Data hiding, also known as information hiding, is a fundamental concept in object-oriented programming (OOP) aimed at safeguarding the integrity and security of data within a program. At its core, data hiding involves concealing the internal details of an object from external entities, thereby preventing unauthorized access and manipulation. The primary objective of data hiding is to restrict access to certain data elements within a class, ensuring that only designated methods can interact with them. By encapsulating sensitive data within a class and exposing it only through controlled interfaces, data hiding mitigates the risk of inadvertent modifications or breaches of data integrity.
In practical terms, data hiding involves selectively shielding specific data components of a program from direct access by external objects or methods. When an attempt is made to access hidden data without proper authorization, the program will typically return an error, signaling the violation of data-hiding principles.
By enforcing data hiding, programmers can maintain a clear separation between a class’s internal implementation details and its external interface, promoting modularity, encapsulation, and abstraction. This separation of concerns enhances the reliability, security, and maintainability of the codebase, as changes to the internal implementation can be made without impacting external dependencies.
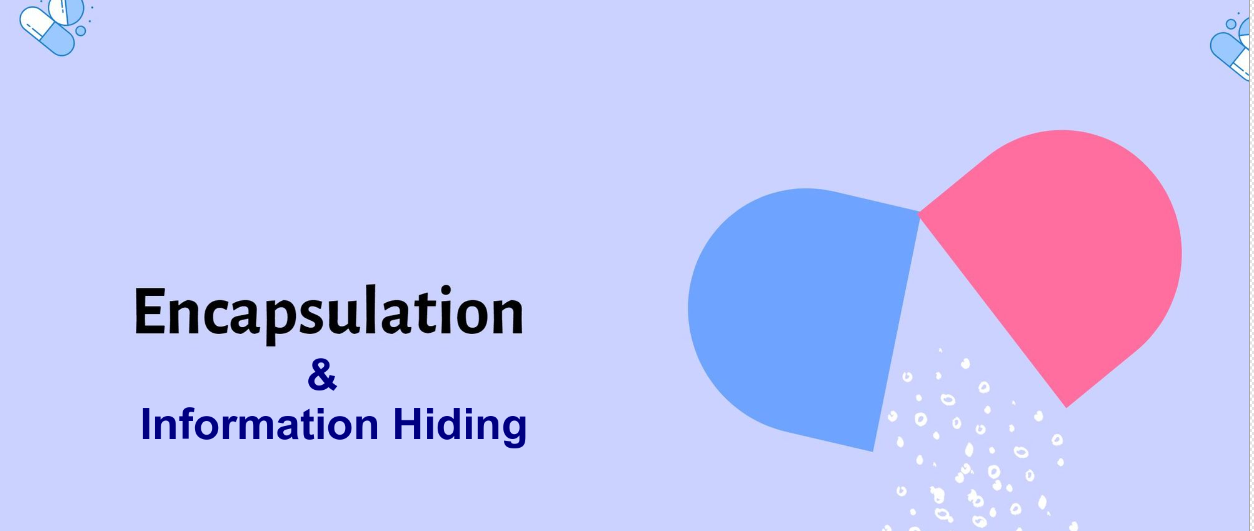
Furthermore, data hiding enables developers to control the visibility and accessibility of data members, ensuring that only essential information is exposed to external components while keeping implementation details hidden. This reduces the risk of unintended dependencies and enhances the overall robustness of the software system.
In essence, data hiding serves as a protective barrier that shields sensitive data from unauthorized access and manipulation, fostering a secure and reliable foundation for object-oriented programs. By adhering to data-hiding principles, developers can build software systems that prioritize data integrity, security, and encapsulation, ultimately leading to more resilient and maintainable codebases.
Understanding Encapsulation
Encapsulation is a concept in object-oriented programming, involving the packaging of data and functions from a real-world entity into a programmable enclosure, typically classes/objects. The data represents attributes and properties, while the functions represent possible behaviors and operations.
