- Home
- /
- Programming
- /
- Java
- /
- Page 2
Tutorial Category: Java
Java is a high-level, object-oriented programming language that is widely used for developing a variety of applications, including desktop software, mobile apps, and web applications. It was originally developed by Sun Microsystems in the mid-1990s and is now owned by Oracle Corporation.
Java is known for its “write once, run anywhere” philosophy, which means that code written in Java can be compiled and executed on any platform that supports the Java Virtual Machine (JVM), including Windows, macOS, Linux, and many others.
Some of the key features of Java include:
Object-oriented: Java is a fully object-oriented programming language, which means that everything in Java is an object.
Platform-independent: Java code can be compiled and run on any platform that supports the JVM.
Garbage collection: Java uses automatic garbage collection to automatically free up memory that is no longer in use.
Strong typing: Java is a statically typed language, which means that variables must be declared with a specific type.
Exception handling: Java includes built-in support for exception handling, which makes it easy to write code that can handle errors and unexpected events.
Rich API: Java includes a large library of built-in classes and APIs for performing common tasks, such as reading and writing files, networking, and user interface development.
Java is widely used in enterprise applications, including banking, healthcare, and insurance. It is also commonly used for developing mobile applications for the Android operating system.
Operators In Java
Comments In Java
Equality, Relational, and Conditional Operators In Java
Expressions, Statements, and Blocks
Intro To Loops In Java
Java Basics - First Look At Java Methods
Introduction To Object Oriented Programming In Java
A Sneak Peek Of Objects in Java
Class In Object Oriented Programming
A More In-Depth Inspection Of Class In Java
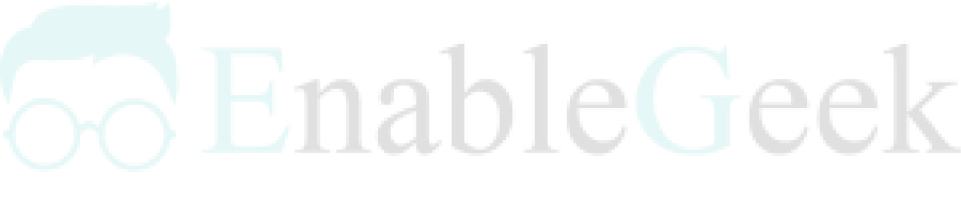
Check Our Ebook for This Online Course
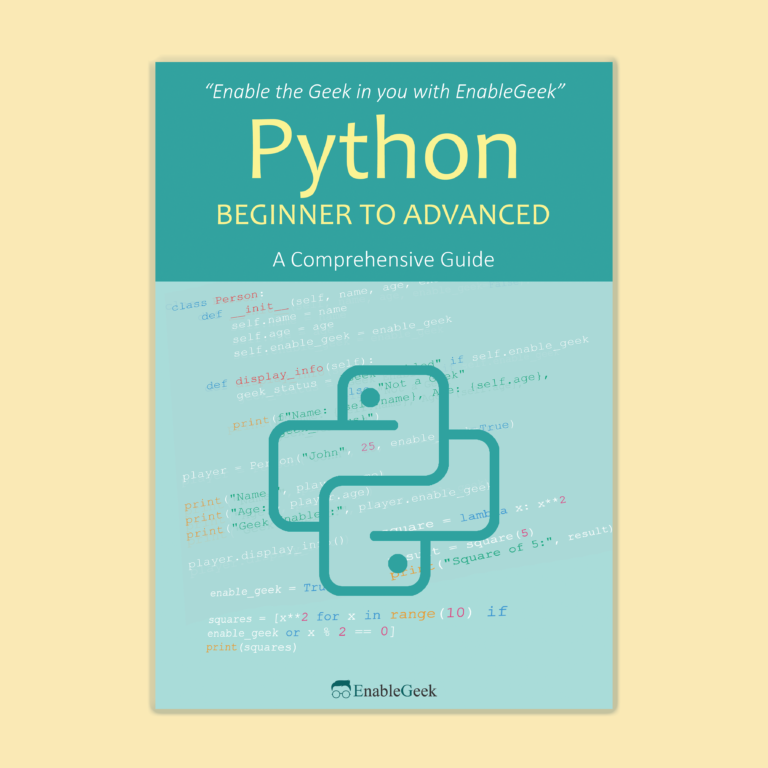
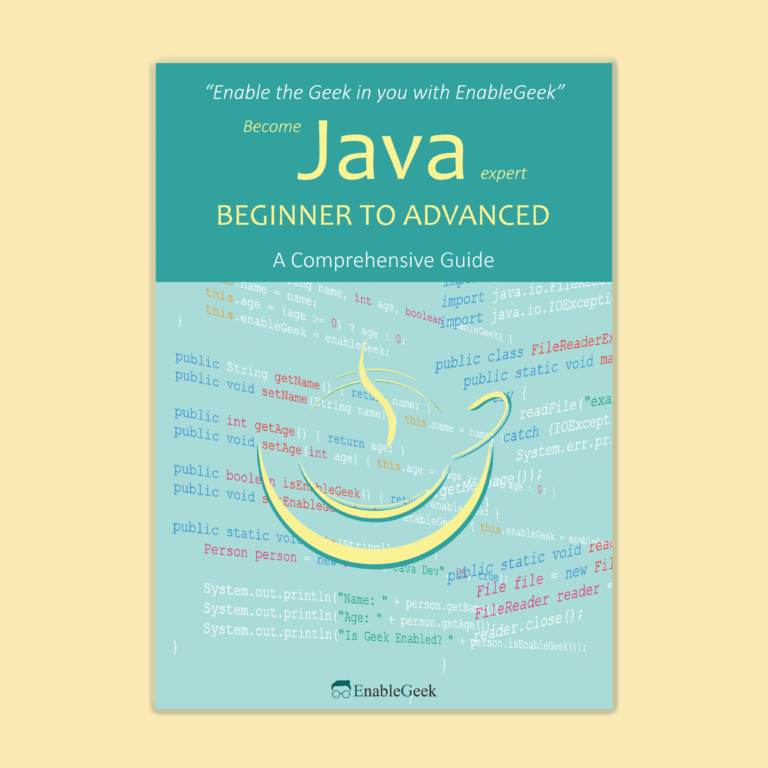
Advanced topics are covered in this ebook with many examples.