Since Python is a dynamically typed language, variables can contain any kind of value, and the type of a variable is decided upon at runtime. In some circumstances, though, you might need to verify an object’s type in your Python code. Knowing the standard method for checking for types in Python is crucial, regardless of whether you’re using it for runtime type checks, user input validation, or guaranteeing interoperability across various components of your codebase.
We’ll look at a variety of methods for type-checking in Python in this in-depth article, including how to use third-party libraries, built-in functions, and best practices for managing type-checking in Python projects.
Type Checking in Python
The practice of confirming that an item complies with a specific type or class is known as type checking. Type checking in Python helps make sure your code works as intended, finding mistakes early in the development process, and making your code easier to understand and maintain.
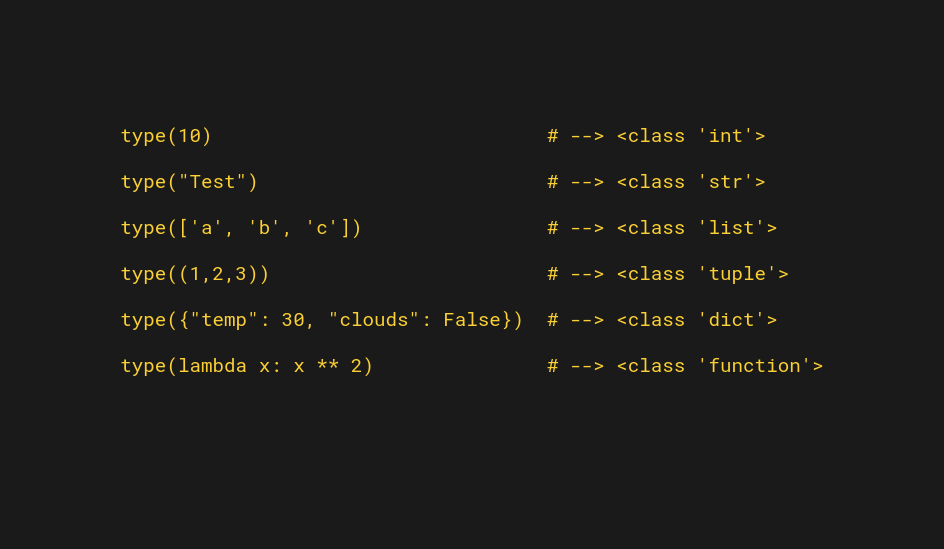
Type checking is accomplished by many built-in Python functions and techniques, each with specific advantages and applications. Writing solid and dependable Python code requires an understanding of these strategies and an understanding of when to apply them.
Using the “type()” Function
When saving data items in a variable in Python, the data type of the variable is not explicitly specified. Therefore, we utilize Python’s built-in type() method to determine the kind of data stored in a variable. A built-in Python method called type() is used to output the type of function parameters according to the arguments that are supplied to it. There are two distinct ways to send parameters to the type() function, or, in other words, there are two types of type() functions.
The syntax is as follows –
type(object)
type(name, bases, dict)
where,
name = name of the class, which becomes ___name___ attribute
bases = tuple from which current class is derived, becomes ___bases___ attribute
dict = a dictionary which specifies the namespaces for the class later becomes ___dict___ attribute
The function’s sole parameter is accepted by the first approach. On the other hand, the second approach takes three parameters.
The type(object) method returns the object’s type if you offer it just one parameter. The type(name, bases, dict) method builds a class dynamically and returns a new type object when three parameters are passed to it.
Example:1
a = 10
b = 10.5
c = True
d = 1 + 5j
print(type(a))
print(type(b))
print(type(c))
print(type(d))
Output:
<class ‘int’>
<class ‘float’>
<class ‘bool’>
<class ‘complex’>
Example:2
my_str = 'Hello World!'
my_list = [1, 2, 3, 4, 5]
my_tuple = (10, 'Hello', 45, 'Hi')
print(type(my_str))
print(type(my_list))
print(type(my_tuple))
Output:
<class ‘str’>
<class ‘list’>
<class ‘tuple’>
Using The “isinstance()” Function
Python has the isinstance() method, which lets you determine if an object is an instance of a specific class or type, to overcome some of the drawbacks of the type() function.
x = 42
print(isinstance(x, int))
s = "Hello, World!"
print(isinstance(s, str))
l = [1, 2, 3]
print(isinstance(l, list))
Output:
True
True
True
Example: 2
In this example, we will use Python to determine an object’s class, or whether it is an instance of a class or a derived class.
