The foundation of effective and maintainable software development is software design patterns. These are the tried-and-true fixes for the common design issues that developers run into when working on projects. We will delve deeply into software design patterns in this extensive guide, examining their categories, histories, and practical applications.
Why Design Patterns?
Recently, there has been some debate in the programming community about design patterns, mostly because of the belief that using them excessively can result in code that is more difficult to read and maintain.
It is imperative to realize that Design Patterns were not intended to be arbitrarily combined into shortcuts and applied to your code in a “one-size-fits-all” fashion. In software engineering, true problem-solving skills are ultimately indispensable.
Nonetheless, it is still true that, when applied appropriately, Design Patterns can be constructive. When applied wisely, they can greatly increase a programmer’s productivity by preventing them from having to “invent the wheel” and instead utilizing techniques that have already been perfected by others. They also offer a helpful common language to conceptualize recurring issues and their fixes when having conversations with others or overseeing code in bigger groups.
Categories of Design Patterns
The three primary categories of design patterns are behavioral, structural, and creational patterns. Every category has a unique function in addressing particular kinds of design issues.
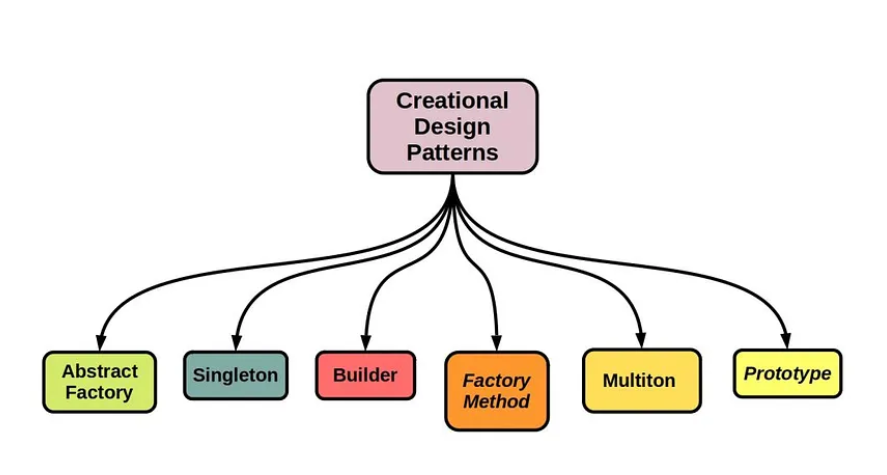
Creational Patterns: Mechanisms for creating objects are covered by creational design patterns. They offer methods for object creation that conceal the instantiation logic, increasing the system’s flexibility and decoupling.
Singleton Pattern (The Distinctive Element)
The Singleton Pattern ensures a class has only one globally accessible instance, much like there is only one Mona Lisa painting. This is especially helpful for resources like database connections that ought to be shared. Ensuring that a class only has one point of access avoids the needless duplication of resources.
Pros :
- Single Instance: makes sure a class only has one instance for the duration of the application. Managing resources that need to be shared by the entire application can benefit from this.
- Global Access Point: makes it simple to retrieve the unique instance from any area of the application by offering a centralized access point.
- Memory Conservation: The Singleton pattern can help save resources in cases where making multiple instances of a class uses a lot of memory or other resources.
Cons :
- Testing Challenges: Writing unit tests for classes that rely on a singleton can be tough because of the global state, as it can be hard to isolate and mock the singleton instance.
- Potential for Overuse: Overuse of the Singleton pattern can result in an overabundance of global states and make it more difficult to understand how data flows through an application.
- Subclassing Challenges: It can be challenging to subclass a Singleton because extra caution must be taken to guarantee that the subclass creates and manages its unique instance appropriately.
Factory Pattern (Crafting Flexibility)
Picture a busy factory that manufactures a range of goods without requiring the precise details of each one to be known. In a similar vein, your code can create objects without defining their precise class thanks to the Factory Pattern. Because it allows for the seamless integration of new products (classes) without requiring changes to the existing code, this flexibility improves code maintainability and extension.
