Programming is a field where adaptability is essential. Programmers frequently struggle with the requirement to design functions that are flexible enough to accommodate a range of situations. To provide this flexibility in Python, overloading functions with optional parameters is a potent strategy. We’ll go further into the idea of function overloading, examine the subtleties of optional parameters, and present a ton of examples to help you grasp everything in this extensive book.
Function Overloading
The ability to specify numerous functions with the same name but distinct parameters or argument lists is known as function overloading. This gives programmers the ability to write more expressive and succinct code by offering many methods for calling a function depending on the inputs supplied. While function overloading is supported out of the box by more conventional languages like C++ and Java, Python has a more flexible strategy, depending instead on default parameter values and dynamic typing to accomplish comparable functionality.
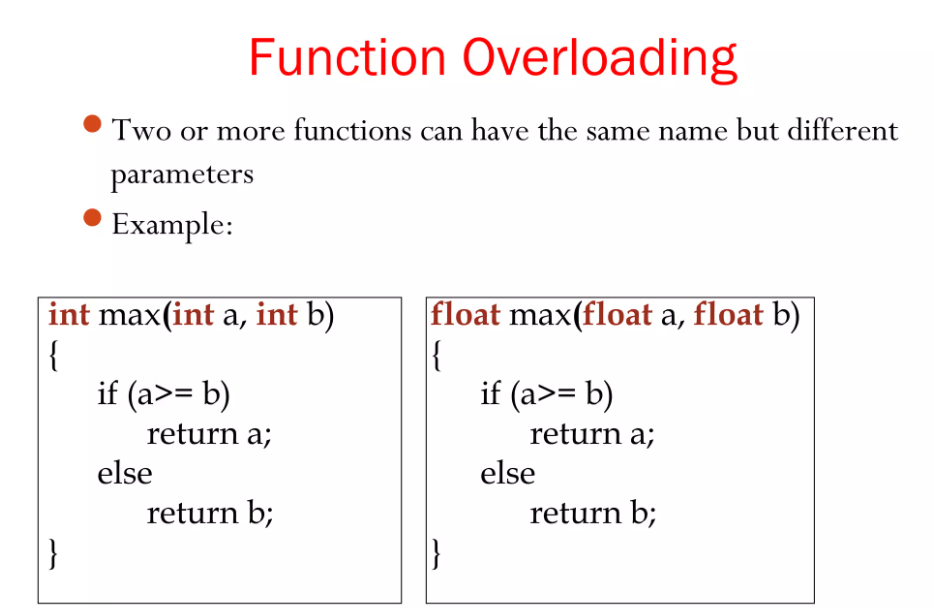
Understanding Optional Arguments
Functions that have default values assigned to them are called optional arguments, default arguments, or default parameter values. Default values are used when a function is called without a corresponding argument for a given parameter. This increases the flexibility of functions by enabling them to be called with different numbers of arguments, with default values being used for any unspecified parameters.
Function parameters in Python can be made optional by giving them a default value, as in def greet(name=’World’):. This makes it possible to invoke the function with fewer parameters than those for which it is designed. Here’s a simple example:
def greet(name='World'):
print(f'Hello, {name}!')
greet()
greet('Python')
Output:
‘Hello, World!’
‘Hello, Python!’
Defining Functions with Default Arguments
Python allows default values for function parameters. The parameter is assigned its default value if the function is called without an argument.
Python represents syntax and default values for function parameters distinctly. If no argument value is given during the function call, default values mean that the function argument will use that value. Using the assignment(=) operator with the format keywordname=value, the default value is assigned.
Let’s use a functioning student to better grasp this. Two of the three arguments in the function student have default values set to them. Hence, the function student allows two optional parameters in addition to the necessary one, the first name.
def student(firstname, lastname ='Mark', standard ='Fifth'):
print(firstname, lastname, 'studies in', standard, 'Standard')
# 1 positional argument
student('John')
# 3 positional arguments
student('John', 'Gates', 'Seventh')
# 2 positional arguments
student('John', 'Gates')
student('John', 'Seventh')
Overloading Functions with Variable-Length Argument Lists
Arguments that may also take an infinite amount of data as input are known as variable-length arguments or varargs for short. When using the data, the developer is not required to encapsulate them in a list or any other sequence.