# declaring classes
class gfg1:
a = 10
# inherited class
class gfg2(gfg1):
string = 'GeeksforGeeks'
# initializing objects
obj1 = gfg1()
obj2 = gfg2()
# checking instances
print("Is obj1 instance of gfg1? : " + str(isinstance(obj1, gfg1)))
print("Is obj2 instance of gfg2? : " + str(isinstance(obj2, gfg2)))
print("Is obj1 instance of gfg2? : " + str(isinstance(obj1, gfg2)))
# check inheritance case
# return true
print("Is obj2 instance of gfg1? : " + str(isinstance(obj2, gfg1)))
Output:
Is obj1 instance of gfg1? : True
Is obj2 instance of gfg2? : True
Is obj1 instance of gfg2? : False
Is obj2 instance of gfg1? : True
Because it considers inheritance, the isinstance() method is more versatile than type(). If the object belongs to the class or any of its subclasses that is given, it returns True. For testing object types in polymorphic code, where objects could belong to several subclasses of a same base class, this makes it very helpful.
Using issubclass() Function for Checking Class Hierarchy
A built-in method in Python called issubclass() may be used to determine if a class is a subclass of another class or not. If the supplied class is a subclass of the provided class, this method returns True; otherwise, it returns False. Using issubclass(), we may find out whether a Python class is an inheritance.
We’ll examine how to verify the built-in functions’ subclasses in this example.
print("Float is the subclass of str:", issubclass(float,str))
print("Bool is the subclass of int:", issubclass(bool,int))
print("int is the subclass of float:",issubclass(int,float))
import collections
print('collections.defaultdict is the subclass of dict: ', issubclass(collections.defaultdict, dict))
Output:
Float is the subclass of str: False
Bool is the subclass of int: True
int is the subclass of float: False
collections.defaultdict is the subclass of dict: True
Type Annotations and the typing Module
Developers may now add type information to function signatures and variable declarations to give more context and explanation for their code, thanks to the arrival of type hints in Python 3.5 and the typing module.
from typing import List
def process_numbers(numbers: List[int]) -> List[int]:
return [n * 2 for n in numbers]
By defining the anticipated types of function arguments and return values, type annotations facilitate understanding and verification of the intended behavior of your code by other developers and tools such as static type checkers.
Third-Party Libraries for Type Checking
Several third-party libraries offer extra features and capabilities for type-checking in Python applications, even though Python’s built-in type-checking tools are rather strong. Several well-liked choices consist of:
- mypy: A static type checker for Python that analyzes your code statically to identify mistakes and inconsistencies related to types. It also supports type annotations.
- Pydantic: A data validation package that enables runtime type checking and input data validation. It lets you design data schemas using Python data classes.
- pytype: Python code static analyzer that does type inference and looks for mistakes related to types, such as missing type annotations, incompatible types, and other type-related problems.
Best Practices for Type Checking in Python
It’s critical to adhere to recommended practices when type-checking Python code to make sure it’s comprehensible, efficient, and maintainable. Among the recommended procedures to bear in mind are:
- To give your code more context and description, use type annotations.
- When runtime type checking is required, use isinstance(); but, do not rely too much on type checks.
- Write more reusable and adaptable code by avoiding explicit type tests and favoring polymorphism whenever feasible.
- To do static analysis of your code and identify type-related mistakes early in the development process, use static type checkers such as mypy.
Performance Considerations
Type checking has benefits for readability and maintainability of code, but you should also take performance into account while writing Python code. Type-checking often adds overhead to your code, particularly when it’s done frequently or in parts where performance is crucial. Take into account the following tactics to lessen type checking’s negative performance impact:
- Type annotations should only be used when necessary to give your code the relevant context and documentation.
- Steer clear of pointless or redundant type tests, particularly in areas of your code that are performance-critical or include tight loops.
- Instead of depending just on runtime type checks, do static analysis of your code using tools like mypy to identify type-related issues early in the development process.
To sum up, type checking is essential to Python development and helps to ensure that your codebase is correct, dependable, and maintainable. Having a firm understanding of the various methods and best practices for type checking in Python can help you write more readable, robust code that will be easier to debug and maintain in the long run.
I suggest reading through the official Python documentation on type hints and the typing module to gain a deeper grasp of type checking in Python. Furthermore, utilizing tools like mypy and Pedantic to explore tutorials and third-party materials on static type-checking can offer insightful advice on how to incorporate efficient type-checking techniques into your Python applications. By implementing these resources into your learning process, you will be well-equipped to maximize Python’s type-checking capabilities and improve the caliber of your code.