Pros:
- Encapsulation of Object Creation Logic: The factory class contains all the functionality needed to create objects. This facilitates loose coupling between the client and the created objects by removing the need for the client code to understand the intricacies of object creation.
- Centralized Control: The factory serves as the hub for the production of goods. When you want to run extra checks or logic before creating an object, this can be helpful.
- Supports Open/Closed Principle: The Open/Closed Principle is supported by the Factory Pattern, allowing you to add new subclasses and change the existing code without changing the system’s behavior.
Cons:
- Potential Overhead: There may occasionally be a slight performance overhead due to the factory’s added degree of indirection. Modern compilers, however, can frequently optimize this to a very small degree.
- Can Lead to a Large Number of Factory Classes: Multiple factory classes might be required in complex systems with a wide variety of object types, which could eventually result in an overabundance of factory classes.
- Knowledge of Subclasses is Necessary: The subclasses that the factory is capable of creating must be known to it. This implies that the factory might need to be modified to make room for new subclasses if they are introduced.
Abstract Factory Pattern
Without defining their concrete classes, the Abstract Factory pattern offers an interface for building families of related or dependent objects. It lets you design things that integrate like a factory of factories.
Pros:
- Concrete Class Isolation: The interaction between the client code and the abstract interfaces keeps it separate from the concrete implementations. This facilitates loose coupling and makes switching between object families simpler.
- Supports Open/Closed Principle: It makes it simple to extend by adding new object families (concrete factories) without changing the client code already in place. The Open/Closed Principle is supported by this.
- Ease of Unit Testing: Client code is less dependent on concrete classes and more dependent on abstract interfaces, which makes it simpler to mock or replace implementations during testing.
Cons:
- Complexity: placing the Abstract Factory pattern into practice may add more complexity, particularly when there are several families of related objects with various variants.
- Not Suitable for Small-Scale Applications: Implementing the Abstract Factory pattern may not be worth the overhead in small applications or situations where there are few related object families.
- Static Configuration: The flexibility for dynamic configuration may be limited if the concrete factory to use is decided upon at compile time or application startup.
Builder Pattern
This pattern divides the process of creating a complex object into its representation and its construction. This keeps the construction process consistent while enabling you to create objects step-by-step with a wide range of configuration options.
Pros:
- Separation of Concerns: Different builder implementations can produce distinct representations of the same product because the Builder pattern isolates the construction process from the representation.
- Flexibility in Object Creation: The pattern makes it possible to build several iterations of an object with the same construction method. This is especially helpful when making objects with features that are configurable or optional.
- Supports Fluent Interface: Because a fluent interface makes setting an object’s properties more natural and intuitive, builders frequently use it.
Cons:
- Potential for Redundancy: The builder pattern can result in redundant code if it is not used carefully, particularly if the construction process has a lot of identical steps.
- Pattern Prototype: a pattern that makes it possible to create new objects by replicating an already-existing one (the prototype).
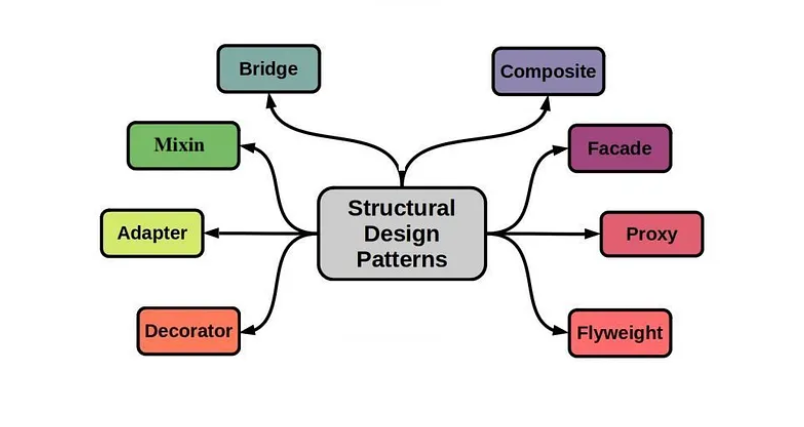
- Structural Patterns: By emphasizing the way classes and objects are put together, structural design patterns facilitate the formation of larger, more intricate structures while maintaining their adaptability and efficiency.
