Object-oriented programming is a popular approach to software development used by many programming languages, including Java. In OOP, code is organized around objects, which are instances of classes that have properties and methods. Java is an ideal language for learning OOP due to its built-in support for the language constructs that are essential to OOP, such as inheritance, polymorphism, and encapsulation. With Java, developers can write code that is modular, reusable, and easy to maintain, making it a powerful tool for creating complex software systems.
In our previous tutorials, the main() method is where we placed all of our code. Not quite object-oriented, that. In fact, that isn’t even remotely object-oriented. Actually, we didn’t create any of our own object types; we only used a few existing ones. It’s time to say goodbye to traditional procedural programming for good. We need to leave the procedural world behind, get out of main(), and start making our own objects. We’ll investigate what makes object-oriented (OO) development in Java so enjoyable. We’ll examine the distinction between a class and an object. We’ll look at how objects can improve your life (at least the programming part of it).
The articles in this Java OOP series are listed below.
- Introduction To Object Oriented Programming In Java
- A Sneak Peek Of Objects in Java
- Class In Object-Oriented Programming
- A More In-Depth Inspection Of Class In Java
- FAQs About Classes In Java
- Class Fields
- Memory Management In Java
- Methods In Java
- Java OOP – Introduction To Arrays
- Constructors In Java
- Exception Handling In Java
- Java Application Programming Interfaces (APIs)
- Inheritance In Java
- Polymorphism In Java
What Is Object Oriented Programming?
Let us first take a brief history lesson.
According to Wikipedia
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of “objects” that can contain both data and code: data in the form of fields (also known as attributes or properties) and code in the form of procedures (often known as methods).
In other words, Object-oriented programming (OOP) is a type of computer programming (software design) in which programmers define not only the data type of a data structure but also the operations (functions) that can be performed on the data structure.
Are you confused right now? Do not worry. We have just started. It all will make sense to you in due time.
In simple terms, we would like to explain it with an example. First, ask yourself, “Why are we all programming?”. The answer to this question is to find practical solutions to real-life problems, that will save time and human effort. Assume that, as a worker, it is your responsibility to put cash to the ATM. Therefore, you must use logic to determine how many notes of 500, 2000, or 100 you should keep there in order to fully satisfy the expectations of the individual withdrawing money. This is how object-oriented programming is done.
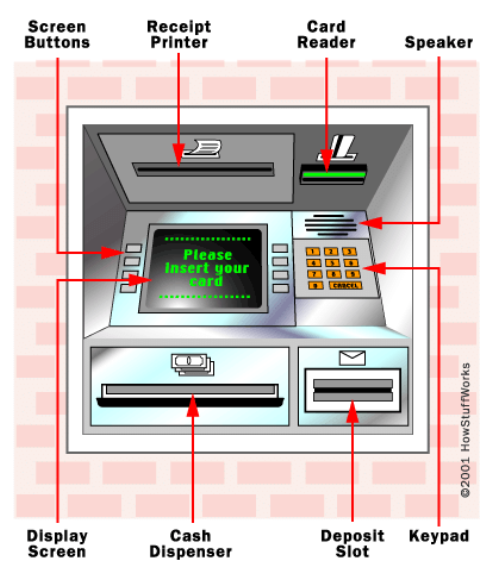
Understanding Objects and Object-Oriented Programming (OOP)
Consider yourself an object in this world. So you have some characteristics like height, weight, and so on, and you participate in real-world problem-solving. On OOP, an object is represented by its data, behavior, and associated functions.
The two fundamental terms in the OOP concept are CLASS and OBJECT. For example, the C language employs a procedural approach, which means that the program will be executed in the order specified by the programmer. However, using the Java language, you can divide the problem into class and object approaches and solve it using methods and other object-oriented programming features. Do not worry if does not make any sense to you right now. We will go through these in great detail in our later tutorials.
Objects all have procedures (or methods) attached to them that can access and modify the object’s data fields. The most popular OOP languages are class-based, which means that objects are instances of classes, which also determine their types.
One of the common misconceptions about object oriented programming is that it is all about syntax or the language you use. Object Oriented programming, on the other hand, is primarily concerned with a specific way of programming and organizing your code. However, many Object Oriented languages have been developed to facilitate this type of work.
