, right? Moreover, to further complicate the addressing situation, every time a program is executed, a new random location is allocated for that data, giving us a new memory address associated with that data. Our CPU can handle this situation. But we can not. We, humans, can not possibly keep track of the memory address, expressed in some weird hexadecimal format, where the data is stored.
Let us realize how complicated this situation is. To realize that, consider we need an integer type data, say 23, in our program. We must store this data in the RAM so that processor can access it and process it. For our example’s sake suppose 23 is saved in the memory location: 0xaacc2270. This memory location is randomly allocated in the RAM. We can not do anything about it. 23 is converted to a 32-bit pattern and stored in the randomly allocated space.
The 32-bits representation of 23 is: 00000000000000000000000000010111
If we represent 23 as an integer or in 4 bytes, then the representation would be: 00000000 00000000 00000000 00010111
Data is stored in bits like this in the location: 0xaacc2270. Now, you may want to replace the value at this location, i.e., 0xaacc2270.
Suppose, you know the memory address where this number is saved in the main memory: 0xaacc2270. You can directly access this storage location using the memory address and replace 23 with a new value, say, 29. Of course, the bits pattern will change in that location.
The 32-bits representation of 29 is: 00000000000000000000000000011101
If we represent 29 as an integer or in 4 bytes, then the representation would be: 00000000 00000000 00000000 00011101
But here is a catch. Every time we run a program, the memory is allocated randomly. Suppose, we execute the program again, 23 will be stored in the memory again, but this time 23 will be saved in a different location in the memory. In other words, it would not be in the address: 0xaacc2270. But the bits and bytes pattern will remain the same. No change there. After all, there is only one way to represent integer 23 in binary digits. Only the memory address will change.
If you want to replace 23 with a new value then you have to know the new memory address. This becomes complicated when the program has to run hundreds of times. You have to keep track of the memory addresses for all those times if you want to use/replace that value. And in a typical application, there are dozens of values that need to be stored in the RAM. So this approach of directly accessing a value in the memory using the memory address of the location it is stored is not efficient at all.
To solve this problem, and make our program more efficient computer scientists in the earlier days came up with a concept called variables. Instead of keeping memorizing all the memory addresses, we defined a new way to ease this complication by associating an alias with a memory location.
In computer programming, a variable is an abstract storage location paired with an associated symbolic name, which contains some known or unknown quantity of information referred to as a value; or in simpler terms, a variable is a container for a particular set of bits or type of data (like integer, float, String etc…). A variable can eventually be associated with or identified by a memory address.
In the following sections, we will try to make sense of this definition of variables in programming. First, we will try to understand the giving of an alias to a memory address. Then we will discuss how variables can be regarded as containers.
A variable is a named location in main memory that has three characteristics - a name, a content and a memory address.
You might have the following statement in a Java program:
int number;
This statement says, “Allocate 4 consecutive bytes in the memory(32 contagious bits) and name that memory location “number” “. What a cool nickname for a memory location, right? We are happy that we do not have to face that weird and scary-looking hexadecimal number format anymore.
Who will do this allocation is not important right now. Just know that someone will do it for us to make our programming life a little bit easier. When the program runs, the computer reserves space for the variable called number somewhere in memory. That location has a fixed address in the memory space, like this: 0xaacc2270. Remember, the memory location is a property of the hardware and we can do anything to change anything. We can however change the value that may be stored in that location.
After giving the memory location an alias, you no longer have to keep track of the memory location every time you execute the program. The variable “number” will be doing this task for you. Every time the program is executed, 32 bits of memory are allocated in the memory space for an integer. We will always call this space “number” and we can access that address by simply calling the variable name, in our case, it is “number”. No matter in what location 32 bits are allocated for an integer, we can access that space just using the alias.
While you think of the variable called the number, the computer thinks of a specific address in memory (for example, 0xaacc2270). Therefore, when you create a statement like this:
number = 23;
The compiler might translate that into, “Load the value 23 into memory location xaacc2270.” The computer is always thinking of memory in terms of addresses and values at those addresses. And by using variables we give those addresses aliases. When you want to replace 23, say, with 29, you simply write,
number = 29;
The compiler again might translate that into, “Load the value 29 into memory location xaacc2270.”
Note: In every execution of a program data will be allocated in random locations. This is hard to work with. So we give those addresses aliases.
No, let us discuss how a variable acts as a container.