Bridge Pattern
The Bridge pattern distinguishes between the implementation and abstraction of an object. It lets you modify the implementation specifics without affecting the client code. This is particularly helpful if you wish to support several databases or platforms.
Pros:
- Decouples Abstraction from Implementation: The abstraction (an interface or abstract class) and the implementation (concrete classes or implementations) are distinguished by the Bridge pattern. Changes in one can be made without impacting the other thanks to this decoupling.
- Allows for Run-Time Binding: The implementation can be selected at runtime thanks to the Bridge pattern. This can be useful when deciding which implementation to use is a dynamic process.
- Reduces Class Explosion: You could wind up with a plethora of subclasses for various combinations of abstractions and implementations if the Bridge pattern weren’t used. This class explosion can be prevented by using the Bridge pattern.
Cons:
- Potential for Over-Engineering: The Bridge pattern can result in over-engineering if it is not used carefully, which would make the codebase more complex than is necessary.
- Increased Number of Classes: An increase in the number of classes in the codebase due to the introduction of abstractions and implementations may result in higher complexity and maintenance costs.
- Potential for Misuse: It’s possible to apply the Bridge pattern excessively or in circumstances where more straightforward structural patterns are adequate.
Adapter Pattern (Bridging Gaps)
An adapter bridges the gap between incompatible parts by changing the plug on your device into one that fits the socket. Comparably, the Adapter Pattern translates a class’s interface into an interface that clients expect, facilitating the smooth cooperation of incompatible parts.
Pros:
- Enables Integration of Incompatible Interfaces: When integrating third-party or legacy code into a system, the Adapter pattern can be especially helpful in making objects with incompatible interfaces work together.
- Maintains Separation of Concerns: Adapters help to manage and maintain the two parts independently by separating the concerns of the new code from those of the existing code.
- Minimizes Impact on Existing Code: The risk of introducing bugs is reduced when adapters are added without necessitating major modifications to the current codebase.
Cons:
- Performance Overhead: The addition of adapters often results in a slight performance hit due to the additional indirection.
- Requires Careful Design: Adapter design can be difficult to do well. To make sure the adapter properly fills in the gap between the incompatible interfaces, careful consideration is required.
- Adds Another Layer of Indirection: Because adapters add another level of indirection, their use can make the code more difficult to understand.
Decorator Pattern (Topping Your Code)
A pizza’s flavor is improved by adding toppings without changing the fundamental ingredients. Likewise, the Decorator Pattern dynamically expands an object’s scope of responsibility without changing the object’s fundamental structure. This promotes code efficiency and scalability by providing a flexible substitute for creating multiple subclasses.
Pros:
- Preserve Single Responsibility Principle: Every decorator class is in charge of just one issue. This prevents various responsibilities from being mixed into a single class, which encourages a clean and organized code.
- Flexible Extension of Functionality: Decorators can be stacked to give an object multiple levels of behavior. This makes it possible to have precise control over an object’s functionality.
- Dynamic Addition of Functionality: Runtime additions and deletions of decorators enable dynamic behavior modification of an object.
Cons:
- The Decorators’ Order: Applying decorators in a certain order can have an impact. The behavior of the final object may change depending on which decorators are added in what order, depending on the details of the implementation.
- Increased Memory Usage: The addition of a layer to an object by each decorator may increase memory usage, particularly when multiple decorators are applied.
- Not Suitable for All Scenarios: In simpler scenarios where behavior can be readily added by composition or direct subclassing, the overhead associated with decorators might not be worth it.
Proxy Pattern (Virtual Representation)
A proxy is a celebrity’s stand-in at events, similar to a body double. By acting as a virtual representative, the Proxy Pattern restricts access to an item. Additionally, it can include extra features like lazy loading. This pattern can handle interactions with complex objects in the same way that a proxy manages interactions with a celebrity.
Pros:
- Controls Access to an Object: You can manage who has access to the actual subject object by using the Proxy pattern. For adding security checks, logging, or lazy initialization, this can be helpful.
