What Is Computer Networking?
It is the infrastructure that allows multiple end devices (such as computers) to communicate in the same logical environment. It is also necessary to understand how to manage this infrastructure in order to control how and in what manner the end devices communicate with one another.
Java was the first programming language created specifically for network applications. Java was originally designed for proprietary cable television networks rather than the Internet, but it has always prioritized the network. A web browser was one of the first two real Java applications. As the Internet expands, Java is uniquely suited to developing the next generation of network applications.
One of Java’s best kept secrets is that it makes writing network programs simple. In fact, writing network programs in Java is far easier than in almost any other language.
This tutorial will walk you through some complete programs that make use of the Internet. One thing you’ll notice is how little code is devoted to networking in fully functional applications. Almost all of the code in network-intensive programs, such as web servers and clients, handles data manipulation or the user interface. The network section of the program is almost always the shortest and simplest. In summary, Java applications can easily send and receive data over the Internet.
Before we proceed, let us define some terms related to computer networking.
Network
A network is a collection of computers and other devices that can send and receive data in real-time from one another. A network is frequently linked by wires, and bits of data are converted into electromagnetic waves that travel through the wires. Wireless networks, on the other hand, use radio waves to transmit data, and most long-distance transmissions are now carried over fiber-optic cables, which send light waves through glass filaments. There is nothing sacred about any physical medium for data transmission. In theory, data could be transmitted by coal-powered computers that communicate via smoke signals. Such a network’s response time (and environmental impact) would be quite poor.
Node
Each machine on a network is referred to as a node. Printers, routers, bridges, gateways, dumb terminals, and Coca-Cola, ATM machines are examples of nodes. You might use Java to interact with a Coke machine, but you’ll mainly communicate with other computers.
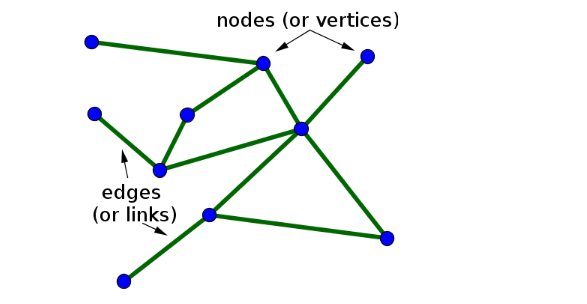
Host
Hosts are nodes that are fully functional computers. We will use the term node to refer to any network device, and host to refer to a node that is a general-purpose computer.
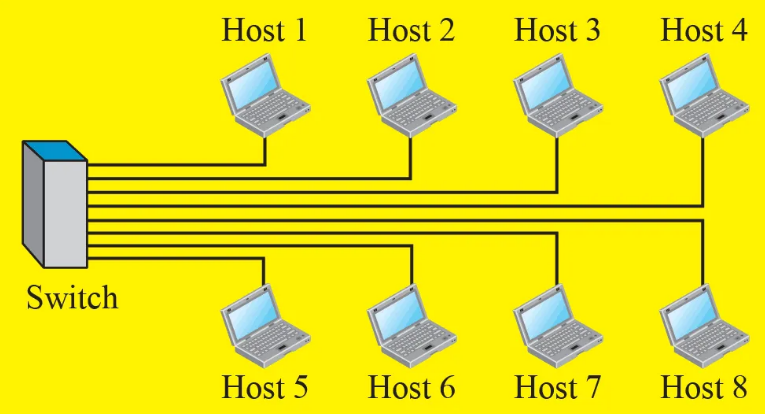
Address
Every network node has an address, which is a sequence of bytes that identifies it uniquely. This group of bytes can be thought of as a number, but the number of bytes in an address, or the ordering of those bytes (big endian or little endian), is not guaranteed to match any primitive numeric data type in Java. The more bytes in each address, the more addresses are available, and the more devices can be connected to the network at the same time.
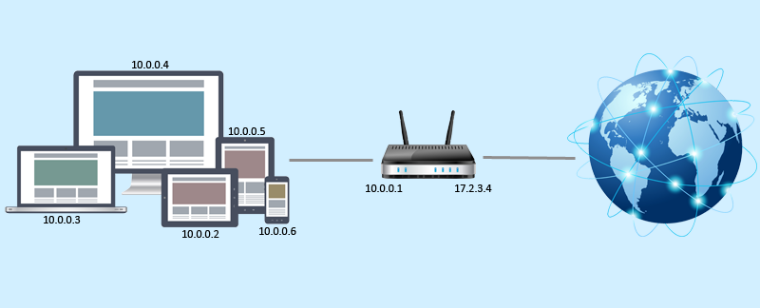
Protocol
A protocol is a detailed set of rules that define how computers communicate, such as the format of addresses and how data is divided into packets. There are numerous protocols that define various aspects of network communication.
