In the realm of object-oriented programming (OOP), two essential techniques, inheritance, and composition, serve as building blocks for crafting intricate software systems. While both are powerful, they offer distinct approaches to structuring code and managing relationships between classes.
Inheritance operates on the principle of hierarchy, where a new class can inherit properties and methods from an existing class, known as the base or parent class. This allows for the reuse of code and promotes code organization. However, inheritance can lead to tight coupling between classes and can result in rigid, inflexible designs.
Conversely, composition stresses modularity and flexibility by emphasizing the building of things through connections as opposed to inheritance. By mixing basic components to create complex ones, composition promotes a more modular and loosely connected architecture. Greater flexibility and simplicity of maintenance for big software systems are made possible by this method.
To demonstrate the advantages of composition over inheritance in software development, we go into detail in this article and provide real-world Java examples. We illustrate how composition, as opposed to inheritance, encourages code reuse, improves flexibility, and allows for better code structure using real-world examples and code snippets.
Understanding Inheritance
A fundamental idea in object-oriented programming (OOP), inheritance encourages the development of hierarchical relationships between classes and makes code reuse easier. Fundamentally, inheritance enables characteristics and behaviors from an existing class (superclass or base class) to be inherited by a new class (subclass or derived class). This indicates that the members (variables, methods, and properties) declared in the superclass are accessible to and used by the subclass.
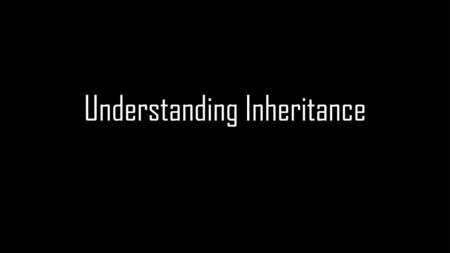
OOPs support the three different types of inheritance as given below:
- Single inheritance
- Multiple inheritance
- Hierarchical Inheritance
Single Inheritance: A derived class is produced in this inheritance process from a single base class.
Since Class B inherits the traits and characteristics of its parent class A, it is the child class in the example provided, and Class A is the parent class.
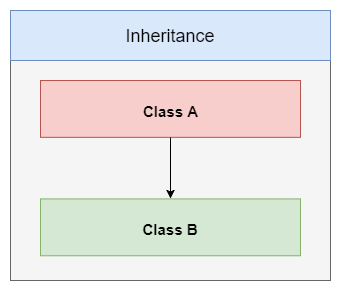
Example:
//Base Class
class A
{
public void fooA()
{
//TO DO:
}
}
//Derived Class
class B : A
{
public void fooB()
{
//TO DO:
}
}
Multiple Inheritance: A derived class is produced in this inheritance process from many base classes. Java language and .NET languages like C#, F#, and others do not allow this inheritance.
Class C in the example inherits class B and class A’s traits and behaviors at the same level. Thus, Class C’s parent classes are A and B in this instance.
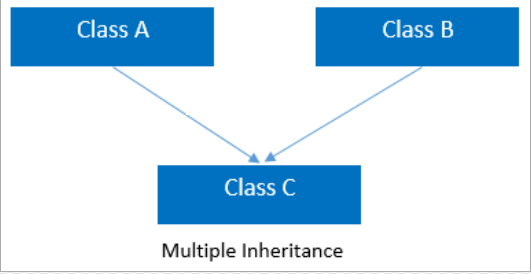
Example:
//Base Class
class A
{
public void fooA()
{
//TO DO:
}
}
//Base Class
class B
{
public void fooB()
{
//TO DO:
}
}
//Derived Class
class C : A, B
{
public void fooC()
{
//TO DO:
}
}
Hierarchical Inheritance: In this inheritance, a single base class gives rise to several derived classes, and additional child classes serve as parent classes to multiple child classes.
Class A has two offspring, classes B and D, in the example provided. Additionally, classes B and C each have two children, class D and E, or class F and G, respectively.