- Caching: By storing the outcomes of pricey operations, proxies can reduce the need to repeat those operations repeatedly. This is known as caching.
- Increases Safety: Access control policies can be enforced by proxies, guaranteeing that only authorized users can carry out specific tasks.
Cons:
- May Introduce Latency: Because proxies add a layer of indirection, they may introduce some latency depending on the details of the implementation.
- Can Complicate Debugging: Debugging through the additional layer of indirection when using proxies may be required, and this can be more difficult than working directly with the original object.
- Requires Careful Design: It can be challenging to properly design and implement proxies, particularly when there are numerous varieties with varying roles.
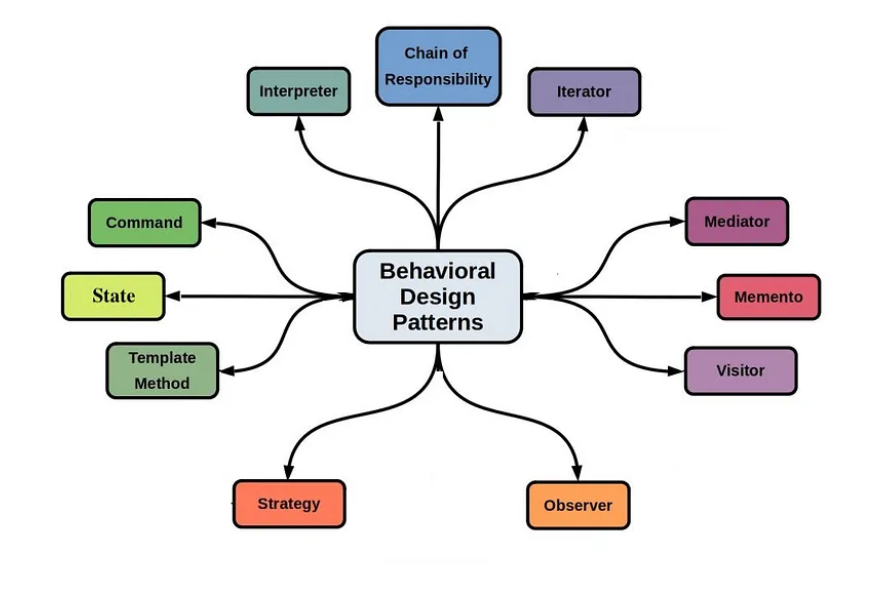
Behavioral Patterns: Behavioral design patterns define the patterns of communication between objects by examining how they interact and communicate with one another.
Command Pattern (Your Program’s To-Do List)
Envision a to-do list where tasks are listed as concrete actions. In a similar vein, the Command Pattern allows clients to be parameterized with various requests by encapsulating requests as objects. This pattern encourages code flexibility by making it easier to queue up requests and supporting undo operations.
Pros :
- Decouples Sender and Receiver: More flexibility and decoupling between the sender and the processing object is made possible by the Command pattern, which divides the two.
- Supports Undo Operations: Putting undo functionality in place is made simpler by the Command pattern. Every command can store the state required to undo an operation.
- Facilitates Asynchronous Execution: Asynchronous command execution is advantageous in situations requiring background or concurrent processing.
Cons :
- Potential for Command Explosion: The codebase may grow more complex and challenging to manage in scenarios with a lot of distinct commands.
- Can Increase Development Time: Compared to directly invoking methods, implementing the Command pattern might take more time and effort, especially in simpler scenarios.
- Potential for Misuse: The Command pattern can cause over-engineering and an unnecessarily complex codebase if it is not used carefully.
State Pattern (Adapting to Changes)
The State Pattern enables objects to change their behavior when their internal state changes, much like a traffic light reacting to changes in traffic flow. This promotes modularity and maintainability by preventing code from growing unnecessarily complex when new states are added.
Pros :
- Scalable and Modular: The State pattern encourages the management of state-specific behavior in a neat and orderly manner. Since each state is contained within a separate class, adding, modifying, and removing states is simple.
- Promotes Clean Code: Code that is cleaner and easier to maintain is produced when concerns are separated according to the State pattern.