For example, the Hypertext Transfer Protocol (HTTP) specifies how web browsers and servers communicate. The IEEE 802.3 standard defines a protocol for encoding bits as electrical signals on a specific type of wire.
Protocol standards that are open and published allow software and equipment from various vendors to work together. A web server doesn’t care if the client is a Unix workstation, an Android phone, or an iPad because all clients, regardless of platform, use the same HTTP protocol.
HTTP Protocol
HTTP is an abbreviation for Hyper Text Transfer Protocol. Let’s break it down to understand what this is. “Hypertext” is the text displayed on a computer screen or other electronic device that contains references to other text that the reader can access immediately. Hypertext documents are linked together by hyperlinks, which are typically activated by a mouse click, keypress set, or screen touch. A series of requests and responses are used to carry out the “Transfer Protocol”. When you click on a link, the client computer (your computer) sends a request to the computer (server) that is serving that link for the information represented by the link.
The server receives the request, also known as an HTTP Request, and processes it to retrieve the information requested by the client’s computer. Once the information has been retrieved, the server will respond (HTTP Response) by transferring it back. The information is then received by the client, who displays it on your screen.
TCP/IP Protocol
TCP/IP stands for Transmission Control Protocol/Internet Protocol. TCP/IP is a networking protocol that enables two computers to communicate with one another.
The Internet Protocol (IP) is the Internet’s address system, and its primary function is to deliver information packets from a source device to a target device. IP is the primary method of connecting networks and serves as the foundation of the Internet. The Internet Protocol (IP) does not handle packet ordering or error checking. Such functionality necessitates the use of another protocol, most commonly TCP.
TCP/IP is analogous to sending someone a message written on a puzzle through the mail. The message is written down, and the puzzle is disassembled. Each piece can then take a different postal route, some of which are faster than others. When the puzzle pieces arrive after traveling through their various paths, they may be out of order. The Internet Protocol ensures that the pieces arrive at their intended location. The TCP protocol can be thought of as the puzzle assembler on the other side who arranges the pieces in the correct order, requests missing pieces to be resentful, and informs the sender that the puzzle has been received. TCP keeps the connection with the sender open from the time the first puzzle piece is sent until the last piece is sent.
IP Address
An IP address is an abbreviation for “Internet Protocol address”. Assume your friend wishes to meet you but does not know where you live. He inquires about your address, and you respond with something like 221 B, Baker Street, London, United Kingdom. He can easily locate you after you give him this address. This residing address varies from person to person.
In the case of the internet, everyone is assigned an address. However, because the internet is used by everyone, different addresses of the same format are assigned to each computer, mobile phone, and other device that connect to the internet. This is referred to as an “Internet Protocol address”.
When you make a specific request on the internet, the request is entertained for the IP address assigned to you, and you can see the requested page on your screen.
IP addresses are of 2 types:
IPv4 IPv6
IPv4 is an abbreviation for Internet Protocol version 4. It employs a 32-bit addressing scheme. It has four sections that can hold values ranging from 0 to 255. It somewhat looks like 68.87.156.28. It can generate approximately 4.2 billion unique addresses before repeating.
IPv6 is an abbreviation for Internet Protocol version 6. It employs a 128-bit addressing scheme. It is designed to work around the limitations of IPv4. IPv6 has the ability to generate a large number of unique IP addresses.
Packet
In a computer network, a packet is a smaller portion of a larger message. A message is not transmitted all at once in computer networks; rather, it is divided into smaller pieces that can be transmitted much more easily. Consider the following real-world scenario: a person wishes to transport construction materials for his custom house in his pickup truck. That person isn’t going to load all of the construction materials into his pickup truck at once—his truck isn’t big enough for that. Instead, he divides his list of construction materials into smaller sections so that he can go to the hardware store multiple times and pick up the smaller section.
What Is The Difference Between TCP, IP, And HTTP?
TCP is a transport-layer protocol, and HTTP is an application-layer protocol that runs on top of TCP.
To understand the distinction (and many other networking topics), you must first grasp the concept of a layered networking model. Essentially, there are various protocols that allow a computer to communicate over various distances and layers of abstraction.
The physical layer is at the very bottom of the network stack. This is where electrical signals, light pulses, or radio waves transmit data from one location to another. The physical layer lacks protocols in favor of standards for voltages, frequencies, and other physical properties. You can send data directly this way, but you’ll need a lot of power or a dedicated line, and you won’t be able to share bandwidth without higher layers.