It may be useful to compare OOP to other ways of thinking and coding. You can also program in procedural, functional, and data-oriented ways.
OOP is the process of “organizing your code around objects” and what you can do with these objects. Objects are simply data types with associated functions that perform operations on these data types. OOP languages typically allow inheritance and polymorphism to facilitate this type of thinking. That is, a data type can inherit properties from another data type as well as functions that operate on it.
In functional programming, on the other hand, you think in terms of function. You write functions that accept data as well as other functions as arguments and return functions or data. Data types, like in OOP, exist, but they are not the primary focus. As a result, you don’t have inheritance, where you can inherit properties from another data type. Functional programming is a subset of procedural programming. Data is typically immutable in functional programming, which distinguishes it from other procedural programming languages. You do not change the data you receive as input or return it.
Data-oriented programming entails designing programs to revolve around data flow. Consider it a pipeline or an electronic circuit. Data flows from one unit, is processed, and then flows to the next unit. You continue to work with data types and functions. It’s just that your perception of what those data types are differs. You don’t think of them as objects with behavior that mimics something in the real world. Instead, data is frequently only partially processed data that is sent to the next stage for further processing.
Abstractions in Object-Oriented Programming
All of this boils down to abstractions. You will realize it better when you create a larger program. Then you must consider how to organize everything so that you can make sense of it.
Here, in an excerpt from a 1994 Rolling Stone interview, Jobs explains what object-oriented programming is.
Jeff Goodell: Would you explain, in simple terms, exactly what object-oriented software is?
Steve Jobs: Objects are like people. They’re living, breathing things that have knowledge inside them about how to do things and have memory inside them so they can remember things. And rather than interacting with them at a very low level, you interact with them at a very high level of abstraction, like we’re doing right here.
Here’s an example: If I’m your laundry object, you can give me your dirty clothes and send me a message that says, “Can you get my clothes laundered, please.” I happen to know where the best laundry place in San Francisco is. And I speak English, and I have dollars in my pockets. So I go out and hail a taxicab and tell the driver to take me to this place in San Francisco. I go get your clothes laundered, I jump back in the cab, I get back here. I give you your clean clothes and say, “Here are your clean clothes.”
You have no idea how I did that. You have no knowledge of the laundry place. Maybe you speak French, and you can’t even hail a taxi. You can’t pay for one, you don’t have dollars in your pocket. Yet I knew how to do all of that. And you didn’t have to know any of it. All that complexity was hidden inside of me, and we were able to interact at a very high level of abstraction. That’s what objects are. They encapsulate complexity, and the interfaces to that complexity are high level.
Observing Real-World Objects for Object-Oriented Programming
Objects are essential for comprehending object-oriented technology. Look around you right now for examples of real-world objects: your computer, your desk, your mouse, and your bicycle.
Real-world objects all share two characteristics: state(properties) and behavior(functionalities). For Example, Computers have a state (name, color, ram size, and CPU) as well as behavior (processing, data fetching). Bicycles have state (current gear, pedal cadence, and speed) as well as behavior (changing gear, changing pedal cadence, applying brakes). Identifying the states and behaviors of real-world objects is an excellent way to get started with object-oriented programming.
Right now, take a moment to look around you at the things that exist in your immediate area. Consider two possibilities for each object you see:
what possible states it might be in and what potential behaviors it might perform.
Make sure to record your observations in writing. Your desktop lamp may just have two possible states (on and off) and two possible behaviors (turn on, turn off), whereas your desktop radio may have additional states (on, off, current volume, current station) and behaviors. As you proceed, you’ll see that real-world objects differ in complexity (turn on, turn off, increase volume, decrease volume, seek, scan, and tune). You might also observe that some items will contain yet other objects inside of them. All of these findings from the real world apply to object-oriented programming.
Real-world things and software objects share a conceptual similarity in that both are made up of state and associated behavior. An object exposes its action through methods while storing its state in fields (some computer languages refer to them as variables). The main method for object-to-object communication is a method, which operates on an object’s internal state. Data encapsulation is a key concept in object-oriented programming that involves hiding the internal state and requires all interaction to be carried out through an object’s methods. All these concepts will be discussed when the time comes.