- Facilitates State Transitions: State transitions can be handled simply thanks to the State pattern. This can be helpful when changing between states calls for particular steps or verifications.
Cons :
- Could Be Too Much for Simple Systems: The overhead of implementing the State pattern might not be worth it for systems that are small in size or in scenarios where there aren’t many states.
- Debugging Difficulties: Comparing more complicated systems with direct method calls to simpler ones can make debugging through several state classes more difficult.
- May Introduce Complexity: In simpler scenarios, introducing the State pattern may be overkill because it introduces yet another layer of complexity.
Template Pattern (Crafting Standard Processes)
The Template Pattern establishes an algorithmic structure in the same way that a recipe offers a consistent method for preparing food. Certain steps can be skipped by subclasses while the overall procedure is still followed. This is ideal for developing workflows that can be reused, guaranteeing consistent behavior between different implementations.
Pros :
- Defines a Common Algorithm Structure: The Template Method pattern offers a structure for specifying an algorithm’s steps. This aids in preserving a uniform structure among various implementations.
- Enforces Encapsulation: The implementation details of each step are contained in separate methods, and the template method manages the algorithm’s overall flow. This aids in keeping the code neat and structured.
- Facilitates Consistent Behavior: The Template Method pattern helps guarantee that specific actions are carried out in a particular order, offering a uniform behavior amongst various implementations.
Cons :
- May Lead to Overuse: The Template Method pattern can result in overuse or improper application if it is not used carefully, adding unnecessary complexity to the codebase.
- Potential for Limited Customization: If certain steps are closely coupled, subclasses might not be able to modify the algorithm much depending on how it is designed.
- May Not Be Suitable for All Scenarios: Other patterns or approaches might be more appropriate in situations where the algorithm is extremely dynamic or complex variations are needed.
Strategy Pattern (Agile Strategy Switching)
Imagine a flexible gaming character who can transition between various tactics while playing. Switching between algorithms at runtime is simple and possible with the Strategy Pattern. This helps to make your codebase more flexible by offering multiple ways to complete a task.
Pros :
- Facilitates Code Maintenance: The corresponding strategy class allows for updates or changes to be made to a particular algorithm without affecting other areas of the codebase.
- Reduces Conditional Statements: It makes code cleaner and easier to maintain by removing the need for conditional statements to choose between various behaviors.
- Simplifies Testing: Because each strategy can be tested separately, testing becomes easier and individual algorithms can be tested more thoroughly and with greater focus.
Cons :
- May Lead to Overuse: The Strategy pattern can cause over-engineering and an unnecessarily complex codebase if it is not applied carefully.
- Potential for Code Duplication: Code duplication could result from shared code or data between various strategies, depending on how they are implemented.
- May Require Extra Effort to Set Up: Compared to simpler methods, setting up and configuring the strategy pattern might take more work, especially for straightforward scenarios.
Why do we use it? What are the benefits?
There are various advantages to using design patterns in software development, such as:
- Scalability and Flexibility: As project requirements change, design patterns make it easier to create architectures that are both scalable and flexible.
- Code Reusability: Design patterns provide reusable solutions, allowing programmers to take advantage of pre-existing solutions rather than having to start from scratch.
- Reduced Errors: Design patterns help lower the likelihood of introducing errors or common pitfalls in the codebase by adhering to tried-and-true solutions.
- Streamlined Development Process: Design patterns offer pre-made solutions that help developers communicate and work together more efficiently by creating a common vocabulary and understanding.
- Maintainability: Design patterns help to make code more structured and organized, which facilitates troubleshooting and modification.
Finally, in the field of software development, learning design patterns is like having a powerful toolkit at your disposal. These tried-and-true answers to typical issues offer a road map for creating dependable, expandable, and maintainable applications. Every pattern—from creational to behavioral to architectural—offers a unique strategy for handling problems. Follow best practices, identify anti-patterns, and use these patterns sparingly to enable developers to design elegant and effective solutions. Case studies from real-world situations serve to further illustrate their usefulness. Equipped with this understanding, you can confidently negotiate the intricacies of software engineering. Accept design patterns as a fundamental component of your development process, and allow them to open the door to creative, flexible, and future-proof software solutions.