The link layer is the layer above the physical layer. This layer addresses communication between devices that use the same physical communications medium. Protocols such as Ethernet, 802.11a/b/g/n, and Token Ring define how to handle multiple concurrent physical medium accesses and how to route traffic to one device rather than another. In a typical home network, this is how your computer communicates with your “router”.
The network layer is the third layer. In most cases, this is dominated by Internet Protocol (IP). This is where the Internet’s magic happens, and you can talk to a computer halfway around the world without knowing where it is. Routers direct traffic from your local network to the network where the other computer resides, where its own link layer routes packets to the correct computer.
We’re finally getting somewhere. We can communicate with a computer somewhere in the world. But that computer is running a variety of programs. How should it know who to send your message to? This is handled by the transport layer, which is typically represented by port numbers. TCP and UDP are the two most widely used transport layer protocols. TCP does a variety of interesting things to smooth out the kinks in network-layer packet-switched communication, such as reordering packets and retransmitting lost packets. UDP is less dependable but has less overhead.
So we’ve linked your browser to the web server software on the other end, but how does the server know which page you’re looking for? How do you ask or answer a question? These are the responsibilities of application-layer protocols. This is the HyperText Transfer Protocol, which is used for web traffic (HTTP). There are thousands of application-layer protocols, such as SMTP, IMAP, and POP3 for email, XMPP, IRC, and ICQ for chat, Telnet, SSH, and RDP for remote administration, and so on.
These are the TCP/IP networking model’s five layers, but they are only conceptual. The OSI model consists of seven layers. In reality, some protocols shim between layers or can work at multiple layers at the same time. TLS/SSL, for example, encrypts and transmits session information between the network and transport layers. Application Programming Interfaces (APIs) govern communication with web applications such as Quora, Twitter, and Facebook above the application layer.
The OSI model is overkill for Java network programs. The primary distinction between the OSI model and the TCP/IP model used in this book is that the OSI model divides the host-to-network layer into data link and physical layers, while the TCP/IP model inserts presentation and session layers between the application and transport layers. The OSI model is more general and better suited for non-TCP/IP networks, though it is still overly complex most of the time. In any case, Java’s network classes only work on TCP/IP networks and always in the application or transport layers, so using the more complicated OSI model adds no value to this tutorial.
Your Java program can communicate with a program on another machine. It’s simple. Classes in the java.net library handle all of the low-level networking details. One of the most significant advantages of Java is that sending and receiving data over a network is simply I/O with a slightly different connection stream at the end of the chain. You can read it if you have a BufferedReader. And it makes no difference whether the data came from a file or flew down an ethernet cable to the BufferedReader. In this tutorial, we’ll use sockets to connect to the outside world. We will create client sockets. We’ll construct server sockets. Clients and servers will be created. And we’ll force them to communicate with one another. You’ll have finished the tutorial before you know it.