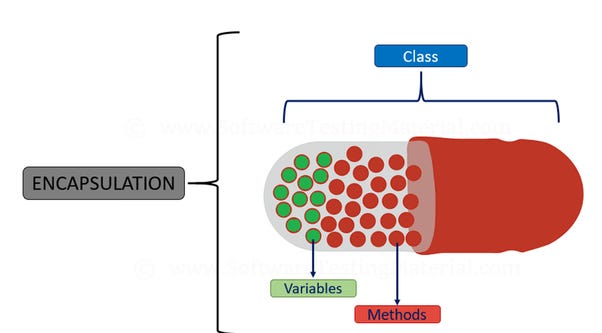
One of the fundamental ideas of object-oriented programming, or OOP, is encapsulation, which is the grouping of variables and functions into separate entities. The entity gains significance from this coupling, which also makes it semantically similar to actual things. By offering abstracted programming and interaction interfaces and shielding the internal state of the item from outside access and alteration, encapsulation conceals complexity. This keeps code errors from leaking into other areas of the program, which is essential for those who handle sensitive data.
Let’s use encapsulation to enhance the design in the last portion. The information and the functions that work with it can be combined into a single class:
class BookEncapsulation
{
public String author;
public int isbn;
public BookEncapsulation(String author, int isbn) {
this.author = author;
this.isbn = isbn;
}
public String getBookDetails() {
return "author name: " + author + " ISBN: " + isbn;
}
}
Here, we improve the code by bundling the implementation with the class. This makes the code modular. Clients can easily invoke the getBookDetails() methods without having much knowledge of its implementation.
Let’s see a unit test to invoke getBookDetails():
@Test
void givenEncapsulatedClass_whenDataIsNotHidden_thenReturnResult() {
BookEncapsulation myBook = new BookEncapsulation("J.K Rowlings", 67890);
String result = myBook.getBookDetails();
assertEquals("author name: " + myBook.author + " ISBN: " + myBook.isbn, result);
}
Although encapsulation makes the code better because the fields utilize a public access modifier, external classes can alter the Book data. There are no hard and fast guidelines for encapsulation regarding the usage of access modifiers. The public, private, and secured access modifiers are available for use.
Encapsulation also aids in adding functionality to a class without interfering with external code that uses the class. Now let’s add an id to the BookEncapsulation class:
// ...
public int id = 1;
public BookEncapsulation(String author, int isbn) {
this.author = author;
this.isbn = isbn;
}
public String getBookDetails() {
return "author id: " + id + " author name: " + author + " ISBN: " + isbn;
}
