Everyone who writes software wants their code to be as simple to test, as maintainable, and as modular as a well-oiled machine. Dependency injection (DI), the design pattern superhero, steps in to help developers accomplish precisely that. However, what precisely is DI and how does it function?
Consider DI as a means of de-tangling your code. It’s all about keeping things loosely coupled, which means components of your code aren’t tightly bound to each other. Rather, they depend on abstractions, which enable the replacement of a component without creating havoc.
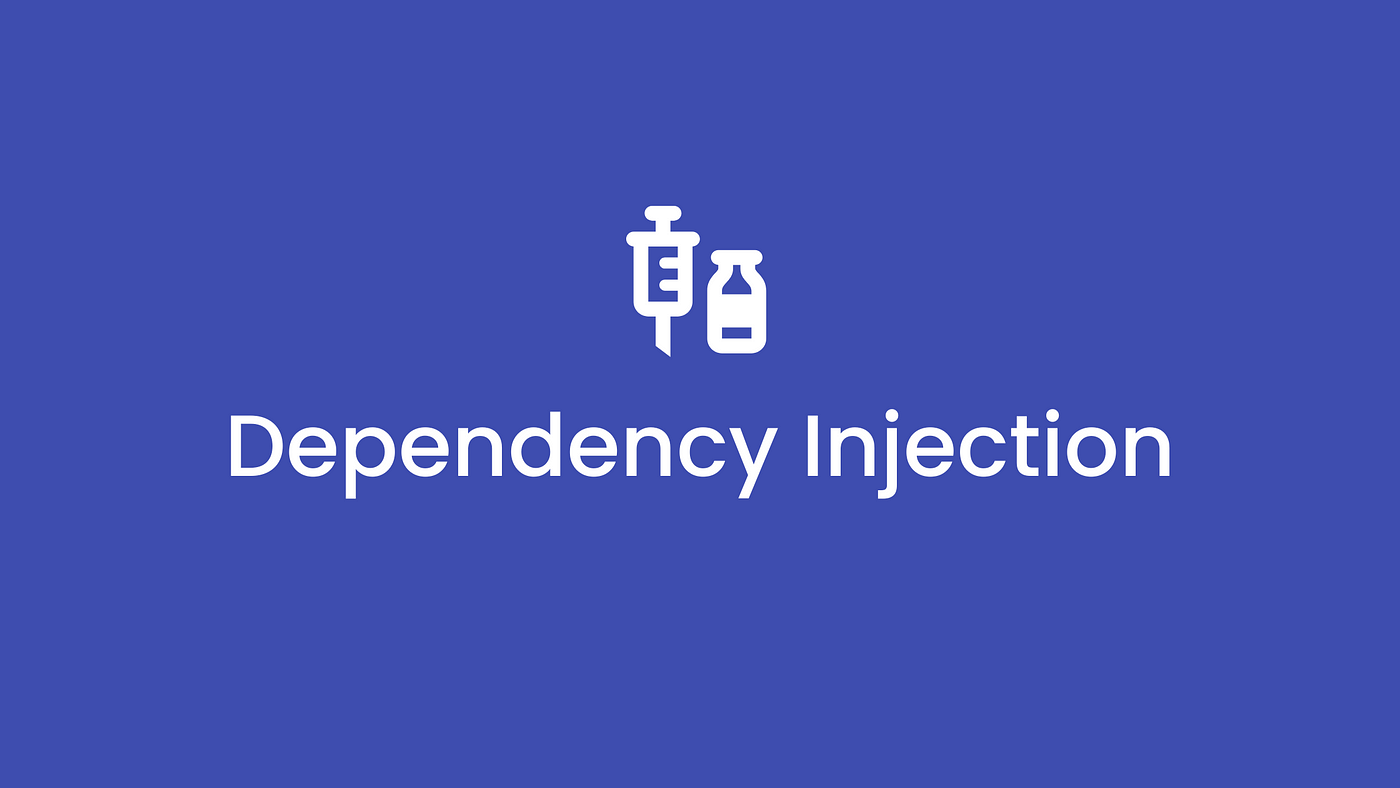
Why, therefore, is loose coupling so beneficial? Essentially, it increases the reusability of your code, which is quite beneficial when developing intricate applications. It also simplifies testing considerably. A well-decoupled code allows you to isolate the component you’re testing and ensure that everything functions as it should by simply swapping in fake objects.
We’re going to go deep into the realm of dependency injection in this guide. Everything will be covered, from the fundamental ideas to the specifics of how things are implemented. Finally, we’ll look at how to use DI in practical settings so you can see firsthand how it can improve your software development skills. So fasten your seatbelts because we’re about to take you on a thrilling adventure into the dependency injection realm!
Understanding Dependency Injection
Dependency injection is a strong programming approach that is essential to creating software systems that are adaptable and durable. Fundamentally, it frees a class from being inextricably linked to its dependents, promoting autonomy and upholding SOLID principles—in particular, the concepts of dependency inversion and single responsibility.
