You have to download java on your machine to be enabled to compile and run Java Applications. You may be wondering what downloading java actually means. We will keep the juicier bits for later. As of now, downloading “Java” means downloading the Java Development Kit, also known as the JDK. Among the many tools that this “JDK” contains, there are tools that you will be used to compile and run a Java Application. The OpenJDK project and Oracle officially distribute the JDK. As its name suggests Java Development Kit is used to develop Java Applications.
There are some other elements you may have heard of, that are also called “Java”. One of them is JRE which stands for Java Runtime Environment. It is actually a subset of JDK. It is used to run an already compiled Java Application as it only contains the tools needed to run a Java Application. The java code can not be compiled by the tools provided in the JRE. OpenJDK or Oracle do not distribute this anymore.
There are other “Java”s called J2EE, Java EE, or Jakarta EE that you may also have heard about. All these acronyms refer to the Java Enterprise Edition. The tools and libraries (we will explain them later) provided by Java Enterprise Edition are used to create enterprise-class applications. This is different from the JDK and is outside the scope of this course.
How Do I Download And Run This JDK?
You can download the JDK from different places but there is a one-stop page that always refers to the latest version of JDK.
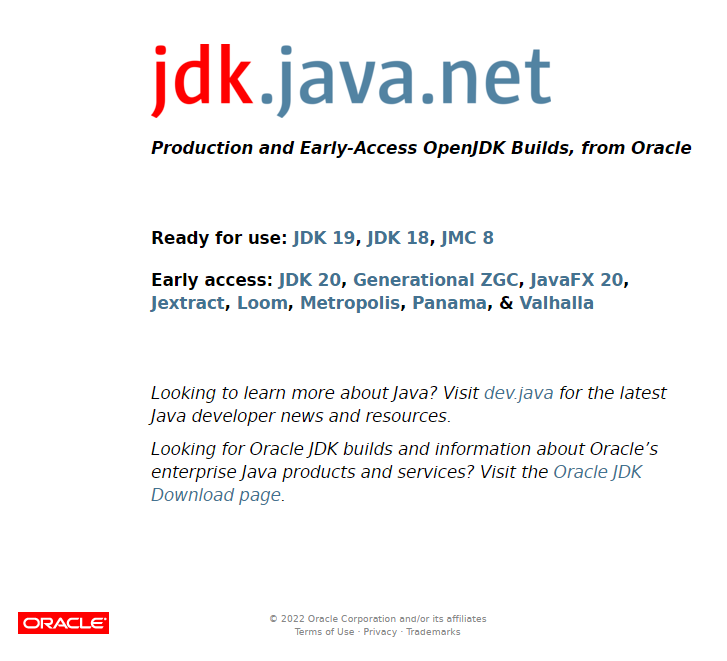
Now you will see an option "Ready for use: JDK{version}". Click here.
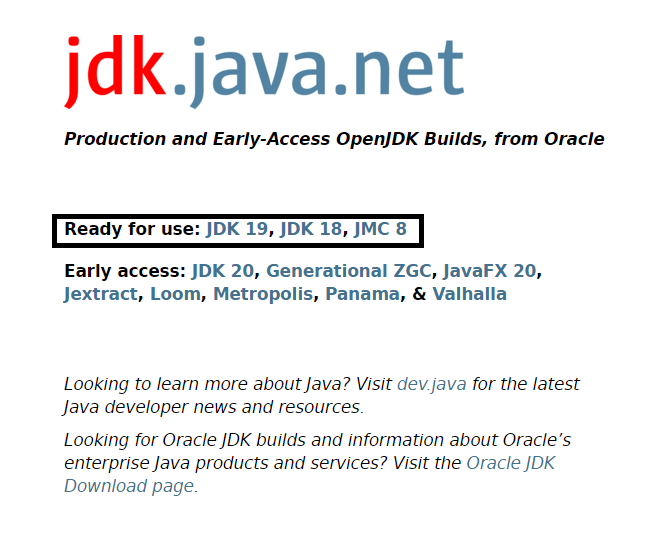
It will redirect you to the page where you can download the version of the JDK you need.
There are four versions in this page for you to download:
Linux/AArch64
Linux/x64
macOS/AArch64
macOS/x64
Windows/x64
This page provides production-ready open-source builds of the Java Development Kit, an implementation of the Java SE Platform under the GNU General Public License, version 2, with the Classpath Exception.
