In the field of data analysis and manipulation, effective analysis and visualization depend heavily on data organization. For managing tabular data, Python’s Pandas library offers a useful feature called DataFrame. Nonetheless, there are times when you may need to reorder the columns in a data frame to optimize data display or analysis. This blog article will cover many ways and approaches for rearranging DataFrame columns in pandas, enabling you to organize your data according to your analytical goals and visualization needs. Pandas provide flexible options to optimize your data manipulation workflow, whether you’re rearranging columns for readability, consistency with other data sources, or certain analytical requirements.
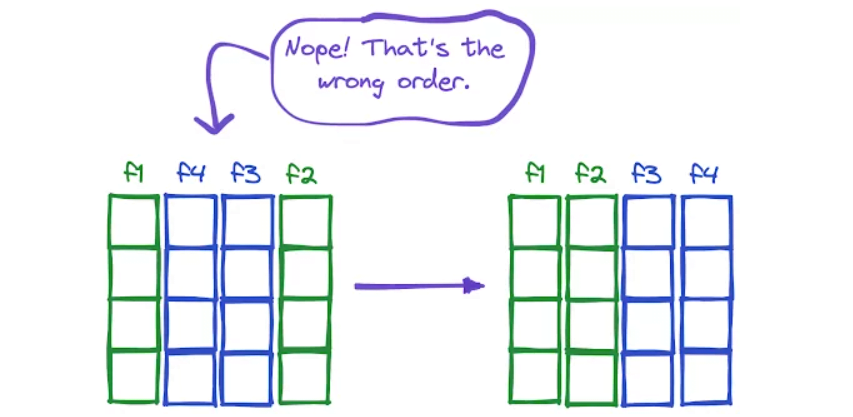
Create a DataFrame with a Dictionary of Lists
To demonstrate how to change the column order with examples, let’s now establish a DataFrame with a few rows and columns. Column titles in our DataFrame include Courses, Fee, Duration, and Discount.
# Create a DataFrame with a Dictionary of Lists
import pandas as pd
technologies = {
'Courses':["Java", "Python", "pandas", "Networking","Dart"],
'Fee' :[20000,25000,24000,21000,22000],
'Duration':['30days', '40days' ,'25days', '60days', '50days'],
'Discount':[1000,2300,1200,2100,2000]
}
df = pd.DataFrame(technologies)
print(df)
Output:
Courses Fee Duration Discount
0 Java 20000 30days 1000
1 Python 25000 40days 2300
2 pandas 24000 25days 1200
3 Networking 21000 60days 2100
4 Dart 22000 50days 2000
Why Change the Order of DataFrame Columns?
Let’s quickly go over the reasons why you would wish to alter the DataFrame columns’ order before getting into the methods:
- Data Presentation: Putting columns in a logical sequence might help with reading and comprehension when presenting data to stakeholders or visualizing it.
- Analysis Requirements: To facilitate data manipulation or calculation, certain analytical jobs may call for particular column ordering.
- Data Transformation: Aligning column ordering can make the process of executing operations like merging or concatenating DataFrames easier.
Now that we know how important column order is, let’s look at several methods for reordering the columns in a DataFrame.
Change the Order of Columns in Pandas DataFrame
The DataFrame columns can be rearranged in any way you like by passing a list of the columns to df[], such as df[[‘Discount’, “Courses”, “Fee”, “Duration”]].
# Using double brackets to change column
df = pd.DataFrame(technologies)
df2 = df[['Discount',"Courses","Fee","Duration"]]
print(df2)
Output:
Discount Courses Fee Duration
0 1000 Java 20000 30days
1 2300 Python 25000 40days
2 1200 pandas 24000 25days
3 2100 Networking 21000 60days
4 2000 Dart 22000 50days
Change Columns Order Using DataFrame.reindex()
As an alternative, you may use the DataFrame.reindex() function to alter the column order in a pandas DataFrame. To rearrange the columns, use df.reindex(columns=change_column) with a list of columns as change_column that are in the desired order.
# Using DataFrame.reindex() to change columns order
change_column = ['Courses','Duration','Fee','Discount']
df = df.reindex(columns=change_column)
print(df)
# You can also try
df = df.reindex(['Courses','Duration','Fee','Discount'], axis=1)
print(df)
Using the reindex() technique, this application will generate a DataFrame with the columns rearranged as per your requirements. The order you choose can be substituted for the change_column list.
Output:
Courses Duration Fee Discount
0 Java 30days 20000 1000
1 Python 40days 25000 2300
2 pandas 25days 24000 1200
3 Networking 60days 21000 2100
4 Dart 50days 22000 2000
Reorder DataFrame Columns in Sorted Order
Using df.columns, you may obtain the pandas DataFrame column names as a list. Then, you can sort the columns using the sorted() function and pass the sorted columns to DataFrame.The reindex() function returns a DataFrame with columns sorted in order.
# Change sorted order columns
df = df.reindex(sorted(df.columns), axis=1)
print(df)
# Reorder DataFrame column in sorted order
df = df.reindex(columns=sorted(df.columns))
print(df)
Output:
Courses Discount Duration Fee
0 Java 1000 30days 20000
1 Python 2300 40days 25000
2 pandas 1200 25days 24000
3 Networking 2100 60days 21000
4 Dart 2000 50days 22000
Using DataFrame Constructor
The columns in the current DataFrame can also be rearranged using pd.DataFrame(df,columns=[‘Courses’,’Discount’,’Duration’,’Fee’]). Make a new DataFrame column and take into consideration the current DataFrame, which is df.
# Using DataFrame constructor
df = pd.DataFrame(df, columns=['Courses','Discount','Duration','Fee'])
print(df)
Pandas Reorder the Columns
To change the order of the pandas DataFrame column, use df=df.columns.tolist() to reorder the list in whatever manner you like. Take the df2=df[-1:]+df[:-1] approach, for example.
df = df.columns.tolist()
# Rearrange the list any way you want
df2 = df[-1:] + df[:-1]
print(df2)
Output:
‘Discount’, ‘Courses’, ‘Fee’, ‘Duration’]
Frequently Asked Questions on Change the Order of DataFrame Columns
- How should columns be rearranged in a DataFrame?
The optimal approach is determined by particular needs and preferences. The reindex() function and direct indexing are two popular and effective methods for rearranging columns within a DataFrame. Select the approach that most closely matches your workflow and coding style.
- What happens to the data in my DataFrame if I change the order of the columns?
A change in the column order does not affect the data itself. It merely modifies the DataFrame’s internal presentation of the data. Regardless of the column order, the values in each row are still linked to the corresponding column labels.
- Is there a limit to the number of columns I can reorder in a DataFrame?
The amount of columns you can rearrange in a DataFrame has no intrinsic restriction. Depending on the needs you have for your data analysis, you can rearrange as many columns as necessary. When dealing with big datasets, however, bear in mind memory constraints and processing efficiency.
- Is it possible to rearrange columns according to particular standards, such data type or alphabetical order?
Columns can be rearranged according to particular standards. For example, to reorganize columns according to data type or alphabetically, you can use conditional expressions or sorting methods. But remember that this can call for extra processing procedures.
We’ve looked at many techniques in this blog article for rearranging DataFrame columns in Pandas. Whether you decide to use DataFrame indexing or reindex(), pandas provides a variety of flexible options to make data organization easy. You may make sure that your DataFrame columns are organized to maximize duties related to data analysis, visualization, and transformation by becoming proficient with these strategies. Have fun with coding!