Let us first create a simple network program before delving into something more complex. Assume you’ve been tasked with creating a Time server. A Time Server is a computer server that reads the current time from a reference clock and distributes it to its clients via a computer network. The time server could be a local network or an Internet time server. In our program we will take our local computer as the reference clock. We will use our local computer as the reference clock in our program. Don’t be concerned about the terms “server” and “client.” We’ll go over them later in this tutorial.
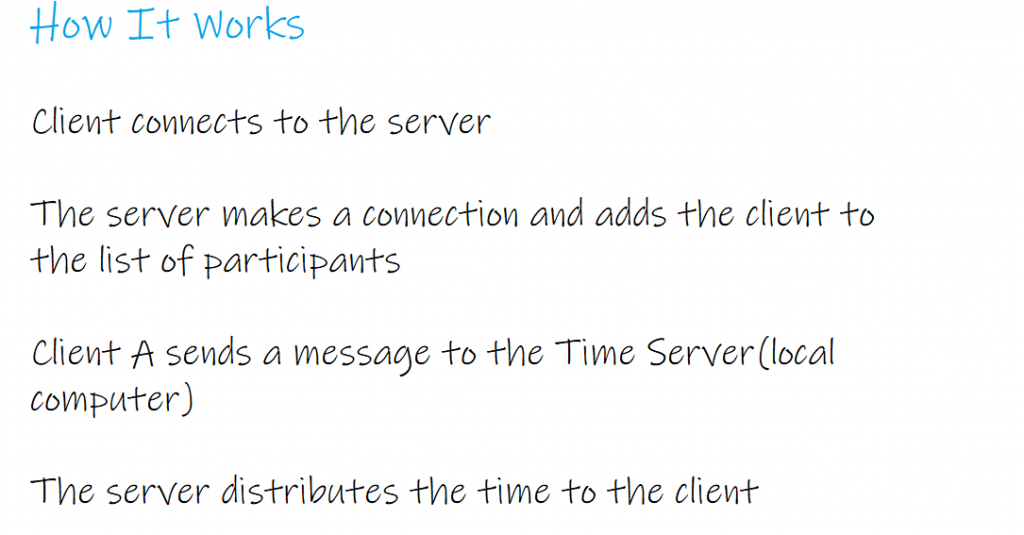
Connecting, Sending, and Receiving
The three things we must learn in order to get the client to work are:
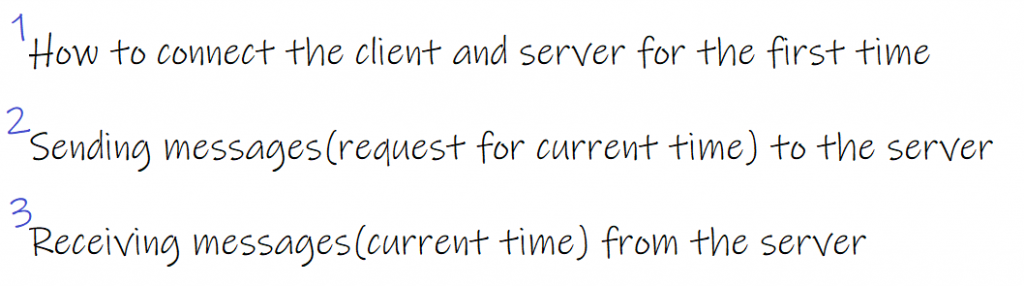
For these things to work, a lot of low-level stuff has to happen. But we’re fortunate because the Java API networking package (java.net) makes it simple for programmers. There will be far more GUI code than networking and I/O code.
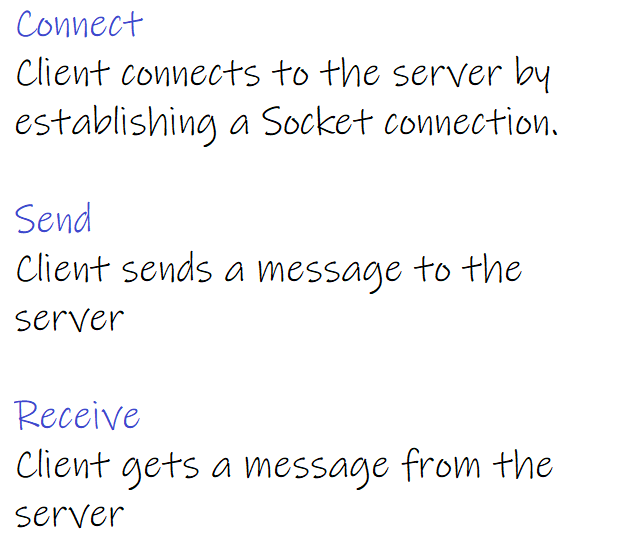
How To Make A Network Socket Connection
A Socket connection is required to connect to another machine.
A Socket (java.net.Socket class) is a network connection object that represents a connection between two machines. What is a connection? A relationship between two machines in which two pieces of software are aware of one another. Most importantly, those two pieces of software can communicate with one another. In other words, how to send bits to each other.
Thankfully, we don’t care about the low-level details because they are handled much lower in the ‘networking stack.’ Don’t be concerned if you’re unfamiliar with the term “networking stack”. It’s simply a way of looking at the layers that information (bits) must pass through to get from a Java program running in a JVM on some OS, to physical hardware (such as Ethernet cables), and back to another machine. Someone has to handle all of the dirty details.
Except for you. That someone is a combination of operating system software and the Java networking API. The part you need to be concerned about is high-level—very high-level—and shockingly simple. Ready?
In simple words, A Socket connection means the two machines have information about each other, including network location (IP address) and TCP port(program).
To establish a Socket connection, you must know two things about the server: who it is and which port it is listening on.
To put it another way, IP address and TCP port number.
TCP ports are simply numbers.
A 16-bit number that identifies a specific server program.
Your IP address is your house number. You can get to the house just by knowing the house number. Suppose each house has ten members. One of the residents has placed a pizza order. Who should get the package? The port identifies the receiver. The pizza should be delivered to the person whose name appears on it.
The idea behind using port numbers is that, with so many applications running on your system, which one should receive the packet? It is determined by the port. So, your IP address is your system’s identifier, and the port number is the identifier of the application running on that system.
Understanding ports is essential for network programming. Let us use another example.
Assume your pal Mia has invited you to her home. She gave you her house address. You arrived at her house on time. But that is not all you need to get into her house, is it?