// ...
However, by using the concept of “information hiding,” we may tighten encapsulation. Defensive programming requires more than just encapsulation. To stop unauthorized data alteration, we must use information hiding along with encapsulation.
Information Hiding
Encapsulation and information hiding are closely related to software design principles. It highlights the necessity of keeping a class’s or module’s implementation details hidden from the outside world and just exposing necessary interfaces. This method encourages code modularity, safeguards sensitive data, and aids in managing system complexity. For software to be flexible, maintainable, and secure, information hiding is essential. Let’s take a closer look at the idea of information masking and see how crucial it is to software development.
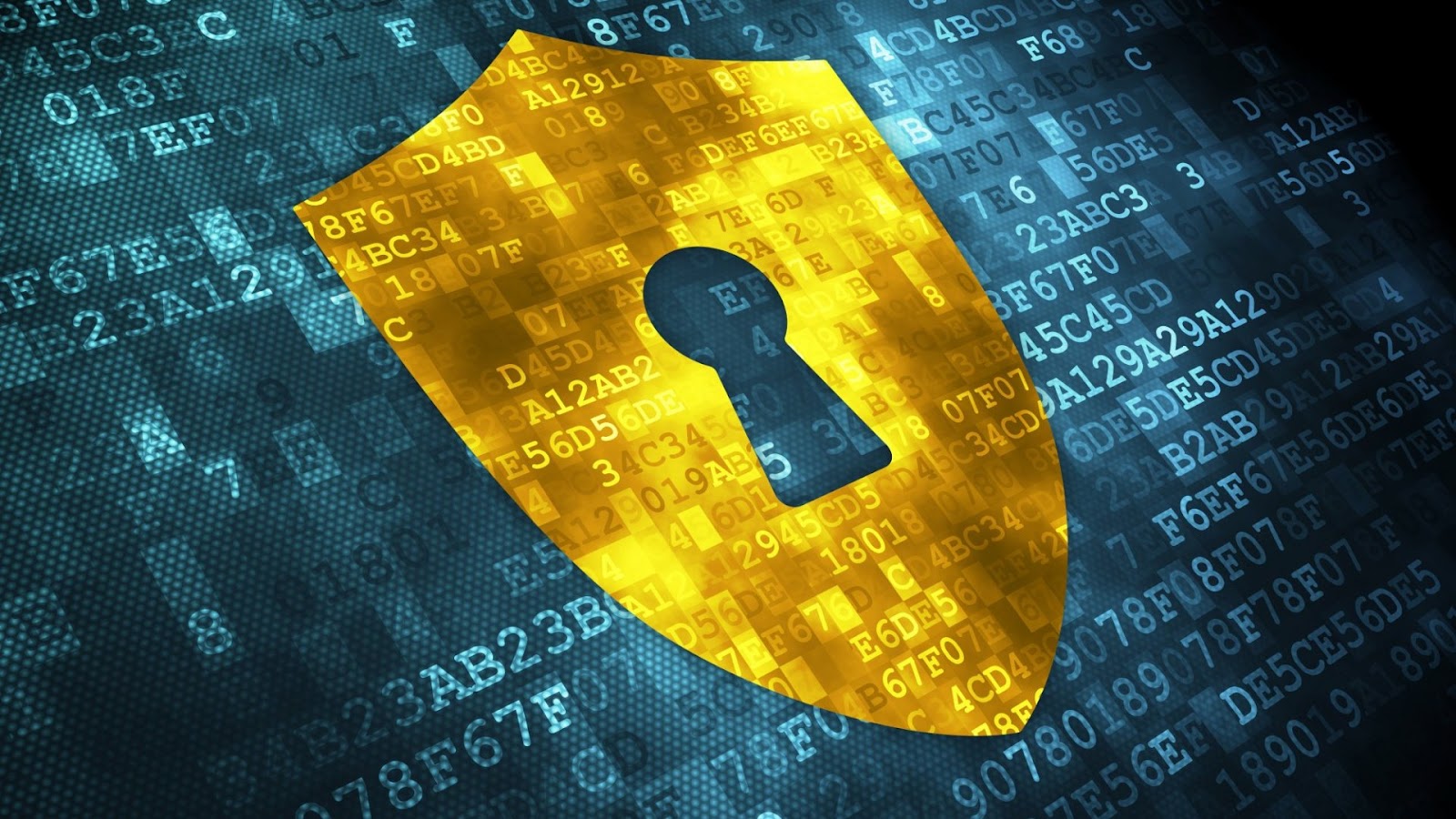
A programming approach called “information hiding” tries to prohibit direct changes to a class’s data. It also offers stringent guidelines for gaining access to and changing class data. Keeping design implementations—especially those that are prone to change—hidden from the customer also helps. Additionally, modular code is ensured when information hiding is combined with encapsulation.
Now let’s add information hiding to the enclosed class to make it better:
class BookInformationHiding {
private String author;
private int isbn;
private int id = 1;
public BookInformationHiding(String author, int isbn) {
setAuthor(author);
setIsbn(isbn);
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getIsbn() {
return isbn;
}
public void setIsbn(int isbn) {
this.isbn = isbn;
}
public String getBookDetails() {
return "author id: " + id + " author name: " + author + " ISBN: " + isbn;
}
}
In this case, we limit access to and modifications to fields from an external class using the private access modifier. In addition, we construct getters and setters to control the field’s access and modification capabilities. Information hiding is accomplished by using a private access modifier instead of encapsulation without information hiding, where any access modifier can be used. This limits access to the class’s internal fields alone. It is more resilient since a client code cannot access the fields directly.