As we have already told you, we are using a windows machine to develop this course. so we will be downloading the Windows version. Click on zip (sha256).
You will be downloading a zip file of size about 180MB. Wait for the download to be completed. You can open this with any ZIP utility software. This zip file contains the JDK. You can unzip the contents of this file anywhere on your computer.
After extraction go to the extracted folder. It will be saved as "openjdk-{version}_windows-x64_bin" in the directory you choose.
Double click on it to access the file. There will be a folder named "jdk-{version}".
Open this folder. Find the bin folder.
Open the bin folder. There are two tools there : java and javac among hundreds of other tools.
We talked about these tools earlier. javac is needed to compile the Java Source Code and java is needed to run the Java Application.
Your computer needs to know about the existence of these two tools to use. You can do this by updating the PATH variable of your system to add the bin directory of your JDK directory to it.
You need to be very cautious while setting the PATH variable because a single mistake like an added white space or a missing semicolon will result in failure.
To check whether the path variable is successfully updated to add the bin directory of the JDK directory open the command prompt. Type java -version. If you have an output as the following the java is set in your machine to run Java Application.
java version "{version}"
Java(TM) SE Runtime Environment (build {version}})
Java HotSpot(TM) 64-Bit Server VM (build {version}, mixed mode)
If it doesn’t give an output like the above then follow the above process until you have an output like this.
To check if the compiler has been set up properly you need to type javac in the command prompt. If the output is as the following then you are all set and ready to compile the Java Source Code and Generate the byte code to run the Application.
If it doesn’t match the above then follow the previous steps again until you get this output.
One important thing to keep in mind:
You have to check if your java version and javac(compiler) version are the same or you may not run the Application. This happens due to version incompatibility.
If they match then alright. You are all set to compile and run the Java Application. Otherwise, follow the steps mentioned above again.
It is very unlikely to be a version mismatch of java and javac while you are directly downloading the JDK..
Once you have properly set your JDK and PATH variable, you are ready to compile your HelloWorld program.
How To Compile And Run A Java Program?
The remaining instructions are the same whether you are using Windows, Linux, or macOS.
1. Open the command prompt again.
2. Change to the directory where you have saved the HelloWorld.java.
3. You are all set to compile HelloWorld.java.
Type the following:
javac HelloWorld.java
At this point two things may happen. Your code may have some errors(in this case it won't happen) and due to this your compiler can not compile your code. The compiler will give you error messages. You need to fix the errors for being able to compile the source code and generate byte/executable code. Or your compiler remains silent and doesn't complain about anything.
Congratulations! You did it! You have generated the byte/executable code from the source code using the java compiler. Your java code has now been properly compiled. You can check this by checking in the directory where HelloWorld.java is saved. You will find a new file there in the directory: HelloWorld.class.
This is the compiled file containing the byte code/executable code. Make sure that the compiler created the HelloWorld.class for you.
To run the application all you have to do is type the following command in the opened command prompt:
java HelloWorld
This should print Hello, World! on the console.
If this is the case: Congratulations. You just run your first Java Application. If it didn’t check the source code and make sure you have typed everything accordingly.
The first Java Application that you wrote, compiled, and ran showed you the basic steps every Java Developer follows to run an application.
1.Create(Type) source code in a .java text file or in a set of .java text files for creating large,complex Java Applications.
2.Compile this(these) file to produce a(or a set of) corresponding .class file(s) called binary file(s) containing byte code/executable code that machine understands and can execute(We will talk about it in detail later. There are some gotchas here in Java).
3. Run it(or them together) as an application.
Why Aren’t We Using IDEs?
You may or may not have heard about Integrated Development Environments or IDE. Developers that work on large applications use them instead of plain text editors to manage their source code. IDEs are complex software applications. They specialize in software development. These applications handle the compilation of your source code automatically, they can help you to track errors in the syntax of your Java code and nail down bugs in its execution, among other things.
At the beginner level or at least until the end of this course we strongly advise you to not use them. Use plain Text Editor, Compile using javac in the command prompt, and run them as a console application. IDE can protect you from many small details that go under the hood to create a Java Application. We don’t want that. We want you to get bruised. This way you can become a seasoned Java Developer. Knowing all the little details will help you in the long run.