To enter the house, you’ll also need doors or maybe a window. That’s what ports are. Ports are what they are. In most cases, doors are installed on one of the vertical walls of a house. So you go around the house, looking for such a door and attempting to enter. These are the well-defined ports with fixed port numbers, and any basic port scan will scan them.
But what if she has a secret underground entrance? Or, perhaps she has a door on the horizontal wall (roof).
These are the other port numbers. So your machine will recognize the well-defined port numbers, but if you want to connect to a server, say foo, but foo has moved its http server from port 80 to port 34251, you will be unable to find it because your browser will attempt to connect to port 80 and find it closed by default. We hope this was helpful.
Port 80 is used by your internet web (HTTP) server. That is the norm. If you have a Telnet server, it is “listening” on port 23. FTP? 20. 110. POP3 mail server SMTP? 25. The Time server is currently at 37. Consider port numbers to be unique identifiers.
They represent a logical link to a piece of software running on the server. That’s all. You won’t be able to turn your hardware box around and find a TCP port. For starters, there are 65536 of them on a server (0 – 65535). As a result, they clearly do not represent a location to plug in physical devices. They are simply a number that represents an application.
The server would have no way of knowing which application a client wanted to connect to if port numbers were not used. And since each application might have its own unique protocol, think of the trouble you’d have without these identifiers. What if, for example, your web browser landed on the POP3 mail server rather than the HTTP server? The mail server will be unable to parse an HTTP request! Even if it did, the POP3 server has no idea how to handle the HTTP request.
When you write a server program, you’ll include code that tells it which port number to use (you’ll see how to do this in Java later in this tutorial).
We chose 5000 in the TimeServer program we’re writing in this tutorial. We only did it because we wanted to. And it met the criteria of being a number between 1024 and 65535. Why 1024? Because the numbers 0 through 1023 are reserved for well-known services such as the ones we just discussed.
TCP port numbers 0 through 1023 are reserved for well-known services. Do not use them for your own server programs!
Port 5000 is used by the time server we’re creating. We simply choose a number between 1024 and 65535.
Also, if you’re writing services (server programs) to run on a company network, check with the system administrators to see which ports are already in use. Your system administrators may tell you, for example, that you cannot use any port number lower than 3000. In any case, if you value your limbs, you will not arbitrarily assign port numbers. Unless it’s your personal network. In that case, you simply need to check with your pets.
Q: How do you know the port number of the server program you want to talk to?
That is dependent on whether the program is a well-known service. If you’re trying to connect to a well-known service, such as those listed above (HTTP, SMTP, FTP, and so on), you can look it up on the internet (Google “Well-Known TCP Port”). Alternatively, contact your friendly neighborhood system administrator.
However, if the program is not one of the well-known services, you must inquire with whoever is deploying the service. Inquire with him. Or her. If someone creates a network service and wants others to create clients for it, they will typically publish the service’s IP address, port number, and protocol.
For example, if you want to write a client for a GO game server, you can go to one of the GO server sites and learn how to write a client for that specific server.
Q: Is it possible to run more than one program on a single port? To put it another way, can two applications on the same server use the same port number?
No! A BindException will be thrown if you attempt to bind a program to a port that is already in use. Binding a program to a port is as simple as starting a server application and instructing it to run on a specific port. Again, we’ll go over this in greater detail when we get to the server section of this course.
How To Communicate Over Socket Connection?
You now have a Socket connection. Both the client and the server are aware of each other’s IP address and TCP port number. What happens next? How do you communicate over that connection? In other words, how do you get bits from one place to another? Consider the types of messages your time client will need to send and receive.

Streams are used to communicate over a Socket connection.
Regular old I/O streams, just like in the previous tutorial. One of Java’s coolest features is that most of your I/O work doesn’t care what your high-level chain stream is connected to. In other words, you can use a BufferedReader in the same way you would when writing to a file, with the exception that the underlying connection stream is connected to a Socket rather than a File!