Consider java variables as containers. Containers that contain something.
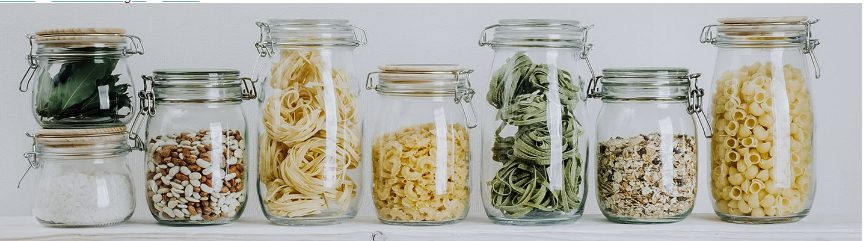
A variable is just a container. It holds something.
A container has a size and type. Depending on a container’s size and type it can hold different types of goods or contents. In this lecture, we will look at the containers that hold primitives type – byte, boolean, integer, character, etc.
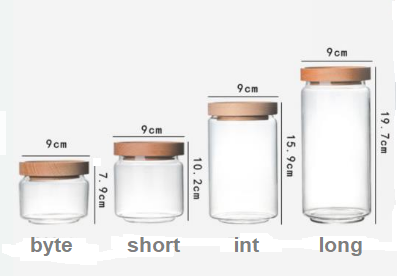
The four containers here are for Java’s four integer primitives.
Each jar holds a value, so instead of saying, “I’d like a tall French roast,” you tell the compiler, “I’d like an int jar(variable) with the value 23 in it, please.” Except for one minor distinction: in Java, you must also give your cup a name. So it’s actually “I’d like an int with the value 23 and the name the jar(variable) “number”.” The sizes for the six numeric primitives in Java are shown below:
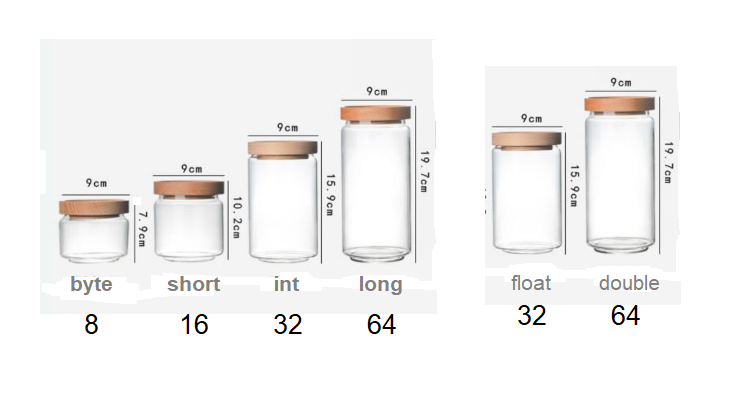
Note : Be sure the value can fit into the variable.
A large value cannot be placed in a small jar(variable). Okay, you can do it, but you’ll lose some. You’ll get spillage, as we say. If the Java compiler can tell from your code that something won’t fit in the container (variable/jar) you’re using, it will try to help prevent this.
For example, you can’t place an int-full of stuff into a byte-sized jar, as follows:
int integerValue = 23;
byte byteValue = integerValue; // won’t work!!
You may be wondering why this does not work!
After all, the value of integer value is 23, which is clearly small enough to fit into a byte. You and we are aware of this, but the compiler is only concerned with the fact that you are attempting to fit a large thing into a small space, with the risk of spilling. Do not expect the compiler to understand what the value of integer values is even if you can see it literally in your code.
So from our study of variables, we can say that variables in Java work like data containers that save the data values during the Java Program Execution. Variables actually can be treated like containers that store data in memory. And they have some cool names, using which we can access the data stored in the memory.
How to declare a Variable?
In Java, every variable needs to be assigned a data type – which determines what type of values can be saved in the variable and the actual that the variable holds. A variable is a memory location name for the data.
We can declare a variable in Java as mentioned in the picture below:
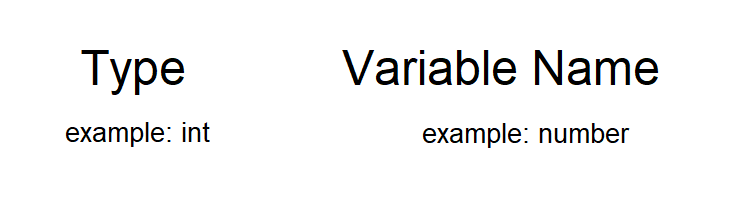
While declaring a variable in java, we have to take care of two things.
