A few significant naming conventions in Python are predicated on the use of the underscore (_) character, either single or double. By following these guidelines, you may prevent name conflicts, build safe classes for subclassing, and distinguish between public and non-public names in APIs, among other things.
You may produce code that appears consistent and Pythonic to other Python writers by adhering to and respecting these standards. This ability comes in particularly handy when you’re building code that other developers will be interacting with.
Single and Double Underscores
The norm in Python is to use a single or double underscore before an object name has certain connotations. These naming standards prevent naming conflicts, preserve code clarity, and provide certain objects with unique functionality.
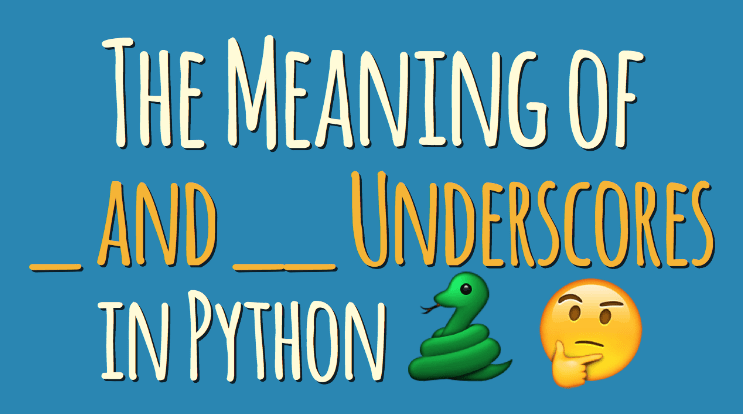
In Python, underscores can be used as prefixes or suffixes. However, they have different functions and affect the way the Python interpreter handles the objects in various ways.
Single Underscore Prefix: Convention for Privacy
An object is private and meant for internal usage within its class or module when a single underscore appears before its name. This practice, which alerts other developers that an object should not be used outside of its module or class, is not mandatory.
class MyClass:
def __init__(self):
self._private_variable = 42
def _private_method(self):
pass
The _private_method and _private_variable in this example are meant only for internal usage by the MyClass class. The single underscore prefix acts as a visual cue to other developers that these objects are private and should be handled as such, even if they may still be accessed from outside the class.
Double Underscore Prefix: Name Mangling
“Name mangling” occurs when an object name inside a class declaration is preceded by a double underscore __ prefix. Python has a method called “name mangling” that allows you to change an object’s name to make it stand out inside its class.
For example:
class MyClass:
def __init__(self):
self.__mangled_variable = 42
def __mangled_method(self):
pass
To make them stand out within the MyClass namespace, __mangled_variable and __mangled_method in this example are prefixed with _MyClass, a process known as name mangling. This lessens the possibility of unintentional name conflicts with external codes and subclasses.
Double Underscore Prefix and Suffix: Dunder Methods
Double underscores appear in the names of several unique Python methods called “dunder” methods, or “double underscore” methods. Because these methods give objects unique behavior and functionality when they are called by the Python interpreter, they are often referred to as “magic” methods.
A few frequently used dunder methods include __iter__, __add__, __str__, __repr__, and __init__, among many more. Python calls these methods automatically in reaction to certain actions or behaviors on objects, giving developers the ability to specify unique behaviors for their classes.
class MyClass:
def __init__(self, value):
self.value = value
def __repr__(self):
return f"MyClass({self.value})"
def __add__(self, other):
return MyClass(self.value + other.value)
obj1 = MyClass(10)
obj2 = MyClass(20)
print(obj1 + obj2)
Output: MyClass(30)
The __add__ dunder method in this example enables specific behavior for addition operations on these objects by allowing instances of MyClass to be added together using the + operator.
Single Underscore Suffix: Used for Variable Names
To prevent naming conflicts with Python keywords or built-in methods, Python developers not only use underscores as prefixes but use a single underscore _ as a suffix in variable names.
class_ = MyClass() # Using a single underscore suffix to avoid conflict with "class" keyword
Variable names that include a single underscore suffix by convention indicate to other developers that the variable has a special function and should be handled carefully to prevent abuse or unintentional reassignment.
Finally, it can be said that the careful use of single and double underscores in Python object names accomplishes many goals, including indicating secrecy, causing name mangling, and defining unique behavior using dunder methods. Understanding and following the standards associated with underscores will help you write more organized, understandable, and maintainable Python code.
I suggest reading through the official Python documentation and community-driven resources that concentrate on object-oriented programming concepts and coding style standards if you want to learn more about Python naming conventions and best practices. These resources provide insightful analysis and helpful advice to improve your Python programming skills and codebase quality.