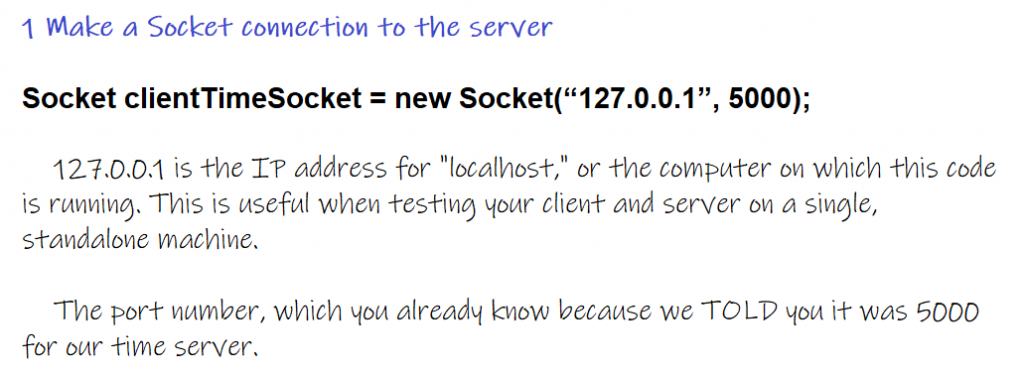
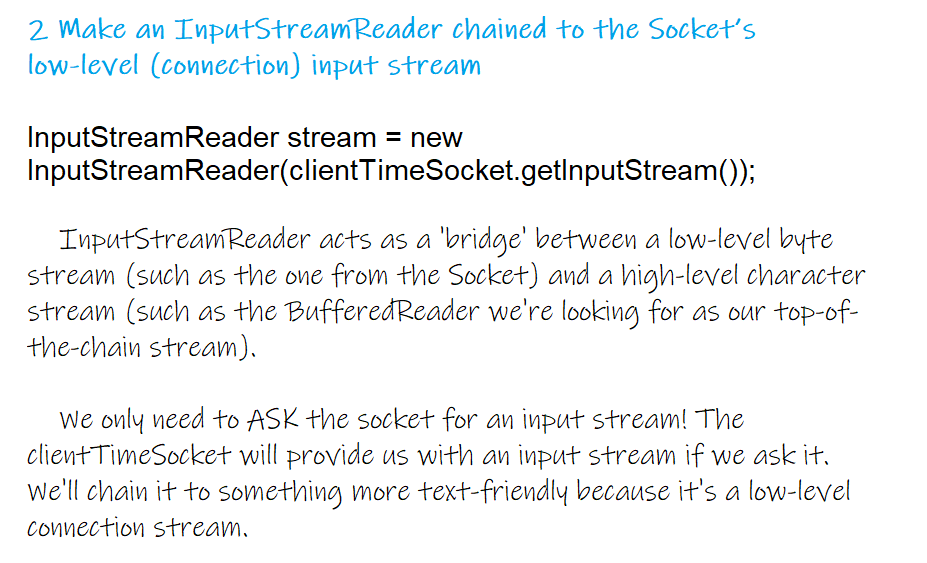
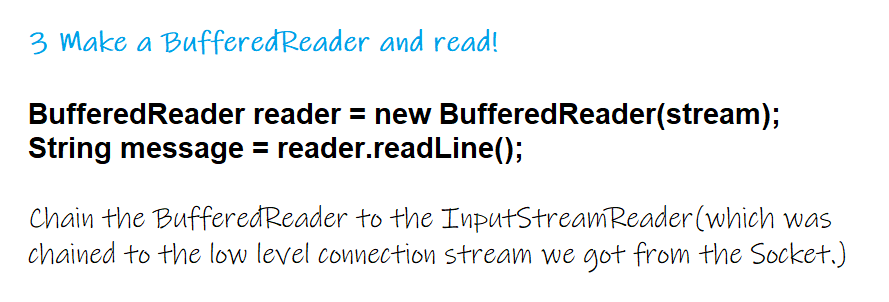

In the previous tutorial, we did not use PrintWriter, but rather BufferedWriter. We have a choice here, but when writing one String at a time, PrintWriter is the default. You’ll also recognize PrintWriter‘s two key methods, print() and println()! Just like the old System.out.