- DataType: As Java is statically typed so we must declare the type of data that is stored in a variable beforehand.
- Data_Name: We have to give the variable a name.
In this way, a variable is declared in java. Or, we can say that in this way, we name a memory location for our convenience. Now we will discuss how we can assign or store values in variables.
We can do this basically in two ways:
- Variable initialization
- Assigning value by taking input
How can we Initialize variables?
We can initialize the variable in the following way.
int number = 23;
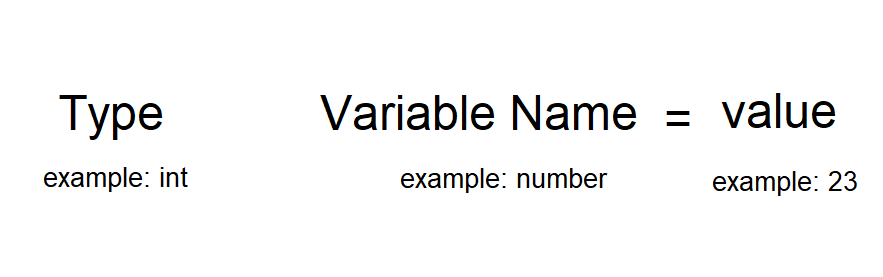
- DataType: As Java is statically typed so we must declare the type of data that is stored in a variable beforehand.
- Data_Name: We have to give the variable a name.
- Value: It is the initial value stored in the variable.
public class Variable{
public static void main(String[] args) {
//Variable declaration and initialization
int number = 23;
}
}
Save this as Variable.java, compile it, and run it. To see the value stored at a variable number add the following line:
public class Variable{
public static void main(String[] args) {
//Variable declaration and initialization
int number = 23;
System.out.println("Value stored at number : "+number);
}
}
Output:
Value stored at number : 23
Naming Convention Of Variables In Java
Variables are the names given to computer memory locations where values are stored in a computer program. It is difficult to properly name variables, but it is necessary. Our code must be as descriptive as possible. Any other developer who may read one of our programs in the future should be able to figure out what our code does. sensible and descriptive variable (and method) names go a long way to serving this purpose.
Our variable names should be descriptive of what they contain while also being concise. This can be difficult to accomplish. You might even be concerned about running out of unique, descriptive, and concise variable names. Don’t worry, all programming languages have what’s known as scope. The term “scope” refers to the fact that not all variables exist everywhere in a program. If they did, a variable you write in file A might accidentally overwrite a variable created by your friend in file B. When you have a scope for variables, you can be more confident that you are not overwriting someone else’s work when you create variables.
We will talk more about scope when we discuss methods in Java. It will not make much sense to you now.
Every programming language has its own set of rules and conventions regarding the kinds of names that can be used for naming variables, and the Java programming language is no exception. The following are the summarized rules and conventions for naming variables:
- In Java variable names are case-sensitive. You can use any legal identifier — an unlimited-length sequence of Unicode letters and digits beginning with a letter, the dollar sign “$,” or the underscore character ” ” as a variable’s name. The convention, however, is to always begin your variable names with a letter rather than “$” or ” “. Furthermore, by convention, the dollar sign character is never used. The dollar sign may appear in some auto-generated names, but you should avoid using it in variable names. A similar convention exists for the underscore character; while it is technically legal to begin your variable’s name with “_”, this practice is discouraged. White space is not permitted.
- The following characters can be letters, digits, dollar signs, or underscores. Conventions (and common sense) also apply to this rule. When naming variables, use full words rather than cryptic abbreviations. This makes your code easier to read and understand. In many cases, it will also make your code self-documenting; for example, variables named number1, and number2 are far more intuitive than abbreviated versions like n1 and n2. Keep in mind that the name you choose cannot contain a keyword or a reserved word.
- If your chosen name consists of only one word, spell it in all lowercase letters. If there are multiple words, capitalize the first letter of each subsequent word. This convention is evident in the variable names gearRatio and current gear. If your variable stores a constant value, such as static final int NUM_GEARS = 6, the convention changes slightly, capitalizing every letter and using the underscore character to separate subsequent words. The underscore character is never used elsewhere by convention.