When we designed this course, we originally planned to teach you structured programming first, before moving on to Object Oriented Programming. We designed this course in this way so that we can teach you the fundamentals of computer science first and advance from there. Building our understanding of Object Oriented Programming concepts on top of that would be more efficient for you. We assumed that you have no prior experience in programming. You are a complete beginner. This course is actually intended for people who are learning Java as their first programming language and who have never programmed before.
But while teaching and learning Java as a programming language, the scenario is a little bit different. We are going to explain it here.
Java was not designed to be used by novice programmers. It was designed to be used by experienced professional programmers who were experienced in coding in C. The creators of the language designed Java with a C/C++ style syntax that the system and application programmers at that time would find familiar. Hence it is more often than not expected that you have some prior basic knowledge of computer science and already know a little bit of programming.
We will not expect this. We will teach you the fundamentals first. In essence, we will teach you conventional structured programming first. Then we will build your foundation of Object Oriented Programming on top of your understanding of structured programming.
But in this section, we are going to deviate from our original lesson plan a little bit. As we have already written our first program in Java using OOP (though we have no idea what Object Oriented Programming or OOP is) we want to show you the anatomy of that program. While we are at it, we are also going to discuss how a Java Program is structured. Let us begin.
What Is The Code Structure In Java?
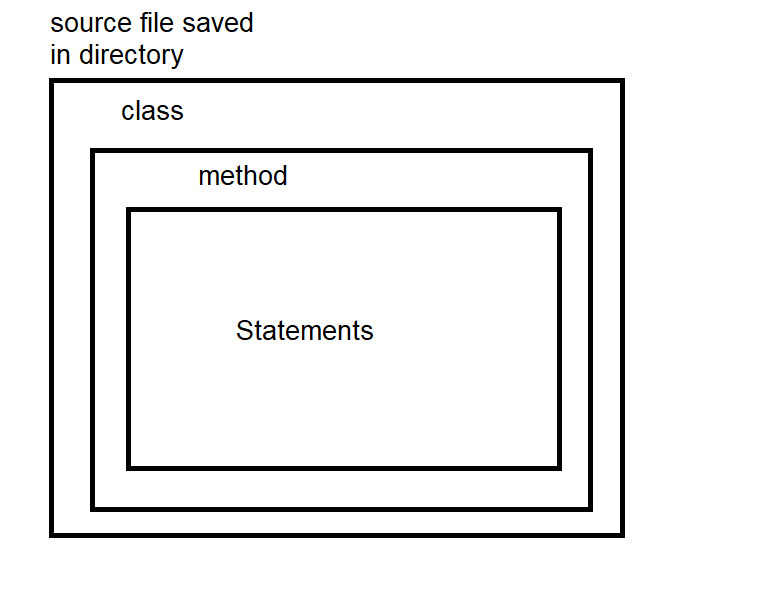
We put a class in a source file We put methods in a class We put statements in a method
If you know what class, method, and statement mean then it’s good. But we assume that you do not know about them. We are going to keep the juicier bit for later. We will be covering them in detail just a few articles later. For now, pay attention to the following.
Remember what We did when we created the HelloWorld java program and ran it?
First, we created a plain text file using Visual Studio Code, wrote some code in it, and saved it as a HelloWorld.java file on our machine.
This file where we wrote our source code and saved it in our machine is called a source file. Every and any file with a .java extension is a java source file because it contains the source code for our program, written in human-readable plain text format. We programmers write them. When we compile source files(in essence source code) using a compiler(in java it is javac), the compiler generates a binary file and automatically saves it in our machine.
Now we hope it’s clear to you what a source file is.
What Goes In A Source File?
Of course, our source code for a program goes in it. But there is more to it. We said over and over again that Java is an Object Oriented Programming language. We will cover class and object in detail later. Nevertheless, we will have a brief discussion here.
In Java Object means exactly the same thing as it means to us in the real world. Cars, computers, Mobile phones, etc are examples of objects. Take a car. It is an example of an object in the real world. What does a car have? It has different attributes associated with it like color, number of seats, etc. We can also call them their properties. Moreover, a car has some functionalities associated with it. You can drive a car, Accelerate a car, or brake and park a car. In Java terminology, these are methods of the car, tasks that a car can perform.
If we need to construct a car we need a blueprint. A blueprint defines its properties and functionalities and how to implement those functionalities. In Java terminology, this blueprint is called class.
Now, look at your HelloWorld program. The first line contains something like this
public class HelloWorld {
We told you that you must save the file as HelloWorld.java to be able to run the program.
A java source file(a file with a .java extension ) holds one(or more) class definitions. If we use the public keyword (Keywords or Reserved words are the words in a language that are used for some internal process or represent some predefined actions. These words are therefore not allowed to be used as variable names or objects. If we do, we will get a compile-time error. The code will not compile) at the beginning, like in our HelloWorld program, then we must save the file with the name that follows the class keyword with a .java extension. This is how we define classes. The class can either represent a whole program or a piece of your program depending on the size of your program. A very tiny application like our HelloWorld needs just a single class and hence a single source file. A real-world java application will need multiple source code files.
Look at our program and carefully observe that the whole class was within a pair of curly braces. The class body must go within a pair of curly braces.
What Goes Into A Class?
You must be wondering what goes on in a class. Methods go in a class. A class has one or more methods depending on the functionalities of the class.
In our HelloWorld program, there was only one task to do. Print Hello, world! on the console. So we only needed one method. It went in the main method. Your methods must be declared inside a class(in other words, within the curly braces of the class). This is the iron rule of java. You can do nothing about it but obey.
What Goes In A method?
Within the curly braces of a method, the instructions for how that method should be performed are defined. Think of it as a procedure you must complete before you can achieve your goal. Method code is basically a set of instructions.
There are a lot of curly braces, right? Do not worry! You will have plenty more of them. These curly braces and one (or several) missing semicolon(s) are most of the time the main culprits behind our source code not compiling. You will have to spend a millennium to find the bug and fix it.
You do not have to worry about memorizing anything right now. This article is just to get you excited and started.
Just remember till we discuss the method, object, and class that your code goes into the main() method.
public class YourClassName{//You Can give meaningful name
public static void main(String[] args) {//You can not change this line even a little bit
//ALL YOUR CODE OR INSTRUCTIONS WILL BE HERE TILL WE DISCUSS METHODS AND CLASS
}
}
Do not change anything that is inside a box. You may only change the class name.
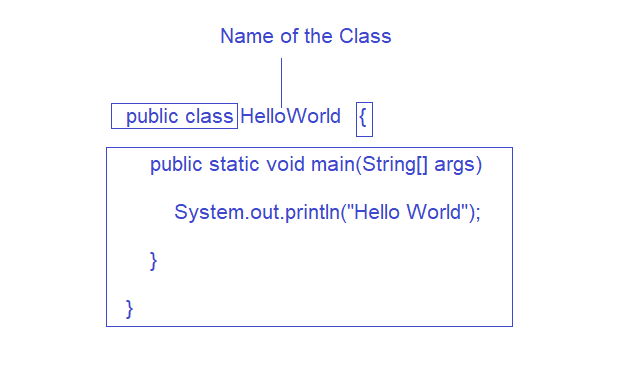