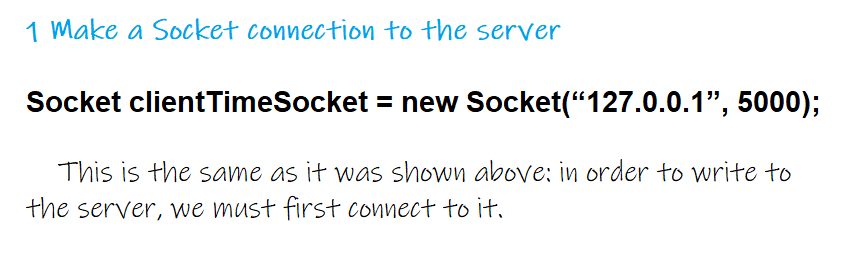
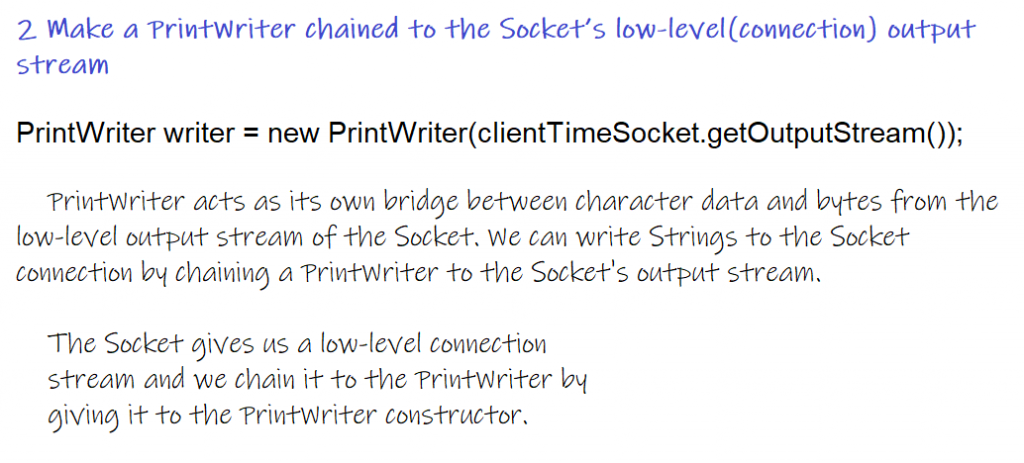
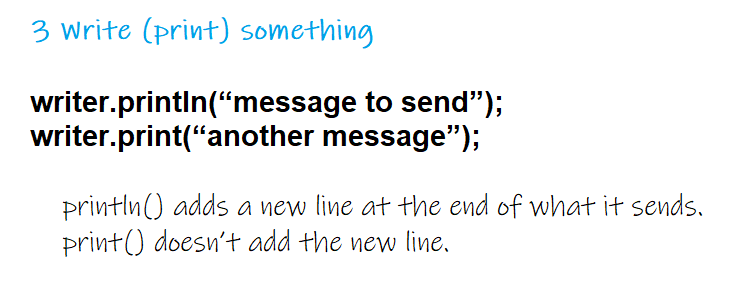
TimeServer is a server program that offers the current time. We’re developing a client for The TimeServer program, which connects to the server and retrieves a message (current time).
What exactly are you waiting for? Who knows what opportunities you would have missed if this app had not been available.
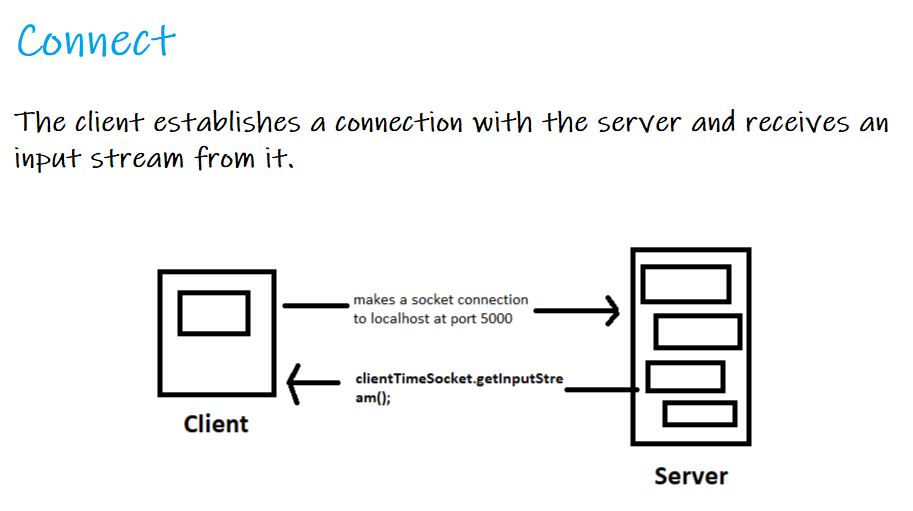
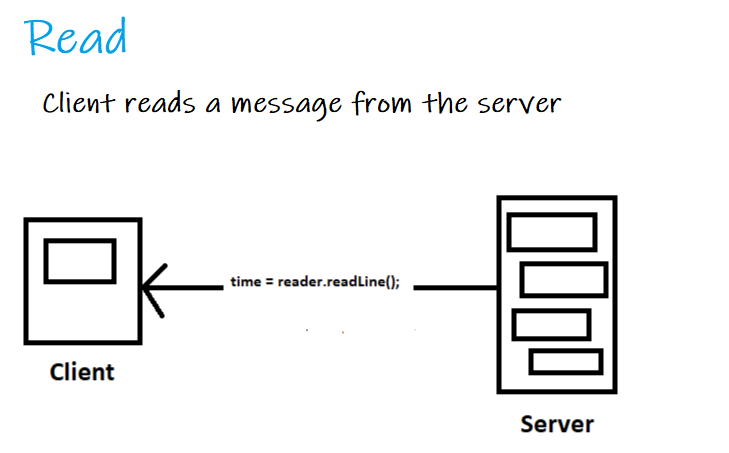
TimeServer Client Code:
This program makes a Socket, makes a BufferedReader (with the help of other streams), and reads a single line from the server application (whatever is running at port 5000).