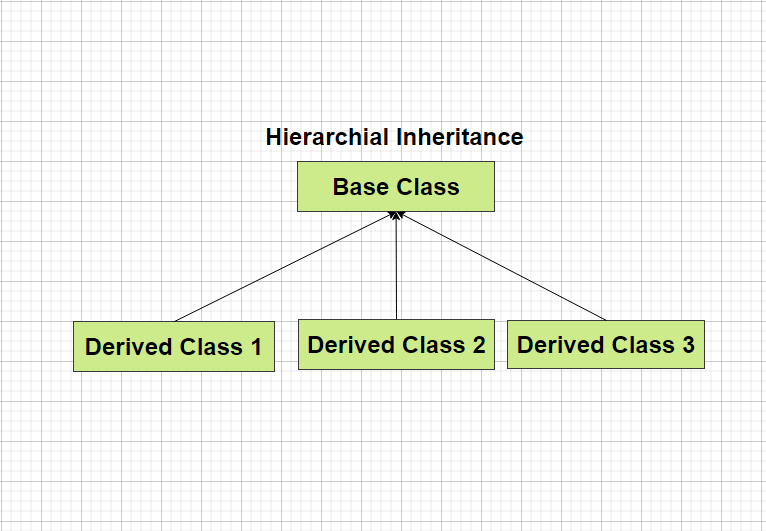
Example:
//Base Class
class A
{
public void fooA()
{
//TO DO:
}
}
//Derived Class
class B : A
{
public void fooB()
{
//TO DO:
}
}
//Derived Class
class C : A
{
public void fooC()
{
//TO DO:
}
}
//Derived Class
class D : C
{
public void fooD()
{
//TO DO:
}
}
//Derived Class
class E : C
{
public void fooE()
{
//TO DO:
}
}
//Derived Class
class F : B
{
public void fooF()
{
//TO DO:
}
}
//Derived Class
class G :B
{
public void fooG()
{
//TO DO:
}
}
Exploring Composition
A connection between items is called composition when one object comprises or is composed of another. An engine object, a wheel object, and a door object, for instance, can be combined to form an automobile object. The engine, wheel, and door objects do not pass any attributes or methods to the car object, but it is still able to utilize them for their purposes. A “has-a” connection is another name for composition, as the vehicle object contains wheels, doors, and an engine.
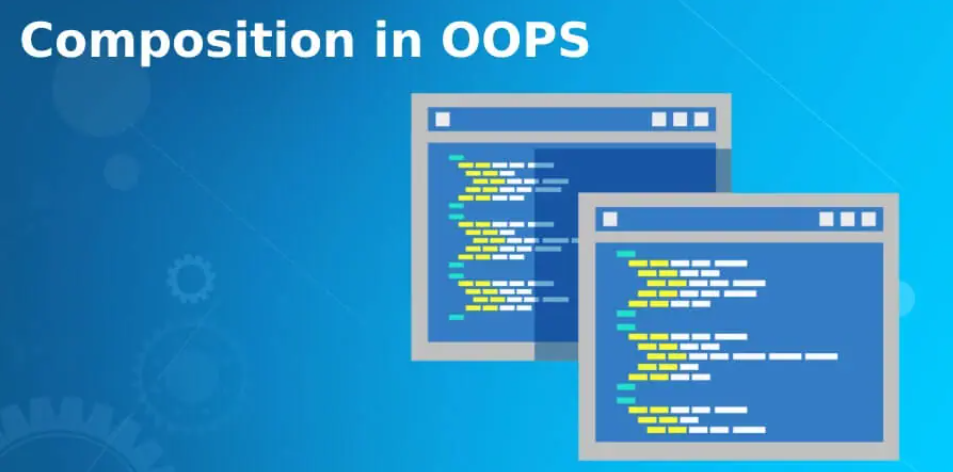
One well-liked OOP language that allows composition is Java. In Java, composition is implemented by first defining the classes of the component and the composite object, after which instances of the component are created and added to the composite object as fields or attributes. For instance, you might write something like this to build an object for a car with an engine, wheels, and doors:
Example:
// Define the component classes
class Engine {
// Some properties and methods
}
class Wheel {
// Some properties and methods
}
class Door {
// Some properties and methods
}
// Define the composite class
class Car {
// Declare the components as fields
private Engine engine;
private Wheel[] wheels;
private Door[] doors;
// Define a constructor that takes the components as parameters
public Car(Engine engine, Wheel[] wheels, Door[] doors) {
this.engine = engine;
this.wheels = wheels;
this.doors = doors;
}
// Define some methods that use the components
public void start() {
engine.start();
}
public void stop() {
engine.stop();
}
public void openDoor(int index) {
doors[index].open();
}
public void closeDoor(int index) {
doors[index].close();
}
}
// Create an instance of the composite object
Car car = new Car(new Engine(), new Wheel[4], new Door[4]);
What does composition over inheritance mean?