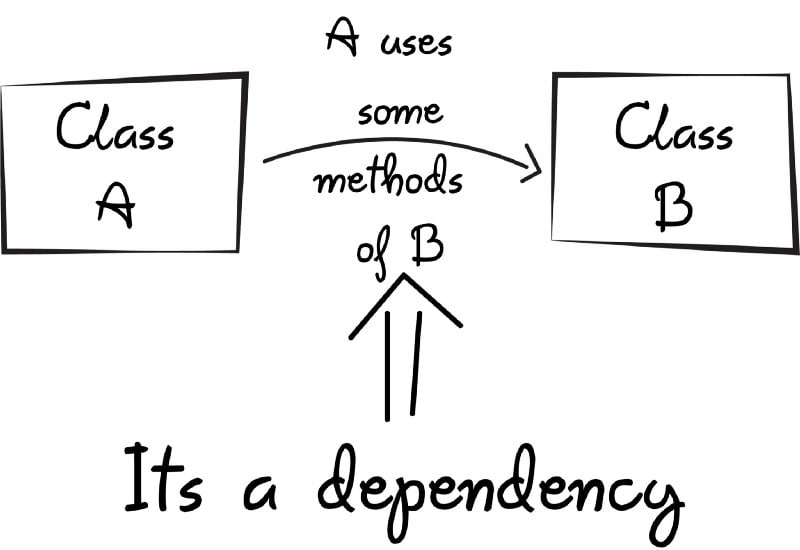
Dependency injection essentially separates an object’s construction and usage, which is in line with the goals of the SOLID principles, which include maximizing code reuse and reducing the frequency of class alterations. Dependency injection allows developers to easily swap dependencies without requiring changes to the consuming class by severing the direct connection between a class and its dependents. This preserves stability and lessens the possibility of unforeseen side effects by minimizing the danger of cascade changes throughout the codebase.
Traditionally, dependency injection was replaced by the service locator pattern. Dependency injection, on the other hand, has become the standard method in modern software development, and many application frameworks now integrate it into their main features. The technical parts of dependency injection are streamlined by these frameworks, freeing up developers to concentrate on building reliable, maintainable software and business logic implementation.
Java Dependency Injection
Since the theory behind Java Dependency Injection sounds a bit confusing, let’s start with a basic example and see how the dependency injection pattern can be used to accomplish loose coupling and extendability in an application. Assume we have an application that sends emails using EmailService. Typically, we would do this as seen below.
public class EmailService
{
public void sendEmail(String message, String receiver)
{
//logic to send email
System.out.println("Email sent to "+receiver+ " with Message="+message);
}
}
The logic to send an email message to the recipient’s email address is stored in the EmailService class. This is how our application code will look.
public class MyApplication
{
private EmailService email = new EmailService();
public void processMessages(String msg, String rec)
{
//do some msg validation, manipulation logic etc
this.email.sendEmail(msg, rec);
}
}
The client code that will send emails using the MyApplication class looks like this.
public class MyLegacyTest
{
public static void main(String[] args)
{
MyApplication app = new MyApplication();
app.processMessages("Hi Pankaj", "[email protected]");
}
}
At first glance, the implementation described above appears to be in good shape. However, the above code logic has certain restrictions.
- The email service must be initialized and used by the MyApplication class. A hard-coded reliance results from this. Future code modifications in the MyApplication class will be necessary if we decide to go to a different sophisticated email service. This makes it difficult to expand our program, and it would be even more difficult if email service was utilized in more than one class.
- We would need to create a separate application if we wanted to add more messaging functionality to ours, such as Facebook messages or SMS texts. Both the client and application classes’ code will need to change as a result.
- Since our application creates the email service instance directly, testing the application will be quite challenging. We are unable to use these objects as mocks in our test classes.
It may be argued that by including a constructor that takes email service as an argument, we can do away with the need to create email service instances in the MyApplication class.
public class MyLegacyTest
{
public static void main(String[] args)
{
MyApplication app = new MyApplication();
app.processMessages("Hi Pankaj", "[email protected]");
}
}
However, in this instance, we are requesting that test classes or client apps initialize the email service, which is a poor design choice. Let’s now examine how the Java Dependency Injection Pattern may be used to address every issue with the aforementioned implementation. In Java, dependency injection needs the minimum of these:
- Base classes or interfaces should be used in the design of service components. Interfaces or abstract classes that specify the services’ contract are preferable.
- Writing consumer classes should be done with the service interface in mind.
- The consumer classes will be initialized once the injector classes have initialized the services.
The Components of Dependency Injection
- Dependency: In this context, an item or service that is necessary for another object to operate is referred to as a dependence. Dependencies may be in the form of primitive data types, classes, or interfaces.
- Client: The class or component that needs dependence to carry out its duties is known as the client. The class in question is the one that will get the inserted dependency.
