Two essential methods in the field of object-oriented programming (OOP) that let programmers create adaptable and extendable codebases are method overloading and method overriding. Although these concepts are similar in that they have several methods with the same name, they have different functions and behave differently. We will travel through the differences between overloading and overriding methods in this in-depth tutorial, illuminating their use cases, advantages, and suggested practices. Come along as we explore the nuances of these fundamental OOP concepts to help you gain a deeper comprehension and boost your coding abilities.
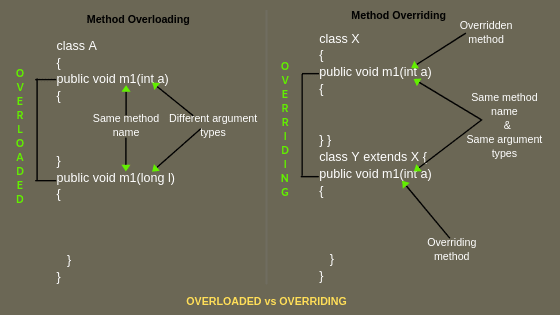
Methods in Java
To carry out particular actions or processes with an object, methods are employed. When a Java program is launched, the main() method is the first one the compiler calls.
The method name and argument list make up a method’s signature. For instance, the signature for the method “addition” may be “int addition(int a, int b)” and it would execute an addition operation.
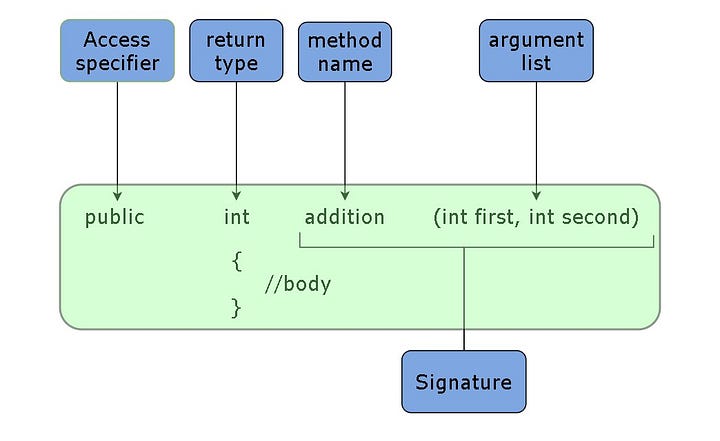
What is Method Overloading?
We can have numerous methods with the same name but distinct argument lists in Java thanks to method overloading. This static polymorphism, also known as a compile-time polymorphism, enables us to carry out a single operation in several ways. Another name for this is early binding. We may generate distinct implementations of a method depending on the inputs supplied by utilizing method overloading. Thus, this will enable us to apply the same method name to several tasks.
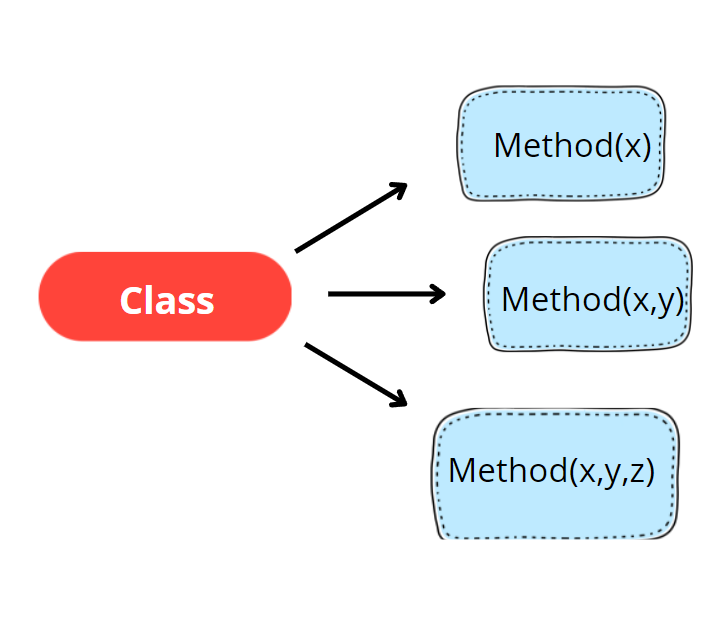
For example, consider the addition method again, but this time you are unsure whether you will have to add three or two integers. So we create two methods with the same name, but one with two integer parameters and the other with three integer parameters.