Composition over inheritance is a method of adding behavior to an object by composing objects instead of using inheritance. This approach has drawbacks, such as dependency and potential bugs. Inheritance can be difficult to maintain and requires understanding of both classes. Composition relies on using small classes with clear responsibilities, allowing for easy modification of functionality and creating new classes for diverging behavior. This approach makes it easier to change objects referencing than inheriting from a new class.
Advantages of Inheritance
Because inheritance has so many benefits, object-oriented programming requires it. First off, letting subclasses inherit public and protected methods and properties from the base class, encourages code reuse. Reimplementing the same code in each subclass would have required more time and effort, which is saved by doing this.
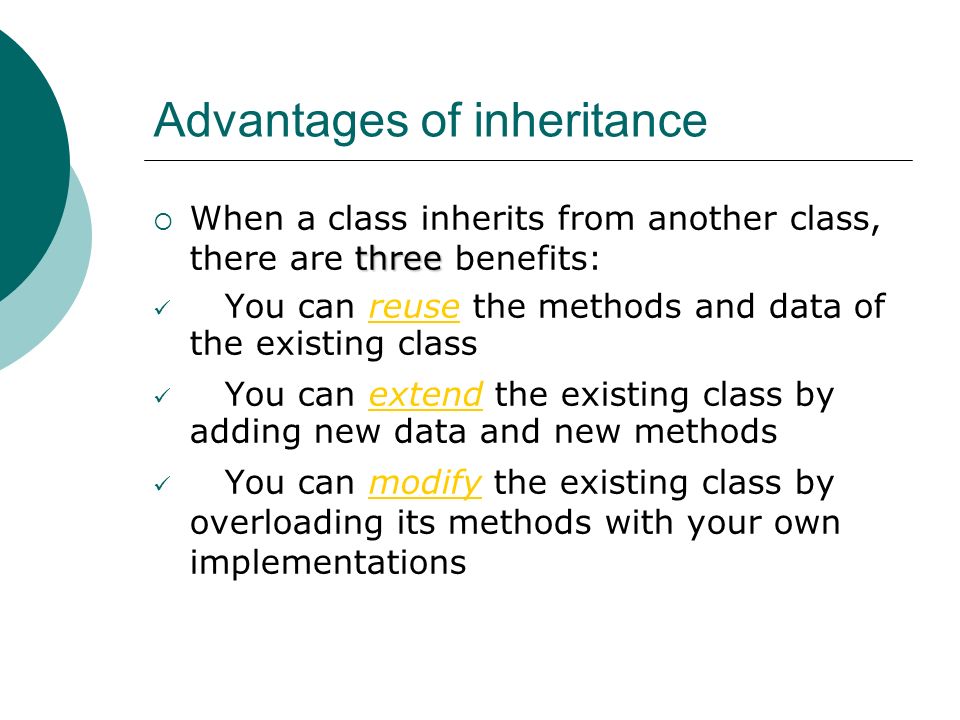
Moreover, polymorphism—which permits objects to assume several forms while preserving a common interface or base class—is encouraged by inheritance. This makes creating and implementing object-oriented systems more flexible.
Lastly, inheritance makes it easier to create hierarchies, which are useful for modeling intricate interactions between different classes. This facilitates system maintenance and modification over time, as well as system understanding.
Advantages of Composition
In software development, composition is a widely used approach with several benefits, especially in object-oriented programming. Composition has several advantages, chief among them being object-oriented system design and implementation flexibility, which lets programmers create more reliable and maintainable programs.
