A graphical interface is required when developing applications for other people to use. You want a graphical interface if you’re writing programs for yourself. Even if you believe that the rest of your natural life will be spent writing server-side code with a web page as the client user interface, you’ll need to write tools at some point, and you’ll want a graphical interface. We’ll spend two tutorials working on GUIs and learning key Java language features like Event Handling and Inner Classes along the way. In this tutorial, we’ll create a button on the screen that does something when you click it.
Learning is always more efficient when you can visualize the concept. In this tutorial, we will build a small GUI project. In this project, we will create a chat app for our small group of friends. We can both send messages and receive messages sent by others. The finished product will look like this.
But don’t be concerned. Everything will be explained as we go. We have shown you the project so that you understand what you are doing and why you are doing it. Let us now proceed.
Note: We still have a long way to go before we can call this a true chat app. In this tutorial, we will only show you the GUI portion of the chat app. In subsequent tutorials, we will turn this into a real chat app.
It all starts with a window!!!
A Frame is a type of top-level window that has a title and a border. A JFrame is the object that represents a window on the screen. It contains all of the interface elements such as buttons, checkboxes, text fields, and so on. It can have a real menu bar with actual menu items. And it has all the little windowing icons for minimizing, maximizing, and closing the window for whatever platform you’re using.
The JFrame appears differently depending on the platform. On Microsoft Windows 10, this is a JFrame:
Your first graphical user interface (GUI) is a button on a frame!!
import javax.swing.*;//Remember to import this swing package.
public class FrameDemo{
public static void main(String[] args) {
// make a frame
JFrame frame = new JFrame("THIS IS A FRAME");
// make a button
JButton button = new JButton("Button"); // You can pass the text you want on the button to the button constructor
frame.getContentPane().add(button); // add the button to the frame's content pane
frame.setSize(400,300); // give the frame a size, in pixels
/*Finally, make it visible!! If you forget this step,
you will not see anything when running this code */
frame.setVisible(true);
/*This line causes the program to quit as soon as the window
is closed (if you leave it out, it will just sit there on the screen forever). */
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Let’s run it and see what happens!!
Q: When you run Windows, will a button look like a Windows button?
If you so desire. You can select one of several “look and feels”—classes in the core library that control how the interface looks. In most cases, you have at least two options: the standard Java look and feel, also known as Metal, and the native look and feel for your platform.
Q: I heard Swing was painfully slow and that no one uses it.
This used to be true, but it is no longer. Swing may cause pain on less powerful machines. However, on newer desktops and with Java versions 1.3 and higher, you may not notice the difference between a Swing GUI and a native GUI. Swing is widely used in a variety of applications today.
Let us now return to our FrameDemo program. We added a button to the JFrame. But nothing appears to happen when we press/click the button. That is not entirely correct. When you press the button, it displays the ‘pressed’ or ‘pushed in’ look (which varies depending on the platform look and feel, but it always does something to indicate when it’s pressed).
“How do I get the button to do something specific when the user clicks it?” is the real question.
We need two things:
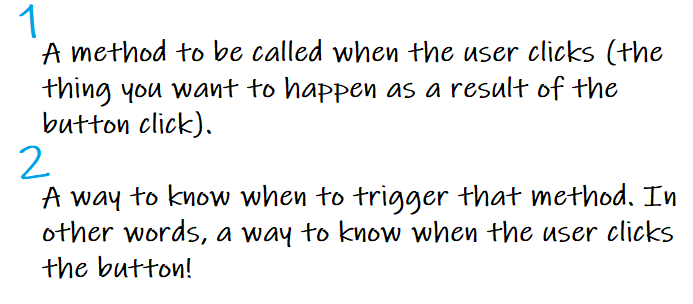
How To Get A User Event?
Assume you want the button’s text to change from “Button” to “I’ve been clicked” when the user presses the button. First, we can create a method that changes the text of the button (a quick search of the API will show you the method):
public void changeButtonText() {
button.setText("I’ve been clicked!");
}
But what now? How will we know when to execute this method? When the button is pressed, how will we know?
The process of getting and handling a user event is known as event-handling in Java. In Java, there are numerous event types, the majority of which involve GUI user actions. An event occurs when a user clicks a button. An event that indicates, "The user desires the action of this button to occur". So the most obvious event is when the user clicks the button, indicating they want an action to take place.
You usually don’t care about intermediate events like button-is-being-pressed and button-is-being-released when it comes to buttons. What you want to say to the button is, “I don’t care how long the user holds the mouse over it, how many times they change their mind and roll off before letting go, etc.”. Just let me know when the user is serious about doing business! To put it another way, don’t call me unless the user clicks in a way that indicates he wants the darn button to do what it says it’ll do!
What Exactly Is The Distinction Between Events, Event Handlers, And Event Listeners?
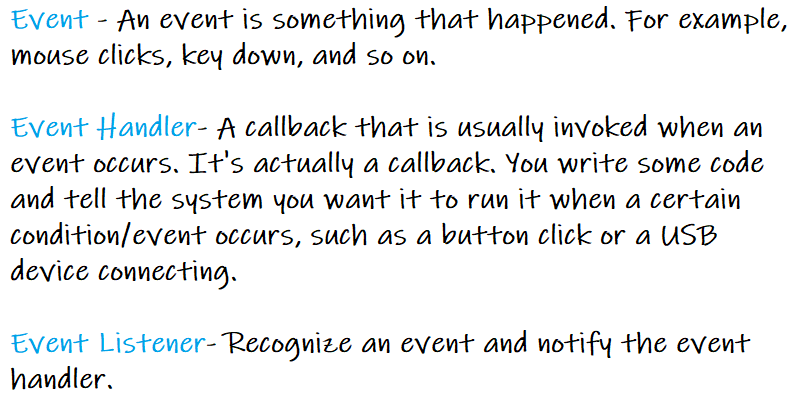
How would you communicate to a button object that you are interested in its events? That you’re an active listener?