Here is an example of method overloading
public class Calculator
{
// Method to add two integers
public int addition (int a, int b) f
return a + b;
// Method to add three integers
public int addition (int a, int b, int c)
{
return a + b + c;
}
}
The compiler determines which version of a method to call by looking up the method’s name and parameter list when the method is called. The compiler will select the appropriate version of a method depending on the arguments supplied to it if there are several methods with the same name but distinct argument lists.
Calculator calc = new Calculator);
// calls the first version of the method
int sum = calc. addition (1, 2);
// calls the second version of the method
int sum2 = calc. addition (1, 2, 3);
If we call calc.addition(1, 2) in the example above, the compiler will search for a method named “addition” that accepts two integer parameters. It will locate the original “addition” method and invoke it with the supplied parameters. Similarly, the compiler will locate the second iteration of the “addition” function and call it with the supplied parameters if we call calc. addition(1, 2, 3).
Understanding Method Overriding
Method overriding is a fundamental concept in object-oriented programming (OOP), providing programmers with a powerful tool to write more flexible and dynamic code. This fundamental approach allows subclasses to override the behavior of methods that they inherit from their superclasses, giving them the ability to customize functionality to meet particular needs without compromising the coherence of their code.
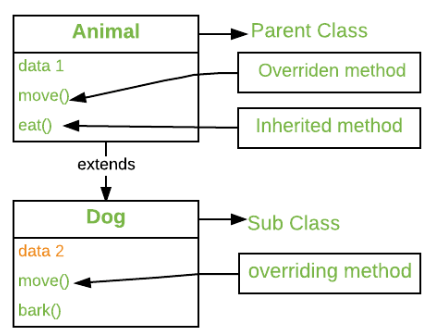
Method overriding is essentially the ability of a subclass to provide a new implementation of a method that is already defined in its superclass. This crucial feature allows programmers to alter how methods behave within subclasses, overriding the default implementations that are carried over from parent classes. As a result, the subclass’s customized implementation is executed when an instance calls the overridden method, allowing for fine-grained control over method behavior and fostering code modularity and extensibility.
public class Shape
{
public virtual void Draw()
{
Console.WriteLine("Drawing a shape...");
}
}
public class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a circle...");
}
}
public class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a rectangle...");
}
}
Shape shape1 = new Circle();
Shape shape2 = new Rectangle();
shape1.Draw(); // Outputs "Drawing a circle..."
shape2.Draw(); // Outputs "Drawing a rectangle..."
The derived classes Circle and Rectangle override the virtual function Draw() of the base class Shape in this example of code. The corresponding overridden implementations are used when we make instances of the derived classes and use the Draw() function. This demonstrates how method overriding may be used to achieve polymorphism.
Key Differences Between Method Overloading and Method Overriding
Two fundamental ideas in programming that frequently confound newcomers are method overloading and method overriding. These are methods for achieving flexibility and code reuse in object-oriented programming. Even if they are similar in several ways, they are distinguished from one another by clear distinctions. This article aims to clarify the differences and similarities between overloading and overriding methods, highlighting their unique features and applications.
Method Overloading | Method Overriding |
takes place in a single class. | transpires in a parent-child class. |
involves several procedures with distinct parameters but the same name. | involves a method that has the same name and arguments in the parent class and a method in the child class. |
Static binding means that a decision is determined at build time. | Runtime decision-making occurs (dynamic binding). |
Either the same or a different return type is possible. | The return type in the child class needs to match or be a subtype of that type. |
There is no need for a link between the overloaded methods. | requires that the parent and child classes have an inheritance connection. |
Methods with distinct capabilities might have the same name thanks to overloading. | Child classes can offer customized implementations for inherited methods by using overriding. |
The kind, sequence, and quantity of method parameters can change. | In both parent and child classes, the type, order, and number of method arguments must match. |
Within a single class, method overloading is accomplished by altering the method signature. | Using the @Override annotation in the child class allows for method overriding. |
Advantages and Best Practices
There are clear benefits to both overloading and overriding methods, which help to create and maintain high-caliber software. Leveraging these features successfully requires understanding these benefits and adhering to best practices. Let’s examine method overloading and overriding in more detail, along with some best practices:
Advantages of Method Overloading
The following benefits of method overloading improve the readability, flexibility, and maintainability of code:
- Improved Readability: Method overloading improves readability of the code by offering numerous methods with the same name but distinct argument lists. The desired functionality may be conveyed by developers through the choice of intuitive method names, which facilitate understanding and maintenance of the code.