- Injector: The injector is in charge of giving the client the required dependencies. By resolving and injecting the dependencies into the client class, it serves as an intermediate.
Types of Dependency Injection
There are several ways to do dependency injection. These are the three main techniques:
- Constructor Injection: This method involves injecting dependencies via the constructor of a class. By doing this, it is guaranteed that the object will be created with the necessary dependencies accessible. Because constructor injection is clear and unchangeable, it is the most popular and advised type of DI.
- Setter Injection: To set the dependencies of a client class, a setter injection is used. It gives flexibility, but if not used properly, it may also result in partly initialized objects.
- Method Injection: Dependencies are given as arguments to certain methods when they are required via method injection. Although less prevalent, this strategy might be helpful in situations when dependencies are only needed for particular tasks.
The 4 roles in dependency injection
You need classes that fit into four fundamental roles to apply this strategy. These are the following:
- The desired service to utilize.
- the person using the service as a customer.
- an interface that the service implements and the client uses.
- The service instance is created by the injector and injected into the client.
By adhering to the dependency inversion concept, you already carry out three of these four responsibilities. By creating an interface, the dependency inversion concept aims to eliminate the reliance between the two classes, the service and the client.
It is possible to directly inject the service object into the client and forego the interface role. However, by doing so, you violate the idea of dependency inversion, and your client becomes explicitly dependent on the service class. In certain cases, this might be acceptable. However, in most cases, it’s preferable to establish an interface to break the client-service implementation dependence.
The only role exempt from the dependency inversion principle is the injector. But since you don’t have to use it, it isn’t a problem. There are ready-to-use implementations of it in all of the frameworks that I mentioned at the beginning of this post.
As you can see, apps that adhere to the dependency inversion concept can benefit greatly from dependency injection. The dependency injection approach allows you to eliminate the last dependent on the service implementation since you have already implemented the majority of the necessary roles.
Best Practices and Common Pitfalls
Effective dependency injection implementation necessitates following best practices and being aware of typical dangers that may negate its advantages. Let’s take a closer look at these advantages and disadvantages:
- Designing Classes with Single Responsibilities: To guarantee that there is only one cause for a class to change, adhere to the Single Responsibility Principle (SRP). This makes dependency management easier and encourages greater code structure.
- Using Constructor Injection by Default: Whenever feasible, use constructor injection rather than setter injection. By guaranteeing that dependencies are available during object instantiation, constructor injection enhances immutability and lowers the possibility of null references.
- Avoiding Excessive Dependency Injection Frameworks or Libraries: dependency injection frameworks can make managing dependencies easier, but only use the capabilities that are essential to avoid adding needless complexity. Analyze the trade-offs of manually implementing dependency injection and utilizing a framework.
- Applying Interface Segregation Principle (ISP): Create interfaces with the fewest possible methods that the dependant class needs. This encourages adaptability and keeps classes from being made dependent on interfaces they don’t utilize.
- Documenting Dependency Contracts and Lifetime: Record the dependencies needed by every class, along with their lifespan, intended behaviors, and any external dependencies they may have. This documentation facilitates correct integration and maintenance by assisting developers in understanding the function and application of each dependency.
- Using Named Constructors for Complex Dependency Configurations: Use named constructors or factory methods to give simple and obvious instantiation alternatives when a class has to supply various dependencies or settings.
Common Pitfalls and How to Avoid Them
- Overusing Setter Injection: When setter injection is used excessively, mutable dependencies may result, which makes it challenging to reason about the state of objects. For required dependencies, use constructor injection; save setter injection for optional or dynamic settings.
- Introducing Unnecessary Complexity with Excessive Indirection: Steer clear of employing excessively intricate dependency injection techniques or adding too many levels of abstraction to prevent introducing unnecessary indirection. Use dependency injection sparingly to preserve a minimal and manageable codebase.
- Failing to Consider the Lifetime and Scope of Injected Dependencies: Consider the lifespan and extent of injected dependencies carefully, particularly in multithreaded or long-running programs. Make sure that dependencies are disposed of and maintained appropriately to avoid memory leaks and resource depletion.
- Inconsistent Naming Conventions for Dependencies: To increase readability and maintainability, keep naming conventions for dependencies consistent throughout the project. Give each dependent a name that correctly conveys its function and significance.
Finally, dependency injection is shown as a key design pattern in object-oriented programming with broad ramifications. Its importance in contemporary software engineering methods is highlighted by its capacity to promote loose coupling, improve code maintainability, and increase testability.
Developers may fully utilize dependency injection by following best practices, avoiding typical errors, and investigating sophisticated solutions. This gives them the ability to create software programs that are robust to changing needs and complexity, in addition to being scalable and modular.
Dependency injection continues to be a solid cornerstone of contemporary software engineering, whether traversing web development frameworks, setting up extensive testing environments, or designing complex microservices solutions. It acts as a beacon of light, empowering developers to confidently, creatively, and relentlessly pursue excellence while navigating the complexities of software design.