If you care about the button’s events, implement an interface that says, "I’m listening for your events".
A listener interface is the bridge between the listener (you) and event source (the button).
The Swing GUI components are event sources. An event source is a Java object that can convert user actions (such as clicking the mouse, typing a key, or closing a window) into events. An event, like almost everything else in Java, is represented as an object. An instance of some event class. If you look through the java.awt.event package in the API, you’ll notice a slew of event classes (they all have Event in their names). MouseEvent, KeyEvent, WindowEvent, ActionEvent, and several others are available.
When a user does something significant (like click the button), an event source (such as a button) generates an event object. The majority of the code you write (and all of the code in this course) will respond to events rather than create them. In other words, rather than being an event source, you’ll spend the majority of your time as an event listener.
Each event type has a corresponding listener interface. Implement the MouseListener interface if you want MouseEvents. Want WindowEvents? Make use of WindowListener. You get the picture.
Remember your interface rules: to implement an interface, you must declare that you implement it , which means that you must write implementation methods for each method in the interface.
Because events come in a variety of flavors, some interfaces have multiple methods. If you implement MouseListener, you can receive events such as mousePressed, mouseReleased, mouseMoved, and so on. Despite the fact that they all take a MouseEvent, each of those mouse events has its own method in the interface. When the user presses the mouse, the mousePressed() method is called if you implement MouseListener. When the user releases the mouse, the mouseReleased() method is called. So, for mouse events, there is only one event object, MouseEvent, but several event methods that represent the various types of mouse events.
How Does The Listener And Source Communicate?
The Listener
You implement the ActionListener interface if your class wants to know about a button’s ActionEvents. Because the button needs to know you’re interested, you register with it by calling addActionListener(this) and passing an ActionListener reference to it (in this case, you pass “this”). When an event occurs, the button needs a way to call you back, so it calls the method in the listener interface. You must implement the interface’s sole method, actionPerformed(), as an ActionListener(). It is guaranteed by the compiler.
The Event Source
Because a button is a source of ActionEvents, it must know which objects are interested listeners. The button has an addActionListener() method that allows interested objects (listeners) to inform the button that they are interested.
When the button’s addActionListener() method is called (because a potential listener invoked it), it takes the parameter (a reference to the listener object) and stores it in a list. When the user clicks the button, it ‘fires’ the event by calling the actionPerformed() method on each listener in the list.
import javax.swing.*;//Remember to import this swing package.
import java.awt.event.*;//a new import statement for the package that ActionListener and ActionEvent are in.
/*Implement the ActionListener interface. This says, "an instance of FrameDemo IS-A ActionListener".
(The button will give events only to ActionListener implementers) */
public class FrameDemo implements ActionListener{
JButton button;
public static void main(String[] args) {
FrameDemo gui = new FrameDemo();
gui.drawGUI();
}
public void drawGUI(){
// make a frame
JFrame frame = new JFrame("THIS IS A FRAME");
// make a button
button = new JButton("Button"); // You can pass the text you want on the button to the button constructor
/*register your interest with the button. This says
to the button, "Add me to your list of listeners".
The argument you pass MUST be an object from a
class that implements ActionListener!! */
button.addActionListener(this);
frame.getContentPane().add(button); // add the button to the frame's content pane
frame.setSize(400,300); // give the frame a size, in pixels
/*Finally, make it visible!! If you forget this step,
you will not see anything when running this code */
frame.setVisible(true);
/*This line causes the program to quit as soon as the window
is closed (if you leave it out, it will just sit there on the screen forever). */
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
/* Implement the ActionListener interface's
actionPerformed() method.. This is the
actual event-handling method! */
/* The button calls this method to let you know an event
happened. It sends you an ActionEvent object as the
argument, but we don't need it. Knowing the event
happened is enough info for us. */
public void actionPerformed(ActionEvent event){
button.setText("I've been clicked!");
}
}
You will not be the source of events for the majority of your stellar Java career. Regardless of how much you imagine yourself to be the center of your social universe. Become accustomed to it. Your job is to be an attentive listener (Which, if done sincerely, can help your social life).
How To Get A Button’s ActionEvent?
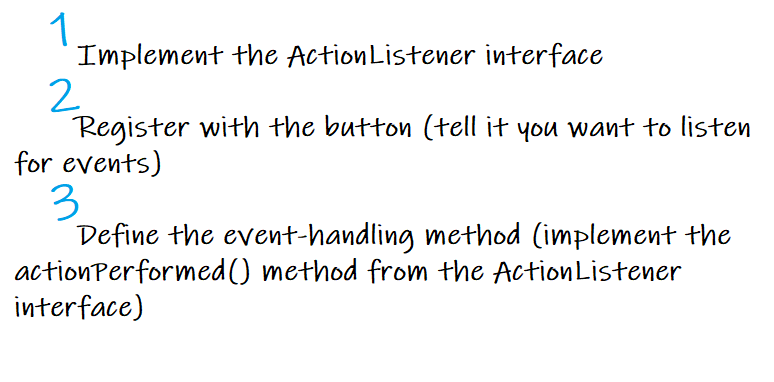