Tutorial Category: Algorithm
An algorithm is a set of instructions or a step-by-step procedure for solving a problem or accomplishing a specific task. It is a well-defined computational procedure that takes some input, performs a series of operations, and produces an output.
Algorithms are used in a wide range of fields, including computer science, mathematics, engineering, and more. They are often used to solve complex problems that require a systematic approach and can be used to perform tasks such as sorting, searching, and data analysis.
A good algorithm is efficient, accurate, and easy to understand. It should be designed to produce the correct output for any valid input and should be able to handle errors or unexpected situations gracefully. Algorithmic efficiency is also an important consideration, as it can have a significant impact on the performance of a system or application.
Some common examples of algorithms include:
Sorting algorithms, such as bubble sort, merge sort, and quicksort, arrange a collection of data in a particular order
Search algorithms, such as linear search and binary search, find specific data within a collection
Encryption algorithms, such as AES and RSA, encode data to keep it secure
Optimization algorithms, such as gradient descent, find the best solution to a problem within a set of constraints
Algorithms are a fundamental concept in computer science and are used extensively in programming and software development.
A Brief Introduction to Algorithm
Bubble Sort Algorithm
Selection Sort Algorithm
Counting Sort Algorithm
Merge Sort Algorithm
Binary Search Algorithm
Ternary Search Algorithm
Breadth First Search Algorithm (BFS)
Depth First Search Algorithm (DFS)
Single Source Shortest Path in an Unweighted Graph
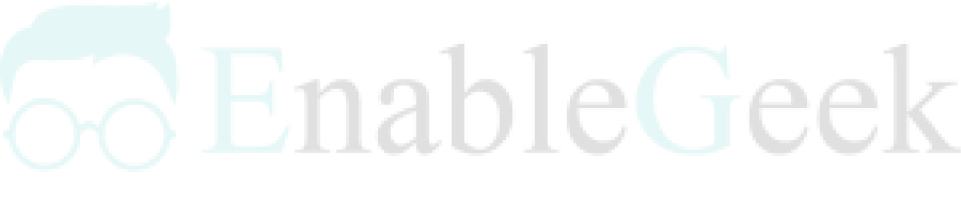
Check Our Ebook for This Online Course
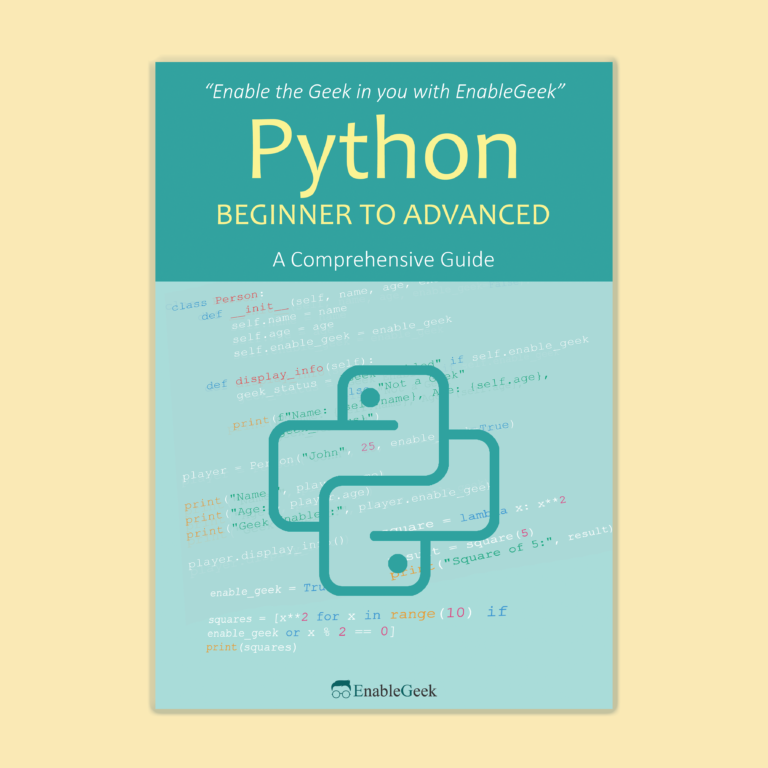
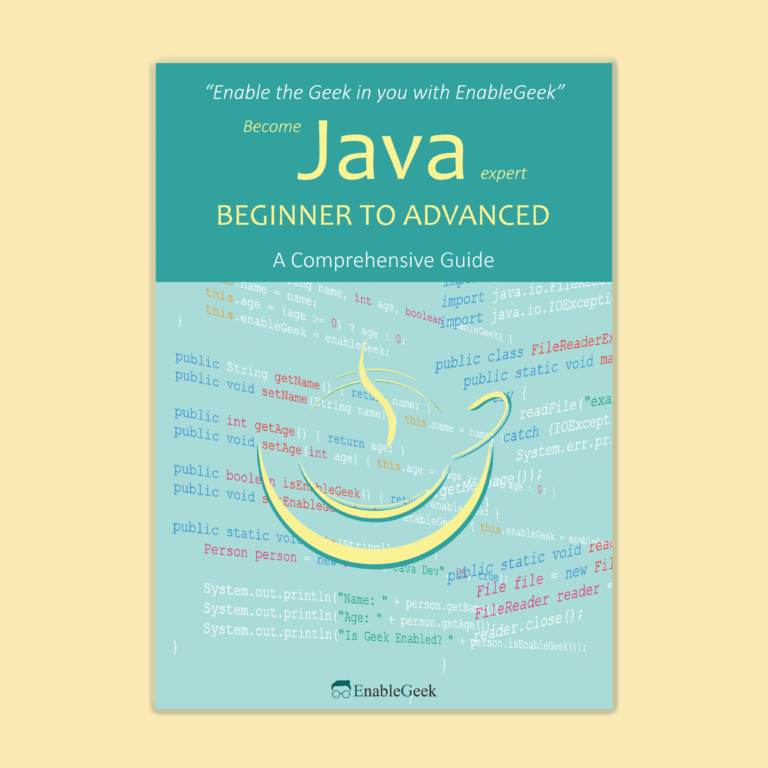
Advanced topics are covered in this ebook with many examples.