Node.js Javascript Runtime
Node.js is a powerful JavaScript-based platform based on the JavaScript V8 Engine in Google Chrome. It’s used to create I/O-intensive web applications, including video streaming sites, single-page apps, online chat apps, and other web apps. It is widely used by both large, established corporations and emerging startups (Netflix, Paypal, NASA, and Walmart, to mention a few). Interested in learning more? This article will guide you through the process of getting started with Node.js.
Node.js is open-source and fully free, and it is used by thousands of developers worldwide. The platform has several advantages, making it a better alternative than other server-side platforms such as Java or PHP.
First, we’ll go over some of the fundamental principles needed to get started with Node.js, and then we’ll build our own Node.js app.
What is Node.js?
Node.js is a cross-platform, open-source JavaScript runtime environment and library that is used to run web applications outside of the client’s browser. It was created by Ryan Dahl in 2009, and the most recent version, 16.17.0 LTS, was published on 2022-08-16. Node.js is a server-side web application framework that is ideal for data-intensive applications due to its asynchronous, event-driven paradigm.
The Architecture of Node.js
To manage several concurrent clients, Node.js employs the “Single Threaded Event Loop” design. The processing paradigm of Node.js is based on the JavaScript event-based model and the JavaScript callback mechanism.
- Clients submit requests to the Web Server.
- Data searching
- Data deletion
- data updating, and so on.
- The requests are added to the event queue by Node.js.
- The Event Loop determines if the requests are basic enough that no external resources are required.
- EventLoop handles basic queries and provides replies to the appropriate customers.
- A single complicated request is allotted a single thread pool thread.
- Thread Pool completes the work and provides the result to the Event Loop, which then returns the result to the client.
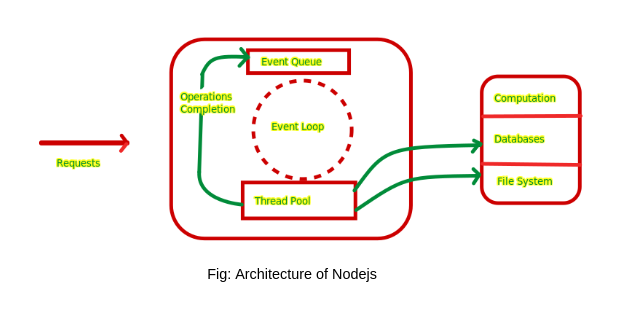
Package Manager for Node (npm)
The Node Package Manager has two key features:
- Online Node.js package/module repositories that are searchable using Node.js docs.
- A command-line program for installing Node.js packages, version management, and Node.js package dependency management.
NPM is installed together with Node.js. If NPM is properly installed, use the following command in CMD/Terminal: npm -v
tahuruzzoha@BattlePC:~$ node -v
v16.17.0
tahuruzzoha@BattlePC:~$ npm -v
8.15.0
Let’s look at different Node.js Modules now that we’ve covered what Node.js is, Node.js Architecture, and NPM as part of this Node.js tutorial.
Modules for Node.js
Modules are similar to JavaScript libraries in that they can be used to include a set of functions in a Node.js application. To include a module in a Node.js application, use the need() method with the module name enclosed in parenthesis.
Example:
let http = require('http');
let server = http.createServer((req, res)=>{
})
server.listen(5000)
Many modules in Node.js offer the fundamental functionality required for a web application. Some of them are listed in the table below:
Module | Descriptions |
http | Includes classes, methods, and events for building a Node.js http server. |
fs | This package contains events, classes, and methods for dealing with file I/O operations. |
util | Includes developer-friendly utility functions. |
url | Includes methods for URL parsing. |
stream | Includes methods to handle streaming data. |
quesrystring | Includes methods to work with the query strings. |
zlib | Includes methods to compress or decompress. |
HTTP module for Node.js
HTTP is a built-in module in Node.js. This module allows Node.js to send data across the internet. To include the HTTP module in an application, we utilize the need() function.
Now, using the HTTP module, let’s build a basic Web server: run in the terminal by executing the following command:
tahuruzzoha@BattlePC:~$ node filename.js
let http = require('http');
let server = http.createServer((req, res)=>{
res.write("Hello Enablegeek");
res.end();
})
server.listen(5000)
To include the HTTP module in the program, we use the require() function.
Then, we use the createServer function to create a server object. Following that, we write a response to the client, ‘Hello Enablegeek’, and then end it. We configured the web server to listen on port 5000.
Then it will start a server.
This is what the web browser output looks like when we go to the URL with the right port.
File System in Node.js
The node.js file system will be covered next in the getting started with node.js guide. The Node.js file system module allows a computer’s file system to function. To incorporate the file system module in the web application, we utilize the require() function.
Example:
let fs = require('fs');
Events in Node.js
Every action performed on a computer is referred to as an event. For instance, opening a file, connecting to the internet, and so on. Node.js includes an events module that allows users to create, trigger, and listen for events. Much of the Node.js core API is based on an idiomatic asynchronous event-driven architecture in which certain types of objects (called “emitters”) emit named events that call Function objects (“listeners”).
When a peer connects to a server object, it emits an event; an fs. When a file is opened, a ReadStream emits an event; a stream emits an event whenever data is ready to be read. The EventEmitter class is used by all objects that emit events. These objects expose an eventEmitter.on() function, which allows one or more functions to be attached to the object’s named events. Event names are typically camel-cased strings, but any valid JavaScript property key can be used.
When the EventEmitter object emits an event, all functions associated with that event are called synchronously. Any values returned by the called listeners are discarded. The following code demonstrates a primary EventEmitter instance with a single listener. The eventEmitter.on() method registers listeners, whereas the eventEmitter.emit() method triggers the event.
Consider the following basic Events module implementation in a Node.js application:
Example:
let events = require('events');
let eventEmitter = new events.EventEmitter();
let myEventHandle = () =>{
console.log("clicked");
}
eventEmitter.on('click', myEventHandle);
eventEmitter.emit('click');
Output:
clicked
Express Framework for Node.js
We’ll look at node.js next in the getting started with node.js tutorial. Express is a powerful Node.js web application framework that allows you to create both web and mobile applications. It is a layer built on top of Node.js that aids in server management and routing.
Let’s take a look at some of the Express framework’s key features:
- Designed for single-page, multi-page, and hybrid web applications.
- Allows developers to create middleware that responds to HTTP requests.
- A routing table is defined to perform various actions based on the HTTP method and URL.
- Allows for the dynamic rendering of HTML pages by passing arguments to templates.
- var express: Importing Express framework into our Node.js application
- app.get(): Callback function with the parameters: request, and response
- Request object: The HTTP request that contains properties for the request query string, parameters, body, HTTP headers, etc.
- Response object: The HTTP response object that an Express app sends when it gets an HTTP request
- The application will listen to the defined port, which in this case is “5000,” and variables “host” and “port” will contain the address and the port, respectively.
- console.log: This is used to show the address and port in the command prompt or terminal.
Node.js with Databases
Next in the getting started with node.js tutorial, we will look at how Node.js can be used with various databases to enable web applications to store data and perform operations with that data.
MySQL
MySQL is among the more popular databases that can be connected to Node.js. It is the most popular open-source relational SQL database management system. It also is one of the best RDBMS used to develop various web-based software applications.
You can install the MySQL module using the following command prompt, and then add it to your file:
const mysql = require(‘mysql’)
MongoDB
MongoDB is also a popular database choice to connect with Node.js. It is an open-source document database and a leading NoSQL database. This database is often used for high-volume data storage.
You can install the MongoDB module using the following command prompt and then add it to your file:
const mongodb = require(‘mongodb’)