There are two types of variable-length arguments in Python-
- Non – Keyworded Arguments denoted as (*args)
- Keyworded Arguments denoted as (**kwargs)
In Python, specific syntaxes called *args and **kwargs are used in function declarations to support varying quantities of parameters. While **kwargs enables you to send keyworded, variable-length arguments, *args is used to pass a non-keyworded, variable-length argument list. Let’s see how this functions in real life:
def func(*args, **kwargs):
for arg in args:
print(f'Non-keyworded argument: {arg}')
for key, value in kwargs.items():
print(f'Keyworded argument: {key} = {value}')
func('Hello', 'World', greeting='Hello', name='Python')
Output:
# Non-keyworded argument: Hello
# Non-keyworded argument: World
# Keyworded argument: greeting = Hello
# Keyworded argument: name = Python
Positional vs Keyword Arguments
Positional and keyword arguments differ primarily in how they are given to a function. Keyword arguments are passed by specifically identifying each argument and its value, whereas positional arguments are provided in the order in which they are declared.
def greet(greeting, name):
print(f'{greeting}, {name}!')
greet(name='Python', greeting='Hello')
Output:
‘Hello, Python!’
Understanding Default Values
A function parameter in Python can have a default value assigned to it. Because the function can be called without passing in a value for this argument, it becomes optional. The default value will then be used by the function.
def greet(greeting='Hello', name='World'):
print(f'{greeting}, {name}!')
greet()
greet(name='Python', greeting='Hi')
Output:
‘Hello, World!’
‘Hi, Python!’
Both the greeting and the name in this case have default values. When the greet() method is invoked without any parameters, the default values are used. As an alternative, we can supply our arguments to override the default settings.
Navigating Common Challenges with Python Optional Arguments
Python optional parameters have several possible drawbacks even though they can be quite useful. Let’s talk about some typical problems you can run across and how to solve them.
Incorrect Argument Order
Incorrect argument order is one prevalent problem. Positional arguments (including optional parameters) must always come before keyword arguments in Python. A SyntaxError will occur if this order is not followed:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet(name='Python', 'Hello')
Output:
# SyntaxError: positional argument follows keyword argument
The positional parameter “Hello” was put after the keyword argument name=”Python” in the example above, which is improper. Just make sure that all positional arguments come before keyword arguments to fix this:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet('Hello', name='Python')
Output:
# ‘Hello, Python!’
Missing Arguments
Missing arguments is another frequent problem. Python will throw a TypeError if you neglect to supply an argument that a function expects, whether it be positional or optional:
def greet(greeting, name='World'):
print(f'{greeting}, {name}!')
greet()
Output:
# TypeError: greet() missing 1 required positional argument: ‘greeting’
Advanced Techniques and Best Practices
It’s crucial to adhere to a few recommended practices when overloading methods in Python with optional parameters to guarantee readability, maintainability, and code compatibility:
- Document Your Functions: Clearly state the intent behind each function as well as any arguments it has, such as any default values or required input types.
- Use Meaningful Default Values: Select default values for arguments that make sense within the context of the function’s goal; do not set default values for changeable objects such as dictionaries or lists.
- Limit the Number of Optional Arguments: Although an excessive number of optional parameters might make a function harder to use and comprehend, they can also increase flexibility. In your function signatures, strive for clarity and simplicity.
- Test Your Functions Thoroughly: To ensure that your overloaded methods behave as intended under a variety of scenarios—such as edge cases and unexpected input—write thorough unit tests.
Common Pitfalls to Avoid
Although overloading methods with optional parameters can be an effective tactic, it’s important to be mindful of any potential risks and disadvantages:
- Ambiguous Function Signatures: To prevent confusion and unexpected behavior, do not define several functions with identical names and optional parameters that overlap.
- Complexity and Maintenance Overhead: A function’s complexity and maintenance overhead may rise if it has too many optional parameters. Proceed with caution while making such additions.
- Incompatibility with Type Annotations: Overloaded functions might be difficult to appropriately annotate since Python’s type hinting mechanism does not explicitly handle overloading functions with optional parameters.
We’ve covered everything from the fundamentals of function overloading to sophisticated strategies and best practices in this extensive tutorial to the idea of overloading functions in Python with optional parameters. You may build incredibly versatile and adaptable functions that satisfy the various requirements of your projects by utilizing keyword arguments, variable-length parameter lists, and default arguments.
To augment your comprehension of this subject matter, contemplate perusing supplementary materials like web-based guides, manuals, and discussion boards. Through persistent learning and experimentation with various methods, you may master overloading functions and open up new avenues for your Python programming endeavors.