- Enhanced Code Reusability: By allowing writers to describe common behavior in a single function and give variants for various input sources, method overloading encourages code reusability. By doing this, duplication is decreased and a modular codebase—wherein similar features may be applied to many contexts—is encouraged.
- Flexibility in Handling Different Input Scenarios: Developers may handle many input conditions using method overloading without overcrowding the code with conditional statements. Developers can offer flexibility in method invocation based on the arguments given by creating various method signatures, each suited to a certain input circumstance.
- Simplified API Design: Method overloading offers a clear and user-friendly interface for dealing with objects, which streamlines the design of APIs. To reduce the cognitive burden for API consumers, developers can utilize method overloading to provide a consistent interface with overloaded methods instead of providing several methods with different names.
Advantages of Method Overriding
To provide customization, polymorphism, and adherence to object-oriented design principles, method overriding has the following benefits:
- Polymorphic Behavior and Runtime Flexibility: Polymorphic behavior, in which objects of various kinds can react differently to the same method call, is made possible via method overriding. This encourages dynamic behavior and runtime flexibility, enabling programmers to create code that adjusts to evolving runtime parameters and contexts.
- Adherence to OOP Principles: Method overriding makes it easier to follow important OOP and LSP (Liskov Substitution Principle) guidelines for object-oriented design. Method overriding encourages code extension and guarantees that subclasses may be used interchangeably with their superclasses without compromising the program’s validity by enabling subclasses to extend and alter the behavior of their superclasses.
- Customization and Specialization of Behavior: Subclasses can offer unique implementations of methods they inherited from their superclasses by using method overriding. This allows for the specialization and customization of behavior, allowing subclasses to inherit common functionality from the superclass and modify it to suit their own needs.
Best Practices for Method Overloading and Method Overriding
Developers should follow these recommended practices to make the most of method overloading and overriding:
- Choose Meaningful Method Names and Parameter Lists: Choose names for your methods that correctly represent the desired functionality. When invoking a method, use parameter lists that offer consistency and clarity.
- Ensure Consistency and Clarity in Method Behavior: Ensure that the behavior of overloaded or overridden methods remains consistent. Steer clear of unanticipated side effects or behavioral changes that might perplex code users.
- Document Method Contracts and Expected Behaviors: Preconditions, postconditions, and invariants should all be included in method contracts to give instructions on how to use them and what actions are expected of you. This documentation makes it easier for developers to use and maintain each method properly by outlining its goals and limitations.
Developers may efficiently utilize the advantages of method overloading and overriding by adhering to these best practices, which will result in codebases that are easier to understand, adapt, and maintain. Furthermore, following best practices encourages method design that is clear and consistent, which facilitates developer collaboration and code maintenance over time.
In conclusion, object-oriented programming (OOP) has strong characteristics like method overloading and overriding that let programmers design adaptable, extendable, and maintainable software. Although both strategies entail naming several procedures under the same heading, they have different functions and provide unique benefits.
Method overloading and method overriding are two techniques used in software development. Method overloading improves code readability and flexibility by providing multiple methods with the same name but different parameter lists, while method overriding allows for customization and adherence to object-oriented design principles. Understanding these techniques helps developers design robust, adaptable, and scalable codebases. They are used in various domains, such as banking systems and web frameworks.
In conclusion, developers need to have both overloading and overriding in their toolbox to create codebases that are beautiful, extendable, and manageable. Through the mastery of these approaches and their prudent use in projects, developers may foster innovation in software engineering and open up new avenues for exploration.