A unit test for the updated class is shown below:
@Test
void givenEncapsulatedClass_whenDataIsHidden_thenReturnResult() {
BookInformationHiding myBook = new BookInformationHiding("J.K Rowlings", 67890);
String result = myBook.getBookDetails();
assertEquals("author id: " + 1 + " author name: " + myBook.getAuthor() + " ISBN: " + myBook.getIsbn(), result);
}
We can also establish stringent guidelines for data modification. For example, by changing the setter, we may prevent a negative ISBN:
public void setIsbn(int isbn) {
if (isbn < 0) {
throw new IllegalArgumentException("ISBN can't be negative");
}
this.isbn = isbn;
}
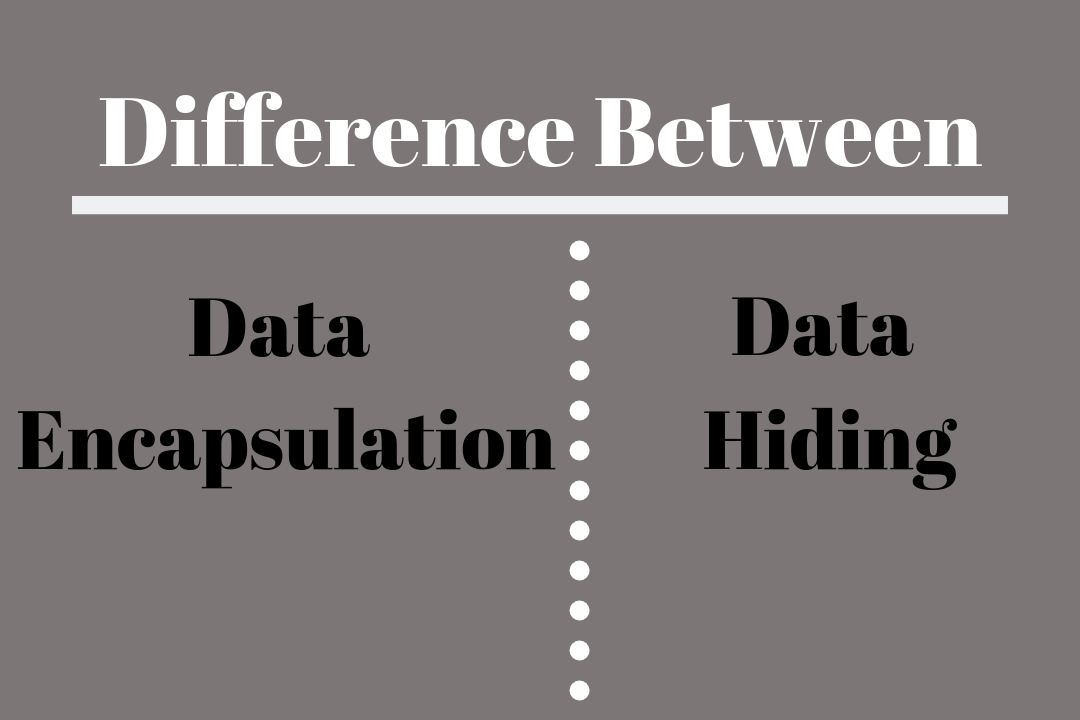
Encapsulation vs. Data Hiding
Data Hiding | Encapsulation |
By limiting deliberate or unintentional modifications, data hiding preserves object integrity and grants class members exclusive access to data. | Access to class members and maintain object integrity by blocking deliberate or accidental modifications. One OOP technique called encapsulation groups together the data and the procedures that manipulate it. |
Data hiding focuses on securing the data while hiding the complexity. | Encapsulation focuses on hiding the complexity of the system. |
Data hiding is a data-protecting process. | Encapsulation is a method of achieving data hiding. |
Data hiding uses a private access modifier. | Encapsulation uses private, protected, and public access modifiers. |
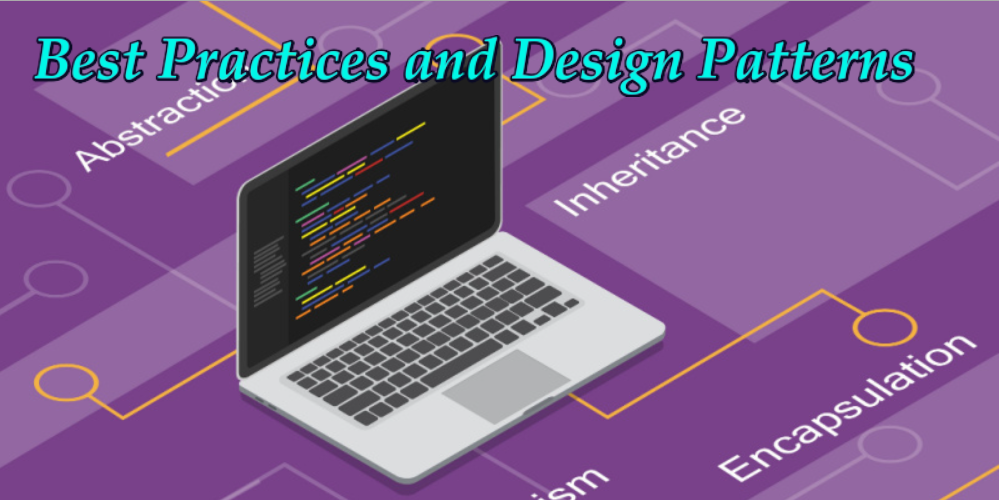
Best Practices and Design Patterns
Using design patterns and best practices in object-oriented programming (OOP) is crucial to developing scalable, extendable, and maintainable software. These best practices and patterns give developers tested methods and blueprints to tackle typical design problems, encourage code reuse, and improve the general caliber of the codebase. Let’s examine some essential OOP design patterns and recommended practices in more detail.