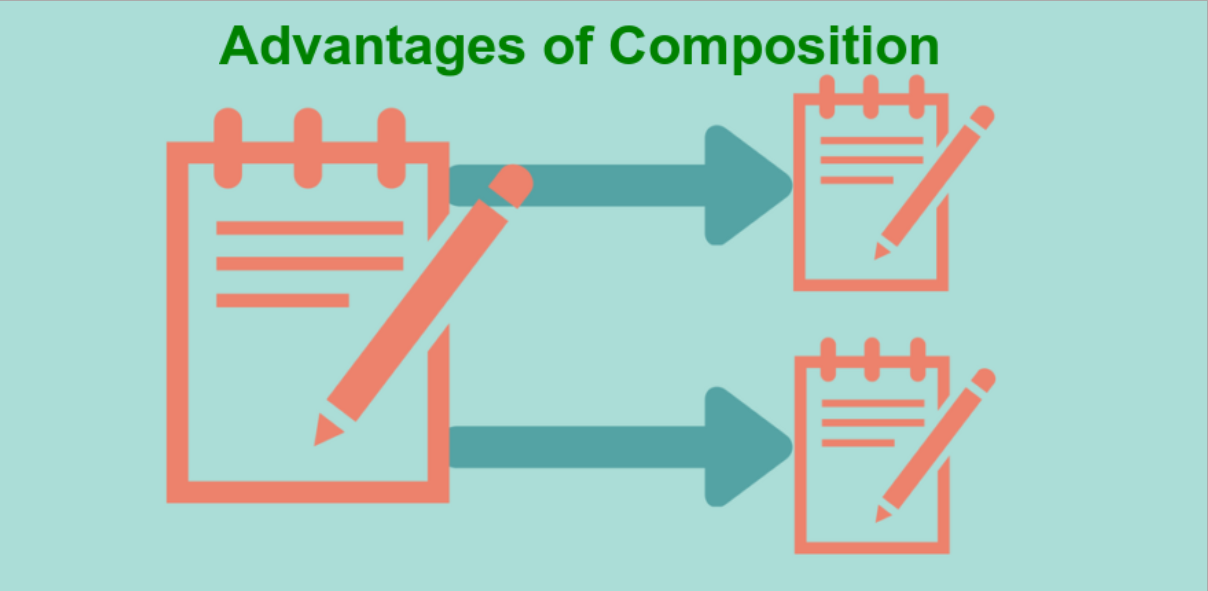
First, by enabling items to be constructed of other objects, composition encourages code reuse. Because of this, extending and changing an object’s behavior becomes simpler and doesn’t need changing the original code.
Furthermore, composition allows smaller, more specialized elements to be combined to create complex, hierarchical systems. More encapsulation and modularity are made possible by this, which facilitates understanding and maintenance of the code.
How to decide Inheritance or Composition?
The following conditions must be met for the inheritance to be applied.
- The subclass belongs to the superclass as a proper subtype.
- The subclass follows the notion of Liskov Substitution.
- The subclass is suitable for the superclass’s implementation.
- The additions or modifications to the superclass’s behavior are the main improvements made by the subclass.
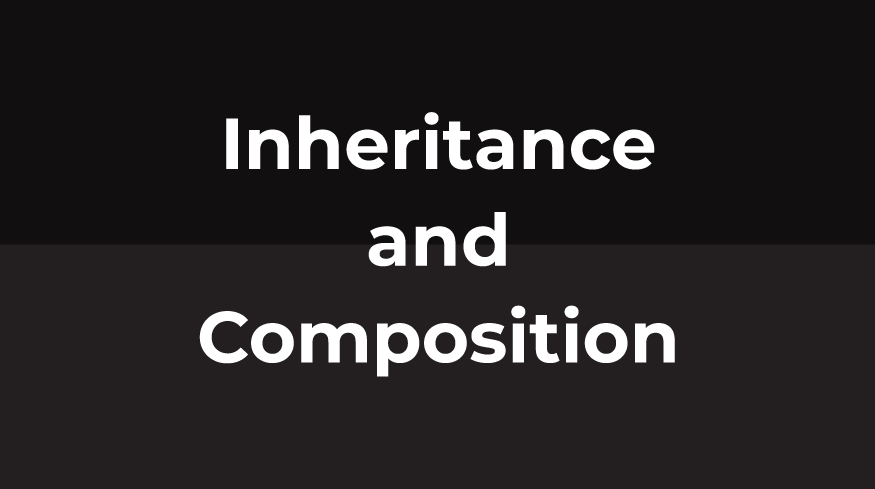
We ought to reconsider our connection if the kid class is eliminating the base class’s functionality. We are forced by inheritance to plan and organize the code appropriately. Since humans are not very skilled at predicting the future, inheritance should be used with caution.