// A Java program for a Client
import java.net.*;
import java.io.*;
public class Client
{
private String address;
private int port;
public Client(String address, int port){
this.address = address;
this.port = port;
}
public void go() {
try {
Socket clientTimSocket = new Socket(address, port);
InputStreamReader stream = new InputStreamReader(s.getInputStream());
BufferedReader reader = new BufferedReader(stream);
String time = reader.readLine();
System.out.println("Current Time is: " + time) ;
reader.close();
} catch(IOException ex) {
ex.printStackTrace();
}
}
public static void main(String args[])
{
Client client = new Client("127.0.0.1", 5000);
client.go();
}
}
Writing A Simple Server
So, what exactly does it take to create a server application? There are only a few Sockets. Yes, a couple of two. A ServerSocket that waits for client requests (when a client calls new Socket()) and a regular Socket socket to communicate with the client.
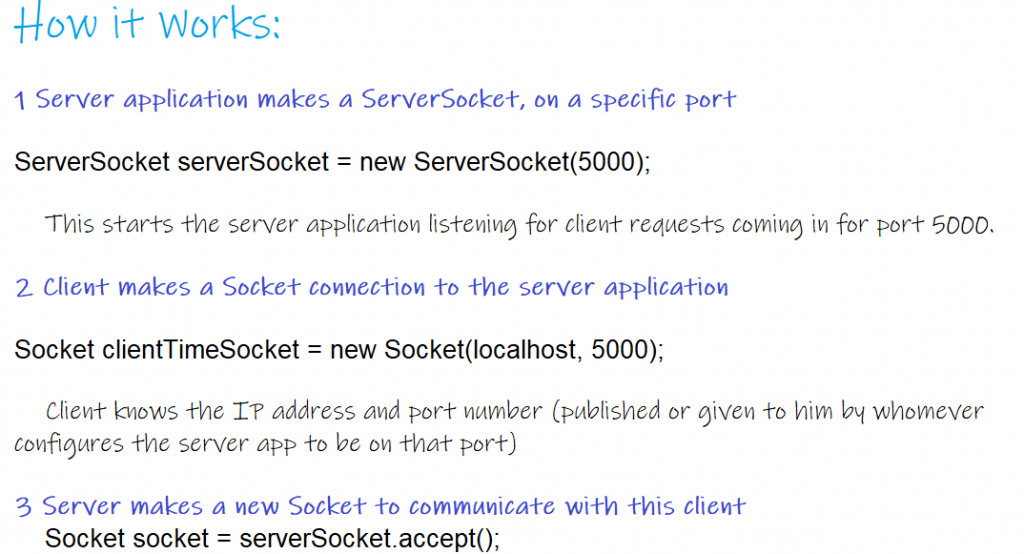
While waiting for a client Socket connection, the accept() method blocks (sits). When a client finally attempts to connect, the method returns a plain old Socket (on a different port) that knows how to communicate with the client (i.e., knows the client’s IP address and port number). The Socket is on a different port than the ServerSocket, allowing the ServerSocket to resume waiting for other clients.
TimeServer Server Code
This program makes a ServerSocket and listens for client requests. When the server receives a client request (i.e. the client says new Socket() for this application), it creates a new Socket connection to that client. The server creates a PrintWriter (using the output stream of the Socket) and sends a message to the client.
import java.io.*;
import java.net.*;
import java.util.Date;
public class TimeServer {
private int port;
private ServerSocket serverSocket;
public TimeServer(int port){
this.port = port;
}
public void go() {
try {
serverSocket = new ServerSocket(port);
while(true) {
Socket socket = serverSocket.accept();
PrintWriter writer = new PrintWriter(socket.getOutputStream());
String time = getCurrentTime();
writer.println(time);
writer.close();
System.out.println(time);
}
} catch(IOException ex) {
ex.printStackTrace();
} finally{
try {
if(serverSocket != null){
serverSocket.close();
}
} catch (Exception e) {
// TODO: handle exception
}
}
} // close go
private String getCurrentTime() {
Date date = new Date();
String time = date.toString();
return time;
}
public static void main(String[] args) {
TimeServer server = new TimeServer(5000);
server.go();
}
}