Now here we discuss the Best Practices for Encapsulation and Information Hiding:
Define Clear and Concise Interfaces:
- Create design classes with well-defined interfaces that make it obvious which attributes and public methods are accessible to outside clients.
- To encourage simplicity and ease of use, keep interfaces simple and concentrate on the most important features.
Use Access Modifiers to Enforce Encapsulation:
- Use access modifiers to manage class members’ visibility and accessibility, such as private, protected, and public.
- Protect sensitive information by designating it as private and granting restricted access via public APIs (getters and setters).
Minimize Exposure of Implementation Details:
- Reduce dependencies and encourage loose coupling between components by keeping implementation details hidden from external clients.
- Provide only the essential interfaces and decouple the implementation code to promote flexibility and maintainability.
Encapsulate Complex Behavior Within Class Methods:
- To encourage code structure and maintainability, encapsulate complicated operations and algorithms into class methods.
- Encapsulation may be used to provide a clear division of responsibilities within the codebase and to group relevant functions together.
Now the preferable Design Patterns Promoting Encapsulation and Information Hiding:
Singleton Pattern:
- A class is guaranteed to have a single instance according to the Singleton pattern, which also offers a global access point to that instance.
- When several components of the program need to access a single common resource, this approach might be helpful.
Factory Pattern:
- Clients may utilize interfaces without having to deal with the actual implementation since the Factory pattern wraps the process of creating objects.
- Factories enable decoupling and flexibility in system architecture by abstracting away the object production process.
Strategy Pattern:
- The Strategy pattern offers flexibility and extension by encapsulating algorithms and enabling runtime selection.
- Strategies allow clients to move between alternative algorithms without changing the client code since they contain changes in behavior.
Applying SOLID Principles:
Single Responsibility Principle (SRP):
- Classes ought to be responsible for just one thing, a single part of the operation of the system.
- This approach makes sure that classes are coherent and focused, which encourages testability, maintainability, and modularity.
Open/Closed Principle (OCP):
- Software entities ought to be closed to alteration yet open to expansion.
- Classes may be expanded without changing the current code thanks to encapsulation and abstraction, which encourages code reuse and reduces the possibility of introducing defects.
Liskov Substitution Principle (LSP):
- Subclasses need to be able to replace their base classes without affecting the program’s validity.
- LSP compliance is mostly dependent on encapsulation and information hiding, which make sure that subclasses follow the contracts set out by their base classes.
Interface Segregation Principle (ISP):
- Subclasses need to be able to replace their base classes without affecting the program’s validity.
- LSP compliance is mostly dependent on encapsulation and information hiding, which make sure that subclasses follow the contracts set out by their base classes.
To sum up, information hiding and encapsulation are the cornerstones of object-oriented programming (OOP), offering crucial tools for building software that is safe, scalable, and extensible. Developers may accomplish several crucial goals by encapsulating data and operations into classes and hiding implementation details from outside entities.
Encapsulation and information hiding are two key techniques in software development. Encapsulation enhances modularity by encapsulating data within classes, facilitating code reuse, and enhancing security by restricting access to sensitive data and implementation details. Both methods contribute to the overall security of a software system.
Moreover, information masking and encapsulation support software systems’ scalability and flexibility. The system’s functionality may be readily expanded and changed by developers by severing the internal implementation from external dependencies. Internal details may be modified without impacting outward components, allowing the system to grow and adapt to new needs with ease.
The concepts of information hiding and encapsulation will always be essential as software systems develop and adapt to new needs and situations. Their foundation is strong enough to support the development of long-lasting, adaptable, and robust applications. Developers may create software systems that not only satisfy users’ and stakeholders’ demands today but also successfully predict and handle opportunities and difficulties down the road by following these guidelines.