There are solutions for many file processing chores in this repetitive and routine Python work of file handling. An example of one of these situations is when you have to import a module from a certain location on your system. Python has the necessary capabilities available to import modules dynamically in cases where their entire path is reachable. Python code demonstrates the strength of flexibility and modularity thanks to these features. In this post, we examine many approaches for importing Python modules with their whole route; each approach has a special benefit for a range of scenarios. Here, an effort is being made to effectively walk you through each technique using practical code samples and step-by-step explanations. You will be able to dynamically import modules from any desired location by the conclusion of this tutorial. Now let’s explore the realm of importing Python modules by utilizing their whole route.
Dynamic Module Loading
In Python, the import statement is usually used to import modules at the start of a script or module. The import module may, however, be decided at runtime or its location may only be dynamically known in certain circumstances. Dynamic module loading becomes crucial in these situations.
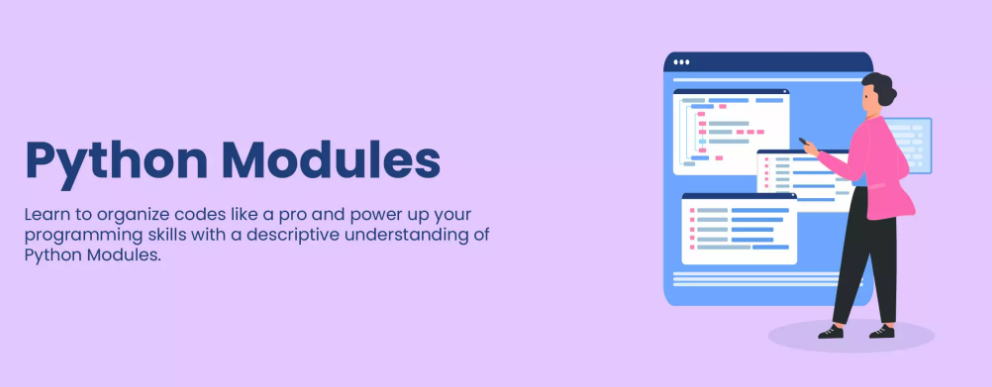
Developers can import modules based on runtime conditions, such as user input, configuration settings, or outside causes, by using dynamic module loading. Applications may adjust to shifting needs and conditions thanks to this flexibility, which eliminates the need for hardcoded import lines.
Understanding Importing Python Modules by Full Path
To start, we try to understand what full path import of Python modules means. The discussion of the code samples and their justifications follows. The sys.path list specifies the directories in which the Python interpreter looks for modules. However, by adjusting the sys.path or utilizing built-in functions and libraries, modules may be dynamically loaded from any place.
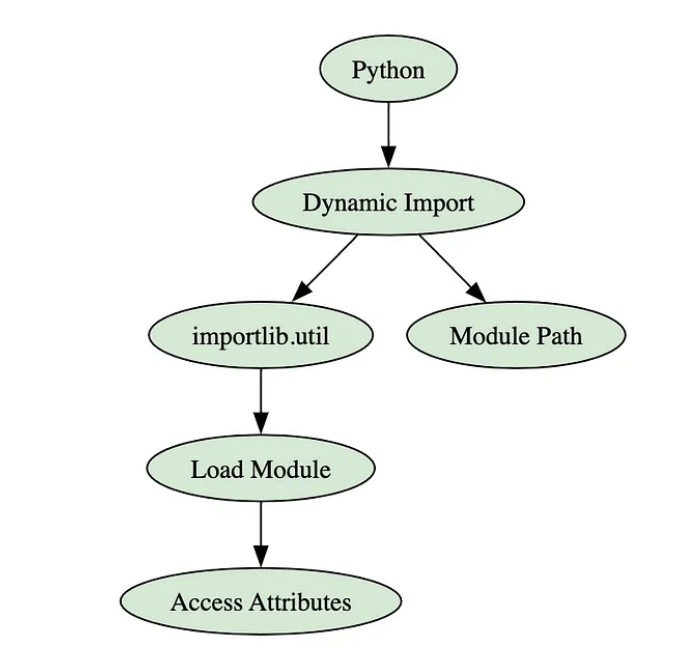
Examining how to use the importlib.util module, create a spec from the file location, load the module from the spec, and then access the module’s attributes to enable dynamic and flexible module management in Python applications is the first step towards dynamically importing a module using its full path in Python.
Different Ways to Import Python Files
The module can be imported using its whole path in several ways. The following are some frequently used techniques that we are employing in this instance to import Python files.
- Using importlib Package
- Using exec() Function
- Using importlib.util.spec_from_file_location()
- Using sys.path.append() Function
- Using SourceFileLoader Class
Using importlib Module
The importlib package offers a Python source code implementation of the import statement that is compatible with all Python interpreters. Users may use this to construct custom objects that assist them to tailor the import process to their own needs. One of the modules in this package that may be used to import the module from the specified directory is importlib.util.
import importlib
module_path = "/path/to/module.py"
module_name = "module"
module = importlib.import_module(module_name, module_path)
Dynamic Import with exec() Function
An alternative method of dynamic module loading is to dynamically run Python code using the exec() function. Although security considerations and the readability of the code make this method less desirable, it can be helpful in some situations.
module_path = "/path/to/module.py"
module_name = "module"
with open(module_path, 'r') as file:
module_code = file.read()
module = {}
exec(module_code, module)
Here, the exec() method is used to perform a dynamic execution of the module file once the contents have been read using the open() function. The module dictionary contains the generated module.
Importing Modules Using importlib.util
Additional utilities for working with modules and their specifications are provided by the importlib.util module. spec_from_file_location() is one such method that takes a file path and outputs a module specification.
Dynamically load Python modules by path, leveraging importlib for advanced, flexible module management.
import importlib.util
import sys
# Define the full path of the module
module_path = '/Users/user_name/notebooks/hello.py'
# Load the module from the given path
spec = importlib.util.spec_from_file_location('module_name', module_path)
module = importlib.util.module_from_spec(spec)
spec.loader.exec_module(module)
# Add the module to sys.modules
sys.modules['module_name'] = module
Dynamically Importing Modules from Zip Archives
Python further facilitates the direct import of modules from zip archives, hence enabling more effective Python code distribution. This capability can be very helpful when deploying apps with several dependencies and modules.
import sys
import zipfile
with zipfile.ZipFile("modules.zip", 'r') as zip_ref:
zip_ref.extractall("/path/to/extracted")
sys.path.insert(0, "/path/to/extracted")
import module
In this example, sys.path.insert() is used to add the contents of the modules.zip zip archive to the Python path once they have been extracted to a temporary directory. Lastly, the normal import statement may be used to import the appropriate module.
We’ve covered a variety of methods in this tutorial for dynamically importing modules in Python by using their whole paths. Python has a variety of options for dynamically loading modules during runtime, whether it be through the use of the importlib module or the exec() method.
Understanding dynamic module loading’s features and best practices may help developers create Python apps that are more scalable and flexible, able to change with changing needs and conditions.
I recommend delving deeper into the official Python documentation on module importing and the importlib module for more investigation. To learn more about the practical uses of dynamic module loading, investigate real-world examples and libraries that make use of it. These materials will improve your knowledge and skills related to dynamic module loading, enabling you to create more resilient and adaptable Python programs.