The aforementioned requirements are typically met if we are writing.
- Structure or expansion of a structure.
- higher-level classes, such as BaseViewModel and BasePresenter, that are defined for software architectural purposes.
- programming with differentiation.
It is advised to employ composition if you are not executing the previously listed tasks. It certainly has advantages over inheritance and maintains loose coupling of the code. With the aid of composition, you may quickly mix and combine the behaviors to assist you in accommodating future changes.
Although the mixture should be used in most situations, it is not a miracle cure.
Inheritance should be used with caution. Use it for more than just reusing the code. When thinking about utilizing inheritance, examine if the subclass is a more specialized form of the superclass. Does it adhere to the idea of Liskov Substitution? Is it more sensible to utilize composition in this situation?
Best Practices
The particular requirements of your project and the trade-offs between the two strategies should be taken into account when choosing between inheritance and composition. The following are recommended practices to bear in mind:
- Apply inheritance to represent “is-a” connections: When modeling relationships that represent “is-a” relationships, such as a subclass representing a more specialized form of its superclass, inheritance is still a helpful technique. But use caution when designing extremely intricate class structures, as they might be challenging to uphold.
- Steer clear of deep inheritance hierarchies: To prevent the fragile base class problem and the combinatorial expansion of subclasses, inheritance hierarchies should ideally be kept relatively small.
- When constructing big items that need the aggregation of several smaller ones, composition is frequently a superior option. This can preserve the modularity and maintainability of the code.
- Whatever strategy you decide on, be careful to properly convey your design decisions to your team and to record them. When working with the codebase, this may assist in guaranteeing that there are no misunderstandings and that everyone is on the same page.
You may write code that is more reliable, manageable, and tailored to the particular requirements of your project by adhering to the guidelines provided here.
In object-oriented programming (OOP), inheritance and composition are crucial techniques for software design. inheritance allows code reuse and hierarchical structures, while composition promotes flexibility, modularity, and testability. However, inheritance can introduce rigidity.
On the other hand, composition offers a more versatile approach by allowing objects to be assembled through relationships rather than strict inheritance. This fosters a modular and loosely coupled design, enabling easier maintenance, and facilitating seamless integration of new features or changes.
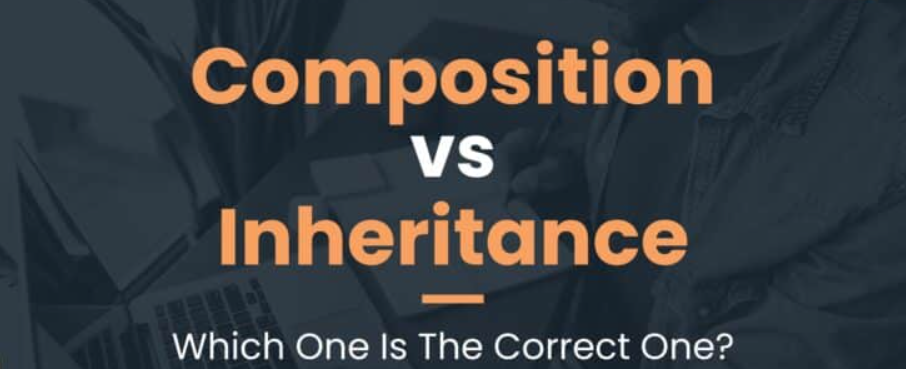
By weighing the principles, advantages, and considerations of both techniques, developers can make informed decisions tailored to the specific requirements of their software projects. Whether opting for inheritance for its code reuse benefits or choosing composition for its flexibility and testability advantages, understanding these concepts empowers developers to architect more resilient, scalable, and maintainable software solutions.
In essence, the choice between inheritance and composition represents a fundamental aspect of software design, influencing not only the structure of the codebase but also its long-term viability and adaptability. Armed with this knowledge, developers can navigate the complexities of OOP with confidence, striving toward the creation of robust and future-proof software systems.